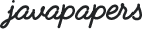
Array is used to store same ‘type’ of data that can be logically grouped together. Array is a fundamental construct in any programming languages. This Java tutorial is planned to provide comprehensive information about Java arrays.
Array is one among the many beautiful things in a programming language. Easy to iterate, easy to store and retrieve using their index. In Java, a beginner starts with public static void main(String args[])
. The argument for the main method is an array. We encounter arrays early on.
In java arrays are objects. All methods of an Object can be invoked on an array. Arrays are stored in heap memory.
Though we view the array as cells of values, internally they are cells of variables. A java array is a group of variables referenced by a common name. Those variables are just references to a address and will not have a name. These are called the ‘components’ of a java array. All the components must be of same type and that is called as ‘component type’ of that java array.
int []marks;
or
int marks[];
marks = new int[5];
5 inside the square bracket says that you are going to store five values and is the size of the array ‘n’. When you refer the array values, the index starts from 0 ‘zero’ to ‘n-1’. An array index is always a whole number and it can be a int, short, byte, or char.
Once an array is instantiated, it size cannot be changed. Its size can be accessed by using the length field like .length Its a java final instance field.
All java arrays implements Cloneable and Serializable.
int marks[] = {98, 95, 91, 93, 97};
One popular exception for java beginners is ArrayIndexOutOfBoundsException. You get ArrayIndexOutOfBoundsException when you access an array with an illegal index, that is with a negative number or with a number greater than or equal to its size. This stands second next to NullPointerException in java for popularity.
After you instantiate an array, default values are automatically assigned to it in the following manner.
Notice in the above list, the primitives and reference types are treated differently. One popular cause of NullPointerException is accessing a null from a java array.
public class IterateJavaArray { public static void main(String args[]) { int marks[] = {98, 95, 91, 93, 97}; //java array iteration using enhanced for loop for (int value : marks){ System.out.println(value); } } }
In language C, array of characters is a String but this is not the case in java arrays. But the same behaviour is implemented as a StringBuffer wherein the contents are mutable.
ArrayStoreException – When you try to store a non-compatible value in a java array you get a ArrayStoreException.
When a component type itself is a array type, then it is a multidimensional array. Though you can have multiple dimension nested to n level, the final dimension should be a basic type of primitive or an Object.
int[] marks, fruits, matrix[];
In the above code, matrix is a multidimensional array. You have to be careful in this type of java array declaration.
Internally multidimensional java arrays are treated as arrays of arrays. Rows and columns in multidimensional java array are completely logical and depends on the way you interpret it.
Note: A clone of a java multidimensional array will result in a shallow copy.
Following example source code illustrates on how to assign values and iterate a multidimensional java array.
public class IterateMultiDimensionalJavaArray { public static void main(String args[]) { int sudoku[][] = { { 2, 1, 3 }, { 1, 3, 2 }, { 3, 2, 1 } }; for (int row = 0; row < sudoku.length; row++) { for (int col = 0; col < sudoku[row].length; col++) { int value = sudoku[row][col]; System.out.print(value); } System.out.println(); } } }
java api Arrays contains static methods for sorting. It is a best practice to use them always to sort an array.
import java.util.Arrays; public class ArraySort { public static void main(String args[]) { int marks[] = { 98, 95, 91, 93, 97 }; System.out.println("Before sorting: " + Arrays.toString(marks)); Arrays.sort(marks); System.out.println("After sorting: " + Arrays.toString(marks)); } } //Before sorting: [98, 95, 91, 93, 97] //After sorting: [91, 93, 95, 97, 98]
You can use the following options to copy a java array:
Comments are closed for "Java Array".
Good summary, it’s essential to have good knowledge about array in java. Base for many programming tasks.
Good analysis and explanation for Java arrays.
Great tutorial for java array. It’s really very nice and easy to understand compared to any other Java tutorials.
Simple and Good explanation about Java array. Thanks
nice blog Sir…it’s so easy to understand for d beginners like me…i liked ur ACCESS MODIFIERS article 2….it helps me a lot….
thnx….
