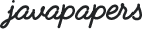
Following are the Java collections interview questions most frequently asked in interviews. I have attempted to keep the answers short and simple, as the objective is to help you prepare and revise topics for interviews quickly.
The best way to filter a Java collection is to use Java 8. Java streams and lambdas can be used to filter a collection as below,
List<Person> passedStudents = students.stream() .filter(p -> p.getMark() > 50).collect(Collectors.toList());
If for some reason you are not in a position to use Java 8 or the interviewer insists on pre Java 8 solution, following is the best way to filter a Java collection.
Use a Comparator to sort a Java Collection.
List<Animal> animals = new ArrayList<Animal>(); Comparator<Animal> comparator = new Comparator<Animal>() { public int compare(Animal c1, Animal c2) { //sort logic here return c2.getHeight() - c1.getHeight(); } }; Collections.sort(animals, comparator);
If Animal implements Comparable, then following is just enough.
Collections.sort(animals);
Instantiate Set using the HashSet.
Set<Animal> animalSet = new HashSet<Animal>(animalList);
Java’s LinkedList implementation is a doubly linked list. ArrayList is a dynamically resizing array implementation. So to compare between LinkedList and ArrayList is almost similar to comparing a doubly linked list and a dynamically resizing array.
LinkedList is convenient for back and forth traversal sequentially, but random access to an element is proportionally costlier to the size of the LinkedList. At the same time, ArrayList is best suited for random access using a position.
LinkedList is best for inserting and deleting an element at any place of the LinkedList. An ArrayList is not suited for inserting or deleting elements in the mid of the ArrayList. Since everytime a new element is inserted, all the elements should be shifted down and dynamic resizing should be done.
With respect to memory usage of LinkedList and ArrayList, LinkedList collection uses more memory as it needs to keep pointers to the adjacent elements. This overhead is not present for the ArrayList, just the memory required for the data is sufficient. Consider these factors and decide between LinkedList or ArrayList depending on the use case.
equals() method is used to determine the equality of two Java objects. When we have a custom class we need to override the equals() method and provide an implementation so that it can be used to find the equality between two instance of it. By Java specification there is a contract between equals() and hashCode(). It says,
"if two objects are equal, that is obj1.equals(obj2) is true then, obj1.hashCode() and obj2.hashCode() must return same integer"
Whenever we choose to override equals(), then we must override the hashCode() method. hashCode() is used to calculate the position bucket and keys.
Java PriorityQueue is a data structure that is part of Java collections framework. It is an implementation of a Queue wherein the order of elements will be decided based on priority of each elements. A comparator can be provided in the constructor when a PriorityQueue is instantiated. That comparator will decide the sort order of elements in the PriorityQueue collection instance.
We have beaten this enough in a old article difference between Vector and ArrayList in Java.
Concurrent Collections were introduced in Java 5 along with annotations and generics. These classes are in java.util.concurrent package and they help solve common concurrency problems. They are efficient and helps us to reduce common boilerplate concurrency code. Important concurrent collection classes are BlockingQueue, ConcurrentMap, ConcurrentNavigableMap and ExecutorService.
A class can implement the Comparable interface to define the natural ordering of the objects. If you take a list of Strings, generally it is ordered by alphabetical comparisons. So when a String class is created, it can be made to implement Comparable interface and override the compareTo method to provide the comparison definition. We can use them as,
str1.compareTo(str2);
Now, what will you do if you want to compare two strings based on it length. We go for the Comparator. We create a class and let it implement the Comparator interface and override compare method. We can use them as,
Collections.sort(listOfStrings, comparatorObj);
The natural ordering is up to the person designing the classes. Comparator can be used in that scenario also and it can be used when we need multiple sorting options. Imagine a situation where a class is already available and we cannot modify it. In that case also, Comparator is the choice.
Comments are closed for "Java Collections Interview Questions – Part I".
Sorting collections using java 8 is really a cool future. Thanks Joe for your commitment and helping people to understand the concepts better.
This site is a really good for java people. It will keep your knowledge refresh
Yes..really good.
Its nice .Can you Please add more like HashMap Collision
Sir , I am a 2 year experienced software professional . it would be more helpful if you add more articles like this in other technologies in java like springs , hibernate etc
Sundeep,
I am starting Hibernate tutorials in and out. You will get the first tutorial in Hiberate series within couple of weeks. Keep watching!
Archi,
Lot more coming on java interview questions. If you notice in the sidebar menu, I have added a category for interview questions.
With respect to Collections interview questions, this is only Part I. Many more parts to follow very soon. Keep watching.
Nice one. I’m preparing for my interview and these questions are very helpful. Thanks Joe.
Ppl like you make our lives easy at work ! Great work …
this is a great site to get started with Java..Thanks Joe.
Very nice collection of interview questions about Java Collections. Thanks Joe for your great contributions. From beginners to expert level, all your articles help us to understand things in a better way. Keep going!
Hi Joe,
It would be very nice if you add examples wherever possible which will give more clarity than searching for examples again somewhere else.
~Satheesh.
Great work … Sir please add java interview programs also it’ll more help ful us..
Superb Work..!! Happy Learning..
Excellent and Concise.. Thanks Joe :)
[…] to help prepare and revise for an interview in quick time. I recommend you to go through the Java collections interview questions part I before reading this […]
Hi Joe
You said the following
“If for some reason you are not in a position to use Java 8 or the interviewer insists on pre Java 8 solution, following is the best way to filter a Java collection.”
But i do not find any example to filter using pre java 8 .
Did i missing anything
Hi Joe,
Thanks for providing a valuable java information as versions and features for all java guys. Please provide me the Hibernate features of latest version.
Thanks in advance
Good one
Wonderful