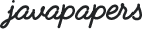
String is one of the widely used java classes. It is special in java as it has some special characteristics than a usual java class. Lets explore java String in this article and this page aims to serve you as a single point reference for all your queries on Java String.
Though you might be knowing most of the things, this will help you to recall them and I am sure you will find one or two new things. I recommend you to take your time and read through this completely as java String is a basic building block of your java programs.
Java String is a immutable object. For an immutable object you cannot modify any of its attribute’s values. Once you have created a java String object it cannot be modified to some other object or a different String. References to a java String instance is mutable. There are multiple ways to make an object immutable. Simple and straight forward way is to make all the attributes of that class as final. Java String has all attributes marked as final except hash field.
Java String is final. I am not able to nail down the exact reason behind it. But my guess is, implementors of String didn’t wany anybody else to mess with String type and they wanted de-facto definition for all the behaviours of String.
We all know java String is immutable but do we know why java String is immutable? Main reason behind it is for better performance. Creating a copy of existing java String is easier as there is no need to create a new instance but can be easily created by pointing to already existing String. This saves valuable primary memory. Using String as a key for Hashtable guarantees that there will be no need for re hashing because of object change. Using java String in multithreaded environment is safe by itself and we need not take any precautionary measures.
In continuation with above discussion of immutability of java String we shall see how that property is used for instantiating a Sting instance. JVM maintains a memory pool for String. When you create a String, first this memory pool is scanned. If the instance already exists then this new instance is mapped to the already existing instance. If not, a new java String instance is created in the memory pool.
This approach of creating a java String instance is in sync with the immutable property. When you use ‘new’ to instantiate a String, you will force JVM to store this new instance is fresh memory location thus bypassing the memory map scan. Inside a String class what you have got is a char array which holds the characters in the String you create.
String str1 = "javapapers"; String str2 = new String("Apple"); String str3 = new String(char []); String str4 = new String(byte []); String str5 = new String(StringBuffer); String str6 = new String(StringBuilder);
We have an empty constructor for String. It is odd, java String is immutable and you have an empty constructur which does nothing but create a empty String. I don’t see any use for this constructor, because after you create a String you cannot modify it.
Do not use == operator to compare java String. It compares only the object references and not its contents. If you say, “if references are same, then the value must be same” – this doesn’t cover, “even if references are not same, the content can be same”. Therefore == operator doeenot 100% guarantee the equality of java String. Consider,
String stringArray1 = new String(“apple”, “orange”);
“apple” == stringArray1[0]; gives FALSE.
“apple”.equals(stringArray1[0]); gives TRUE.
equals() method is part of Object class. Java String class overrides it and provides implementation for equality comparison. String’s equals() implemetation uses three step process to compare two java String:
Shall we always use equals() method for equality comparison in any type of objects? No. You need to check the implementation in its respective class. StringBuffer and StringBuilder do not have an implementation for equals() method.
Use equalsIgnoreCase(String) to compare String irrespective of case (Case insensitivity).
“javaString”.equalsIgnoreCase(“JAVASTRING”); returns TRUE.
First what is lexicographic comparison? Consider, ‘A’ for ‘Apple’ and ‘B’ for ‘Ball’. Term ‘Apple’ appears first in dictionary and later follow ‘Ball’. Java String has got an excellent method to do this sort of comparison for two String and identify which comes first and the other follows.
“Apple”.compareTo(“Ball”);
In the above, “Apple” calls compareTo(String) and “Apple” comes first lexicograpically before “Ball”, therefore this returns value < 0. If calling String and argument String are same then returned value is 0. That is, “Apple”.compareTo(“Apple”); Else, you get > 0.
Java conversion is a huge topic by itself. I will take only String as scope for this section.
Example: 1+” Apple”; results in “1 Apple”.
So how does the above happen? When + operator is used with a java primitive, following happens:
One of the most frequent questions on String conversion is
Best way to convert String to Date is as follows:
String dateInString = "November 21, 2011"; SimpleDateFormat dateFormat = new SimpleDateFormat("MMMM d, yyyy", Locale.ENGLISH); Date formattedDate = dateFormat.parse(dateInString);
How to transform a String to upper / lower case. Java String has got nice utility methods.
toLowerCase(Locale locale)
toUpperCase(Locale locale)
and String can be trimmed using trim()
Hope you remember about the memory pool of java String that we discussed at the start of this article. We have a method named intern() in java String. When you invoke this method on a String,
+ is a special operator when it comes to java String. It helps to concatenate two String. I have already written a post exclusively for String concatenation. Please refer that for more on java String concatenation.
Comments are closed for "Java String".
Nice Tutorial…
it is very nice notes about String..
Thanks for sharing it with us.
and hoping for the same in the future..
yours
amit
thank you so much
very straight forward article.
You are back after a long time with a good article!
this is nice tutorials.
Yes Praveen.
I plan to write as often as possible, hope I keep up the promise.
