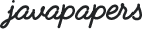
This tutorial will serve as a getting started guide for Maven. If you are not aware of what Maven is, then go through my previous tutorial, it has introduction to Maven, how to setup it and Ant Vs Maven, and its advantages.
This tutorial is for developers who want to know only the essentials of Maven, who just want to dive immediately and swim. This tutorial may not give you the complete picture about Maven, but this will be sufficient to start working with Maven and will serve as a first step towards your mastery in Maven.
Maven defines and follows conventions. Right from the project structure to building steps, Maven provides conventions to follow. If we follow those conventions, with minimal configuration we can easily get the build job done.
There are three built-in build life cycle ‘clean’, ‘default’ and ‘site’. A life cycle has multiple phases. For example, ‘default’ lifecycle has following phases (listed only the important phases),
So to go through the above phases, we just have to call one command:
mvn <phase> { Ex: mvn install }
For the above command, starting from the first phase, all the phases are executed sequentially till the ‘install’ phase.
Repository is where the build artifacts are stored. Build artifacts means, the dependent files (Ex: dependent jar files) and the build outcome (the package we build out of a project). Below shown picture is my local maven repository and its default path where it is stored.
There are two types of repositories, local and remote. Local maven repository is in the user’s system. It stores the copy of the dependent files that we use in our project as dependencies. Remote maven repository is setup by a third party to provide access and distribute dependent files. Ex: repo.maven.apache.org
This is roughly equivalent to the ANT build xml file. Maven pom.xml contains the configuration settings for a project build. Generally we define the project dependencies (Ex: dependent jar files for a project), maven plugins to execute and project description /version etc.
A pom will minimum have the following information,
Example of a simplest pom.xml,
<project> <modelVersion>4.0.0</modelVersion> <groupId>com.mycompany.app</groupId> <artifactId>my-app</artifactId> <version>1</version> </project>
There is an element available for declaring dependencies in project pom.xml This is used to define the dependencies that will be used by the project. Maven will look for these dependencies when executing in the local maven repository. If not found, then Maven will download those dependencies from the remote repository and store it in the local maven repository.
Example declaring junit and log4j as project dependencies,
<dependencies> <dependency> <groupId>junit</groupId> <artifactId>junit</artifactId> <version>3.8.1</version> <scope>test</scope> </dependency> <dependency> <groupId>log4j</groupId> <artifactId>log4j</artifactId> <version>1.2.12</version> <scope>compile</scope> </dependency> </dependencies>
scope – describes under which context this dependency will be used.
All the execution in Maven is done by plugins. A plugin is mapped to a phase and executed as part of it. A phase is mapped to multiple goals. Those goals are executed by a plugin. We can directly invoke a specific goal while Maven execution. A plugin configuration can be modified using the plugin declaration.
An example for Maven plugin is ‘compiler’, it compiles the java source code. This compiler plugin has two goals compiler:compile and compiler:testCompile.
Using the configuration element, we can supply arguments to the plugin.
<build> <finalName>springexcelexport</finalName> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-war-plugin</artifactId> <version>2.2</version> </plugin> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-surefire-plugin</artifactId> <version>2.16</version> <configuration> <skipTests>true</skipTests> </configuration> </plugin> </build>
Maven uses a convention for project folder structure. If we follow that, we need not describe in our configuration setting, what is located where. Maven knows from where to pick the source files, test cases etc. Following is a snap shot from a Maven project and it shows the project structure.
Let us create our first maven project. Hope you have setup Maven already. In Maven we have ‘Archetype’. It is nothing by a template for projects. Maven provides templates to start a project and using this we can quickly start a Maven project. Execute the following command in cmd prompt,
mvn archetype:generate -DgroupId=com.javapapers.sample -DartifactId=first-mavenapp -DarchetypeArtifactId=maven-archetype-quickstart -DinteractiveMode=false
This will create a sample Maven project skeleton using we can start building the application.We will get a a pom.xml and let us use that to build the newly created Maven project. Go inside the newly created Maven project root and execute the command (this is where the pom.xml is available),
mvn package
Now this will execute all the Maven phases till the ‘package’ phase. That is, Maven will compile, verify and build the jar file and put it in target folder under the project.
You can experiment with these Archetypes, maven-archetype-j2ee-simple and maven-archetype-webapp.
Comments are closed for "Maven in 10 Minutes".
Why do we need build tool ant or maven, The IDE itlself give compilation & execution phase. Could you explain please
How to call/load java methods/class from oracle databases?
Nice article.
Could you please cover the ‘parent’ tag in pom.xml and other important things also. This article is very basic.Also, please give details like what is group id, artifact id etc.
The IDE uses any of the build tool. E.g. if you want to use Mavan, you can also use it with IDE. Instead you use the commands in Maven, your IDE will use them. It will provide you a easier way to develop your app.
Hi,
Even my question is same. If i create a project in eclipse then eclipse itself can compile and even we can export as a jar too. So why and where we need a tool like maven or ant. Please explain this….
There are continuous integration tools like Jenkins that can allow you to build the project using maven remotely and automatically, regardless of your development IDE.
Whats is difference between goal and profile
Hi, thank you for the blog, very helpful. Would you please explain the tags in pom.xml, for example, how does maven read the ?
Hi Joe , I was wondering if there is a way to specify dependencies as URLs to the Resources…
What are you trying to achieve? In any case, if the dependency not found in the local repository maven will attempt to download it from central repository or any remote repository it is configured to use.
using loadjava executable file we can load java class in Dabtabase , you can it as calling a normal oracle functions. there are lots of examples on web
[…] Maven dependency for the ZXing QR Code library: […]
How to convert existing ant project to maven?
I really find your blogs interesting.
Thank you!!!:)
[…] For this example application using Spring framework and Java, lets use Maven for managing the dependencies. […]
Is there a way to convert the existing ANT projects to Maven and also as requested by others could you please explain the POM.xml file.
[…] This is a quick tip to help import a Maven project into Eclipse IDE. To learn Maven quickly check the Maven in 10 minutes tutorial. […]