That’s very well documented. java array can’t be explained in more detail
Great patience, I must say!!
precise n important on array in java… great article…
Thanks for this complete tutorial on java array. Easy to understand than any Java book or blogs. Good analysis and explanation.
Thanks for these examples on array in java! Showing different ways to create string arrays is very helpful, as is the Java 5 for loop syntax example.
thanks, i too struggle with the java array syntax. these examples are very helpful.
Good explanation and thanks for this tutorial.
its good can be easily understood. I thought arrays are nothing and need not spend much time on it. after reading this tutorial I found more information on arrays. keep it up.
Nice Java blog….its very useful and easy to understand…… thankz
first, I appreciate your attitude behind writing this arrays article – sharing your knowledge with others. though it sounds like English is not your first language, I must say that you have tried your best to make it simple. no wonder it had a million hit.
Good explanation, but I didn’t not quite understand the physical view array part.
Overall very well written.
Joseph you rock!
best article for java array.
Keep it up friend.
why java index starts from 0 in java array??
tell us what is the fact.
Nice Java blog. Well presented tutorials. Looking forward for more java concepts.
Really nice tutorial on java array
Thanks for the information provided..its gud and easily understandable…but i got one doubt why the size of the array can’t be changed..??? any reason? or any rule?you mentioned that size of an array can’t be changed but why? please tell the answer…..
Superb Explanation. I feel its very useful and thankful. Java arrays are very basic concept. It cannot be explained simpler than this.
Really nice tutorial for java array! thanks.
So cool article dost. I love Java. I also used to write about java technologies. Just now i wrote some articles on java concepts and java programming test. Very soon I will publish them and will need your support.
very nice tutorial on array in java
it is very helpfull to other people
Simple and good. The best tutorial for arrays.
Very nice Java blog, I have bookmarked it.
Really Nice! Good effort for preparing this concise text on arrays. Thumbs up..
Nice.. tutorial for java array.. really helped lot… for the beginners..
All your Java tutorials are easy to understand, thanks.
marvelous, thanks for your effort in publishing these tutorials.
A wonderful effort to solve our problems thanks for your guidance sir. an easy approach to understand concepts of arrays,thanks.
Guys, thank you for all the appreciations.
Really nice tutorial on java array
thanks for writing this article..
arrays are very useful in daily programming :)
this is the nice tutorial for java beginners.
Really valuable.
this is very good and easily understandable definition of array and example is nice, thanks a lot.
Hi Joe,
arrays are objects in java.To create an object we need the corresponding Java class. so
Who creates array object, is it JVM ?
if JVM creates then which class it uses ?
who is the immediate super class of array object ?
is it implementing any interface ?
please explain it.
@Jagdish,
1. Yes, it is the JVM who creates array objects.
2. JVM uses java.lang.Object class to create array objects.
3. Object class from java.lang package is the immediate super class of any array objects.
4. No it does not implement any interface.
Also be informed that irrespective of the array size and dimensions only one array object is created on behalf of array.
i.e int[] arr = {1,2,3,4,5};
Above code creates only on object named arr in the heap memory.
String strArr = {“ONE”,”TWO”};
Three objects get created in above code.
1. strArry 2. “ONE” 3. “TWO”
Hope this solves your doubts.
Thank you for the good explanation.
in a Java program using Array I got exception as ArrayIndexOutOfBoundsException
how to debug this?
As you said, In Java arrays are objects..
For which class the array object is created?
Kindly answer to this question.
Now I have understood the Java arrays completely. Excellent sir, your Java tutorials are simply superb. Thanks.
[…] System – is a final class and cannot be instantiated. Therefore all its memebers (fields and methods) will be static and we understand that it is an utility class. As per javadoc, “…Among the facilities provided by the System class are standard input, standard output, and error output streams; access to externally defined properties and environment variables; a means of loading files and libraries; and a utility method for quickly copying a portion of an array…” […]
Thanks for giving the better explanation. In no other Java tutorials in the net this kind of simple tutorial is available. Thanks for using simple English.
