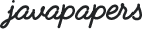
This Java tutorial is to discuss about ArrayBlockingQueue which is an implementation for a Java BlockingQueue Collection. We will see a producer consumer based example using ArrayBlockingQueue to understand the BlockingQueue interface.
ArrayBlockingQueue is based on a bounded Java array. Bounded means it will have a fixed size, once created it cannot be resized. This fixed size collection can be understood using this Java List size puzzle.
ArrayBlockingQueue uses the “Blocking” type method from the four available types described in the Java BlockingQueue tutorial. Attempt to put into the empty ArrayBlockingQueue and take will be blocking the thread.
This is a producer which produces three string values and put() to the BlockingQueue. These three elements are produced between varied intervals.
package com.javapapers.java.collections; import java.util.concurrent.BlockingQueue; public class BlockingQueueProducer implements Runnable { protected BlockingQueue<String> blockingQueue; public BlockingQueueProducer(BlockingQueue<String> queue) { this.blockingQueue = queue; } public void run() { try { Thread.sleep(500); blockingQueue.put("Lion"); Thread.sleep(1000); blockingQueue.put("Crocodile"); Thread.sleep(2000); blockingQueue.put("Jaguar"); } catch (InterruptedException e) { e.printStackTrace(); } } }
This is a consumer which consumes string values by take() from the BlockingQueue. These elements are consumed based on their availability from the producer.
package com.javapapers.java.collections; import java.util.concurrent.BlockingQueue; public class BlockingQueueConsumer implements Runnable { protected BlockingQueue<String> blockingQueue; public BlockingQueueConsumer(BlockingQueue<String> queue) { this.blockingQueue = queue; } public void run() { try { System.out.println(blockingQueue.take()); System.out.println(blockingQueue.take()); System.out.println(blockingQueue.take()); } catch (InterruptedException e) { e.printStackTrace(); } } }
package com.javapapers.java.collections; import java.util.concurrent.ArrayBlockingQueue; import java.util.concurrent.BlockingQueue; public class BlockingQueueExample { public static void main(String[] args) throws Exception { BlockingQueue<String> blockingQueue = new ArrayBlockingQueue<String>(1024); BlockingQueueProducer queueProducer = new BlockingQueueProducer(blockingQueue); BlockingQueueConsumer queueConsumer = new BlockingQueueConsumer(blockingQueue); new Thread(queueProducer).start(); new Thread(queueConsumer).start(); } }
Lion Crocodile Jaguar
Comments are closed for "Java ArrayBlockingQueue".
[…] ArrayBlockingQueue […]
[…] DelayQueue is a collection and an implementation of BlockingQueue. Unlike ArrayBlockingQueue, DelayQueue is an unbounded collection. The BlockingQueue methods are implemented in such a way […]
[…] ArrayBlockingQueue – a blocking queue class based on bounded Java Array. Once instantiated, cannot be resized. […]