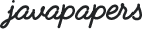
A java exception can be thrown only in the following three scenarios:
(1) An abnormal execution condition was synchronously detected by the Java virtual machine.
– When evaluation of an expression violates the normal semantics (Example: an integer divide by zero)
– An error occurs in loading or linking part of the program
– When limitation on a resource is exceeded (Example: using too much memory)
(2) A throw statement was executed.
(3) An asynchronous exception occurred.
– The stop method (deprecated) of class Thread was invoked
– An internal error has occurred in the java virtual machine
Comments are closed for "What are the causes of exceptions in java?".
I was reading your articles last day[actually first time:-(]..
I have a query while working with StringTokenizer. Please see the code below and please let me know what is wrong in that and why it is throwing PatternSysntaxException? Is it wrong way to use?
class TestHashMap{
public TestHashMap(){
HashMap hMap=new HashMap();
hMap.put(“Name”, “Mahesh”);
hMap.put(“Age”, 26);
hMap.put(“Designation”, “Software Engineer”);
hMap.put(“Company”, “Logica”);
System.out.println(hMap);
String hMapString = hMap.toString();
hMapString = hMapString.replaceAll(“{“, “”);
StringTokenizer hMapStringTokens=new StringTokenizer(hMapString, “,”);
while(hMapStringTokens.hasMoreTokens()){
StringTokenizer keyValueTokens=new StringTokenizer(hMapStringTokens.nextToken(),”=”);
while(keyValueTokens.hasMoreTokens()){
System.out.println(“Token->”+keyValueTokens.nextToken());
}
}
}
}
Hi Mahesh,
try this
import java.util.HashMap;
import java.util.StringTokenizer;
class Test {
public Test() {
HashMap hMap = new HashMap();
hMap.put(“Name”, “Mahesh”);
hMap.put(“Age”, 26);
hMap.put(“Designation”, “Software Engineer”);
hMap.put(“Company”, “Logica”);
System.out.println(hMap);
String hMapString = hMap.toString();
hMapString = hMapString.replace(“{“,””);
hMapString = hMapString.replace(“}”,””);
StringTokenizer hMapStringTokens = new StringTokenizer(hMapString, “,”);
while (hMapStringTokens.hasMoreTokens()) {
StringTokenizer keyValueTokens = new StringTokenizer(
hMapStringTokens.nextToken(), “=”);
while (keyValueTokens.hasMoreTokens()) {
System.out.println(“Token->” + keyValueTokens.nextToken());
}
}
}
public static void main(String[] args){
new Test();
}
}
Output:
{Name=Mahesh, Age=26, Company=Logica, Designation=Software Engineer}
Token->Name
Token->Mahesh
Token-> Age
Token->26
Token-> Company
Token->Logica
Token-> Designation
Token->Software Engineer
In general, unchecked exceptions represent defects in the program (bugs), which are normally Runtime exceptions.
Furthermore, checked exceptions represent invalid conditions in areas outside the immediate control of the program.
hi i need prgrm for there is one arraylist having some field like (id, name,age) another arraylist having field (id).In the list have common values ,display that common field with all details. condition is 1.only (i+j) iteration will perform 2.don’t use contains… i need program for this one…
hi,
I want exactly how vector is thread safe and why we array list is not thread safe………….
I want explanation through example….can u plz write a program as a example
Please describe about the StringTokenizer class
[…] popular exception for java beginners is ArrayIndexOutOfBoundsException. You get ArrayIndexOutOfBoundsException when […]