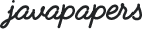
Sending email in Spring framework is simpler than sending email in Java using JavaMail API. If sending email in plain Java is eating a nutty cake, then sending email in Spring framework is eating a nutty ice cream. Let us use GMail SMTP server for sending example email.
So far we have seen how we can send email in Java using JavaMail API and then an email app in Android using JavaMail API and GMail SMTP. Now to complete this email series (at least for now), we shall do email with Spring and GMail.
We should know the basics of Spring framework and how to use Spring MVC. If you are not comfortable then, you should refer the older tutorials. If you combine the Spring MVC and JavaMail knowledge then this article is nothing new.
For this example application using Spring framework and Java, lets use Maven for managing the dependencies.
This is the key Spring Java class in sending email using Spring framework. We have autowired SpringMailMessage and JavaMailSenderImpl. These two are Spring’s classes and they are used for constructing the email message and to send email respectively. Here in this example we are using SpringMailMessage to have a email template and it is a transport helper object from Spring.
We use JavaMailSenderImpl and instantiate a mimemessage (Java’s class), use MimeMessageHelper to set the email parameters and send the email.
package com.javapapers.spring; import javax.mail.MessagingException; import javax.mail.internet.MimeMessage; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.mail.SimpleMailMessage; import org.springframework.mail.javamail.JavaMailSenderImpl; import org.springframework.mail.javamail.MimeMessageHelper; import org.springframework.stereotype.Service; @Service("springMailSender") public class SpringMailSender { @Autowired private SimpleMailMessage emailTemplate; @Autowired private JavaMailSenderImpl javaMailSender; public void setEmailTemplate(SimpleMailMessage emailTemplate) { this.emailTemplate = emailTemplate; } public void setJavaMailSender(JavaMailSenderImpl javaMailSender) { this.javaMailSender = javaMailSender; } public void sendMail(String dear, String content) { String fromEmail = emailTemplate.getFrom(); String[] toEmail = emailTemplate.getTo(); String emailSubject = emailTemplate.getSubject(); String emailBody = String .format(emailTemplate.getText(), dear, content); MimeMessage mimeMessage = javaMailSender.createMimeMessage(); try { MimeMessageHelper helper = new MimeMessageHelper(mimeMessage, true); helper.setFrom(fromEmail); helper.setTo(toEmail); helper.setSubject(emailSubject); helper.setText(emailBody); /* uncomment the following lines for attachment FileSystemResource file = new FileSystemResource("attachment.jpg"); helper.addAttachment(file.getFilename(), file); */ javaMailSender.send(mimeMessage); System.out.println("Mail sent successfully."); } catch (MessagingException e) { e.printStackTrace(); } } }
This XML file contains the Spring configuration and email template for sending email. We define a bean for SpringMailMessage and use it as an email template. For richer email template options we can use Velocity and I will cover that in a future article. GMail’s SMTP parameters for sending email are set as properties in the javaMailSender bean definition.
<beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:p="http://www.springframework.org/schema/p" xmlns:aop="http://www.springframework.org/schema/aop" xmlns:context="http://www.springframework.org/schema/context" xmlns:jee="http://www.springframework.org/schema/jee" xmlns:tx="http://www.springframework.org/schema/tx" xmlns:task="http://www.springframework.org/schema/task" xsi:schemaLocation="http://www.springframework.org/schema/aop http://www.springframework.org/schema/aop/spring-aop-3.2.xsd http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.2.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.2.xsd http://www.springframework.org/schema/jee http://www.springframework.org/schema/jee/spring-jee-3.2.xsd http://www.springframework.org/schema/tx http://www.springframework.org/schema/tx/spring-tx-3.2.xsd http://www.springframework.org/schema/task http://www.springframework.org/schema/task/spring-task-3.2.xsd"> <context:component-scan base-package="com.javapapers.spring" /> <bean id="javaMailSender" class="org.springframework.mail.javamail.JavaMailSenderImpl"> <property name="host" value="smtp.gmail.com" /> <property name="port" value="587" /> <property name="username" value="fromemail@gmail.com" /> <property name="password" value="frompassword" /> <property name="javaMailProperties"> <props> <prop key="mail.smtp.ssl.trust">smtp.gmail.com</prop> <prop key="mail.smtp.starttls.enable">true</prop> <prop key="mail.smtp.auth">true</prop> </props> </property> </bean> <bean id="emailTemplate" class="org.springframework.mail.SimpleMailMessage"> <property name="from" value="fromemail@gmail.com" /> <property name="to" value="joe@javapapers.com" /> <property name="subject" value="Send Email using Spring and Java" /> <property name="text"> <value> <![CDATA[ Hi %s, This is a test email sent from Spring framework using JavaMail. Regards, %s. ]]> </value> </property> </bean> </beans>
This Java class just serves as main class to start the program and send email parameters for the email template. We pass couple of parameters and that will be substituted in the email template as values for %s.
package com.javapapers.spring; import org.springframework.context.ConfigurableApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class SpringEmail { public static void main(String[] args) { ConfigurableApplicationContext applicationContext = new ClassPathXmlApplicationContext( "applicationContext.xml"); SpringMailSender springMail = (SpringMailSender) applicationContext .getBean("springMailSender"); springMail.sendMail("Joe", "Javapapers"); applicationContext.close(); } }
Maven pom xml which contains the application dependencies.
