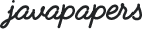
In this Java NIO tutorial let us learn about putting FileVisitor
and glob pattern matching into practical use. In the present Java NIO tutorial series we learnt about what is glob and how to use it. Then we saw about how to walk a file tree using NIO FileVisitor. Now let us combine these two and create a tool using which we can walk through the file system and search for a file or directory.
Glob is a pattern using which we can specify filenames. For example *.txt
means all files that has extension as txt. There are many wild card and characters available using them we can come up with powerful glob patterns.
FileVisitor
is an interface using which we can implement and walk through a file tree starting from a root path. SimpleFileVisitor
is a class provided as part of Java NIO API, which provides an implementation for FileVisitor
. This default implementation walks through all the files starting from the root path passed as argument. We can extend this class and add our glob pattern matching logic in it.
Our FileVisitor
is an extension of SimpleFileVisitor
. In visitFile
and preVisitDirectory
we will match the current path with the glob pattern passed. If the pattern matches then we will increment the match count and print the path.
package com.javapapers.java.nio; import java.io.IOException; import java.nio.file.FileSystems; import java.nio.file.FileVisitResult; import java.nio.file.Files; import java.nio.file.Path; import java.nio.file.PathMatcher; import java.nio.file.Paths; import java.nio.file.SimpleFileVisitor; import java.nio.file.attribute.BasicFileAttributes; public class SearchFile { public static class SearchFileVisitor extends SimpleFileVisitor<Path> { private final PathMatcher pathMatcher; private int matchCount = 0; SearchFileVisitor(String globPattern) { pathMatcher = FileSystems.getDefault().getPathMatcher( "glob:" + globPattern); } @Override public FileVisitResult visitFile(Path filePath, BasicFileAttributes basicFileAttrib) { if (pathMatcher.matches(filePath.getFileName())) { matchCount++; System.out.println(filePath); } return FileVisitResult.CONTINUE; } @Override public FileVisitResult preVisitDirectory(Path directoryPath, BasicFileAttributes basicFileAttrib) { if (pathMatcher.matches(directoryPath.getFileName())) { matchCount++; System.out.println(directoryPath); } return FileVisitResult.CONTINUE; } public int getMatchCount() { return matchCount; } } public static void main(String[] args) throws IOException { Path rootPath = Paths.get("."); String globPattern = "*.txt"; SearchFileVisitor searchFileVisitor = new SearchFileVisitor(globPattern); Files.walkFileTree(rootPath, searchFileVisitor); System.out.println("Match Count: " + searchFileVisitor.getMatchCount()); } }
.\temp.txt .\test\dummy1.txt .\test\dummy2.txt Match Count: 3