Thank you so much for this information!!
it is very nice tutorial to every learner in java strings
really nice article
Very nice tutorial..
nice blogs, i have gone through all of your topics , much helped me for interviews.
1. “Java String is final.”
Immutability necessitates that a class be final or at least there should be no way to extend it(by providing private constructor & factory methods)
2. “Shall we always use equals() method for equality comparison in any type of objects?”
Why not? At worst if a class does not override the equals method, the equals method from Object would be used (which just compares if the two references are equals).
The reason a class may not have overridden the equals method is if the equals method of the Object class is sufficient for it.
Good practice would dictate that equals method be overridden for most class and “equals” method be called when comparing two objects.
3. “It is odd, java String is immutable and you have an empty constructur which does nothing but create a empty String. I don’t see any use for this constructor, because after you create a String you cannot modify it.”
I’m sure that empty constructor is used a lot.
After all how many time have we done,
String default = “”;
Empty String would be created using the default constructor … just because we have a short cut to create strings doesn’t change the fact that Strings are real objects and the JVM needs to call a proper constructor to create it!
Great article. It did cover a lot of good ground!
Hi Sam,
Regarding using empty constructor,
String default=””;
yes I agree we use the above a lot. But my question is, though we are creating an empty String we are not again using the same object. Since java String is immutable, already instantiated empty String is not modified to create the new String. Thats why I told it as odd..
People tend to think of the following as instantiating and assigning. But it is not the case w.r.t String.
String fruit =””;
fruit = “apple”;
Thanks for the comment.
implementors of String didn’t wany anybody else to mess with String type and they wanted de-facto definition for all the behaviours of String.
in this there is spelling amistake instead of want u wrote wany
Hi Joe,
I read your articles and finding it very useful to understand the actual concept.
Could you please explain about
hashCode() method and relation between equals() and hashCode()
Thanks,
Darsh
hello joe,
thanks for this nice blog but i have to ask
String str1 = “javapapers”;
String str2 = new String(“Apple”);
what is the difference between them and when two use String str1 = “javapapers”;
and when using new operator..
really very nice blog.. keep it up.
Hi joe,
Now am the follower of your blog. I need a clear explain of hashCode(). Can you help me about that. I have read some article related to hashCode(). But still am not clear. Can you please explain with an example and output.
Thanks,
Manoj
Hi joe,
I must say that your explanation and examples are very good and easy to understand.
when we create a string object using String str1=”javapapers” jvm will create a string object in the string constant pool.when we use statement String str2=new String(“Apple”) then jvm will create a string object in the heap area.
Thanks for educating all.
Hi,
I likes ur blog
I have a question in my mind.
String s1=”HELLO”;
String s2=”HELLO”+s1+new String(“hai”)+”RAAm”;
String s3= s1+s2;
Now my question is how many objects are creating above in STRING1,STRING2,STRING3
AND EXPLAIN TO ME…..
Such a very nice topic joe. Thanks for posting…..
But i have a question, i didn’t get
References to a java String instance is mutable.
String s1=”Hi”;
In the above statement s1 is a reference to the string object. You have not actually created the object. Already existing “Hi” string from the string pool is assigned to the reference obj rather than creating a new string object. s1 which is a reference is mutable.
If you say
String s2 = new String(“Hi”);
s2 is a new string object which is immutable.
correct me if I am wrong.
thank u very much sir..
you are always great sir…….
Thankx for such a nice job
Very good initiative never seen a good heart to teach and share it like this
[…] String class will be the undisputed champion on any day by popularity and none will deny that. This is a […]
Sir,
In both case gives the result as equal.
System.out.println(“— part1 —“);
String[] strArr = {“as”, “AS”};
if(“as” == strArr[0])
System.out.println(“equal”);
else
System.out.println(“not equal”);
System.out.println(“— part2 —“);
String[] stringArray1 = new String[]{“apple”, “orange”};
if(“apple” == stringArray1[0])
System.out.println(“equal”);
else
System.out.println(“not equal”);
class StringEx
{public static void main(String args[])
{String s1=”hello”;
string s2=”friend”;
s1=s1+s2;
System.out.println(s1);
}
}
sir, the output for the program is hellofriend
then how can we say that string is immutable bcoz as here the string is being retrived by another would you plz explain it as early as possible..
sir..
class StringEx
{public static void main(String args[])
{String s1=”hello”;
string s2=”friend”;
s1=s1+s2;
System.out.println(s1);
}
}
sir, the output for the program is hellofriend
then how can we say that string is immutable bcoz as here the string is being retrived by another would you plz explain it as early as possible..
[…] interviewers. Sometimes we even get bored of this play with String equals. I have written a detailed tutorial on java string and its immutable property, comparing strings and conversion. This is one area where people keep on […]
Hi Joe,
Your blog is very simple to understand and so its awesome.
I have a question here, below string from your doesn’t seem to be a valid
String stringArray1 = new String(“apple”, “orange”);
however
String[] stringArray1 = {“apple”, “orange”};
is fine.
did you mean the below one ?