Hi Joe, I have a question, can we create a dynamic array?, I was asked this question in an interview? I stood in the point that we cant create but we can use ArrayList instead, but the interviewer said it is possible, do you have answer for this?
Why we use (.length) in the code
A nice concise tutorial about arrays in java, thanks.
hi Baskaran,definitely we can create dynamic array in Java by using arraylist,it can store objects of any number and any type.
great . . i found it very useful and easy to understand…keep going :) god bless you
what is the default size of array in java
to creat an object..we write
classname variablename=new classname();
where , classname is data type
varname is object ref holder
new is keyword to allocate memory & return address
classname() is constructor to make an object & to initialze instance variable
but in Array creation we write
datatype []var_nam=new datatype[];
but my que is in creation why there is no constructor & how without it array object is created ?
please reply me sir.
Hey sir, u have helped me a lot in understanding Java arrays. Thanks a lot. Please continue writing more fundamental Java tutorials.
In your next tutorial, tell us more on Java error handling.
its really very easy to understand and thank you sir.
Why arrays start from 0 instead of 1?
This is useful for attending Java interviews, thanks.
Hi Joe,
Could you please provide the internal implementation of ArrayList. As we know, ArrayList internally uses Array. Array stores the addresses consecutively in physical memory but in case of ArrayList, we can add any number objects at any time. How is it going to work internally in terms of storage?
it is very useful for all students
thanks sir. now I have understood arrays concept in Java fully. thank u very much. this is very helpful :-)
Good for Java beginners. Easy to understand tutorial, thanks.
tell me about new technique in array in Java which is easy to learn or implement.
Thank you sir. javapapers is very good for fresher. All the Java tutorials are easy to understand.
Please tell me how to implement values by user through at run time in Java using matrix array?
I am a Java beginner and have a doubt. what is the need of Arrays concept in java?
Java collections looks sufficient for every scenario where I need to use Arrays.
very nice. All your Java tutorials are simply great, thanks.
what is the default size of array in java
Very nice with explanation. Please write lot more such Java tutorials.
It’s fabulos. Helped me a lot. Its been long I read about Java array.
Now recollecting all those Java fundamental concepts is making me feel fresh, thanks.
Thanks a lot :-)
The example code for array using Java sudoku Program is lovely :-)
Very lucidly explained. can’t make a array tutorial better than this. many sites just state the fact rather providing a complete simple code. Thanks to you for your sincere efforts, vision and knowing what starters like us are really looking for. Please continue writing more such Java tutorials!
Explanation was Good, but i have doubt on array iteration.
exp :
int Arr [] = {49, 50, 60, 45, 75};
for(int i=0; i<5; i++)
{
System.out.println(Arr[i]);
}
in the above exp how it will find the Arr[i], directly.
Declaration is ok and Initialization also ok but in print method how it is going to find [i] here.
can you please explain for me
Its very good blog ….helped me a lot as a fresher in java
Hi Sir,
I Want solution for the below question. Could you please send me solution.
Question: We are given an array of 2n integers wherein each pair in this array of integers represents the year of birth and the year of death of a dinosaurs respectively. The range of valid years we want to consider is [-100000 to 2005]. For example, if the input was:
-80000 -79950 20 70 22 60 58 65 1950 2004
it would mean that the first dinosaur had a birth year of –80000 and an year of death of –79950 respectively. Similarly the second dinosaur lived from 20 to 70 and so on and so forth.
We would like to know the largest number of dinosaurs that were ever alive at one time. Write a method to compute this, given the above array of 2n integers.
using method
public int findMaxdinosaur (int[] years);
nice explanation.
the array index value is given by three values. so how many index value are possible for java array?
[…] data area is used to store objects of classes and arrays. Heap memory is common and shared across multiple threads. This is where the garbage collector […]
i like that you mentioned default values in array GOOD
Why array index starts from zero in Java.
Very well explained. I will say even for few things in arrays i never pay attention.. but everything matters in programming. Thank you for this.
[…] is based on a bounded Java array. Bounded means it will have a fixed size, once created it cannot be resized. This fixed size […]