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>com.javapapers.spring</groupId> <artifactId>SpringEmailGmail</artifactId> <version>0.1</version> <dependencies> <dependency> <groupId>javax.mail</groupId> <artifactId>mail</artifactId> <version>1.4.3</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-core</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>${spring.version}</version> </dependency> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context-support</artifactId> <version>${spring.version}</version> </dependency> </dependencies> <properties> <spring.version>3.2.3.RELEASE</spring.version> </properties> </project>
Comments are closed for "Spring Email – Sending using GMail SMTP".
Hi Joe,
I’m getting the below error.
Invalid content was found starting with element ‘props’. One of ‘{“http://www.springframework.org/schema/beans”:meta, “http://www.springframework.org/schema/beans”:constructor-arg, “http://www.springframework.org/schema/beans”:property, “http://www.springframework.org/schema/beans”:qualifier, “http://www.springframework.org/schema/beans”:lookup-method, “http://www.springframework.org/schema/beans”:replaced-method, WC[##other:”http://www.springframework.org/schema/beans”]}’ is expected.
Please help.
Wonderful article.Thanks.
“For richer email template options we can use Velocity and I will cover that in a future article.”
Waiting for this….
Simple and Very Good Explanation.Wonderful article.
I’m getting the below error:
Exception in thread “main” org.springframework.mail.MailSendException: Mail server connection failed; nested exception is javax.mail.MessagingException: Could not connect to SMTP host: smtp.gmail.com, port: 587;
nested exception is:
java.net.ConnectException: Connection refused: connect. Failed messages: javax.mail.MessagingException: Could not connect to SMTP host: smtp.gmail.com, port: 587;
nested exception is:
java.net.ConnectException: Connection refused: connect; message exception details (1) are:
Failed message 1:
javax.mail.MessagingException: Could not connect to SMTP host: smtp.gmail.com, port: 587;
nested exception is:
java.net.ConnectException: Connection refused: connect
at com.sun.mail.smtp.SMTPTransport.openServer(SMTPTransport.java:1706)
at com.sun.mail.smtp.SMTPTransport.protocolConnect(SMTPTransport.java:525)
at javax.mail.Service.connect(Service.java:291)
at org.springframework.mail.javamail.JavaMailSenderImpl.doSend(JavaMailSenderImpl.java:389)
at org.springframework.mail.javamail.JavaMailSenderImpl.send(JavaMailSenderImpl.java:340)
at org.springframework.mail.javamail.JavaMailSenderImpl.send(JavaMailSenderImpl.java:336)
at com.javapapers.spring.SpringMailSender.sendMail(SpringMailSender.java:51)
at com.javapapers.spring.SpringEmail.main(SpringEmail.java:13)
Caused by: java.net.ConnectException: Connection refused: connect
at java.net.DualStackPlainSocketImpl.connect0(Native Method)
at java.net.DualStackPlainSocketImpl.socketConnect(DualStackPlainSocketImpl.java:79)
at java.net.AbstractPlainSocketImpl.doConnect(AbstractPlainSocketImpl.java:339)
at java.net.AbstractPlainSocketImpl.connectToAddress(AbstractPlainSocketImpl.java:200)
at java.net.AbstractPlainSocketImpl.connect(AbstractPlainSocketImpl.java:182)
at java.net.PlainSocketImpl.connect(PlainSocketImpl.java:172)
at java.net.SocksSocketImpl.connect(SocksSocketImpl.java:392)
at java.net.Socket.connect(Socket.java:579)
at java.net.Socket.connect(Socket.java:528)
at com.sun.mail.util.SocketFetcher.createSocket(SocketFetcher.java:284)
at com.sun.mail.util.SocketFetcher.getSocket(SocketFetcher.java:227)
at com.sun.mail.smtp.SMTPTransport.openServer(SMTPTransport.java:1672)
… 7 more
This is resolved.
why Gmail SMTP paramters are used in specific. Can u pls explain why smtp parameters are used
Can u please explain why gmail SMTP servers are used in specific
Hello sir,
can we fetch the recepients(to) email address from database. If so please share the code for the same.
Thank you
super
hello Sir,
I am using annotation not xml, please how do I achieve step 2 using annotation.
regards