String s=”abc”;
s=”bcd”;
it is possible or not,if possible mention reason if possible
Hi joe
can you explain about why stringBuffer and stringBuilder doent override equals and hashcode methods
Not possible.
Hi, Here you are using String literals which are referencing to String literal pool. And contents of s1 are different so they are not referencing the same objects.
Read about string pool if you have any doubts regarding string literals.
hello joi,
Your blog is realy very good. Your explanation is very simple and clear. So if you dont mind plz you also post about operator overloading in java and encapsulation in java. i want to know your view for this two topics. thanks.
String s1 = new String (“hare krishna hare krishna”);
String s2 = new String (“hare krishna hare krishna”);
if (s1==s2) { //Only compare the Object not the Value
System.out.println(“true”);
} else {
System.out.println(“false”);
}
if (s1.equals(s2)) { //Only compare the Value
System.out.println(“true”);
} else {
System.out.println(“false”);
}
//output
false
true
really very nice post…..thanks
thnx this blog is really very useful .but I have one quest i.e. if == is compares only the object references and not its contents. then when and where should use this …..waiting for your replay ……..
Hi joe plz give some hint about that creating a new object everytime whe we create an object.
i have a question when we write a same method in both abstract class and interface and a class imeplents and extends both then which method will be invoked?
ex:
interface a
{
public void show();
}
abstract class b
{
abstract public void show();
}
public class c extends b implements a
{
public void show()
{
System.out.println(“hi”);
}
public static void main(String[] args)
{
c t = new c();
t.show();
}
}
i dont like ur example no one is coreect and not understandable
so tuff not correct
its very nice.
thank you..
Hi joe,
what is difference between two Intialization given below?
String s=”Anwar”;
String s1=new String(“Anwar”);
Hi Joe,
Your explanations and the examples you take are very helpful.
String stringArray1 = new String(“apple”, “orange”);
This particular line in the above post is wrong and should be replaced by
String[] stringArray1 = new String[]{“Apple”,”Orange”};
Please reply if my observation is wrong.
Thanks for your knowledge sharing.
please create a index of all the topics in this tutorial to make navigation easy
btw great tutorial
Is the object hashcode and object reference same?
str1 is a pointing javapapers kk.first of all it checks in String Constant Pool memory.in that javapapers is not there so it create object for javapapers stored in const memory next time you writen str2=”javapapers” str2=str1;
bcoz same string why to create object so pointing to same ref it saves physicalmemory one onebject contains 8bits
Hi Laxmi,
String s1=”Hi”;
=> Here one object with value “Hi” is created and 1 reference s1 to refer that object is created.
=> So string object is immutable but you can use s1 to refer other object.
String s1 = new String(“Hi”);
=> Here two objects created and one reference variable to refer “Hi”
=> So this both object are immutable but you can change reference of s1 to refer other string object.
Hope you got your answer
[…] be you should also go through String equals also at this juncture as String equality is one of the celebrated topic in […]
hai frd….
String a=”hai”;
if we write like this the value of a is gng to store into the String concatenate pool and it may or may not store into the heap memory.
String a=new String(“hai”)’
it is directly stores into the heap memory.
that is the main difference in that
nice arrticle.thanks and keep it up
It is a very nice post.Thanks for posting this explanation.
I have tested its possible please check.
[…] Object has a method named equals. In String class the default behavior is overridden. The String equals behavior is modified to check if the character sequence is same as the compared one and returns […]
Hi joe,
if i create a string as
String s=new String(“Joe”);
is “Joe” will also be created in String Constant Pool along with object in Heap.
if so what is the use of intern() method?
Thanks
String s1 = “hare krishna hare krishna”;
String s2 = “hare krishna hare krishna”;
if (s1==s2) { //Only compare the Object not the Value
System.out.println(“true”);
} else {
System.out.println(“false”);
}
if (s1.equals(s2)) { //Only compare the Value
System.out.println(“true”);
} else {
System.out.println(“false”);
}
check it out
Hi Joe,
A very good article for every who intended to know more about java String,hope you post more about String classes like StringBuffer and StringBuilder
can you give some idea about compareToIgnoreCase
thanks
sorry this one for joe
Hi,
To answer this question,
For s1->1 obejct will be created,s2-> 2 objects created one is for HelloHellohaiRAAM and aonther is hai,For s3-> one more object created by concadinating both the strings.
I guess this is correct based on my understaing, correct me if it is worng
Hi Joe,
I really like your passion towards Java. Can u pls explain intern() in detail.
When we say
String a = “abc”;
First it will scan the String pool if found return the string else it will add that to String pool. Correct me if Im wrong.
I don’t understand what new thing the intern() method does here???
Please make me clear
String stringArray1 = new String(“apple”, “orange”);
“apple” == stringArray1[0]; gives FALSE
But the above statements is giving “TRUE” as output, please verify & explain it.Thanks