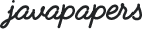
This Java NIO tutorial is to learn about walking a file tree. In a file handling application walking a file tree will be a regular requirement. Java NIO has given FileVisitor
interface using which we can walk through a file tree. This tutorial is part of the Java NIO tutorial series.
FileVisitor is an interface and we need to implement it to walk a file tree. FileVisitor has got the following four methods,
preVisitDirectory
– called before contents of a directory is visited.
postVisitDirectory
– called after contents of a directory is visited.
visitFile
– called on the file that is being visited.
visitFileFailed
– called when there is a failure on visiting a file.SimpleFileVisitor
is a class with default implementation to visit all files and on error it will re-throw errors. Instead of implementing FileVisitor
we can choose to extend SimpleFileVisitor
and override only methods of our need.
Once the FileVisitor is implemented we can use the NIO util class Files to start the File walk. Files class has got the following two methods,
walkFileTree(Path, FileVisitor)
– pass the FileVisitor instance and the root file tree to walk.
Path path = Paths.get("temp.txt"); SimpleFileVisitorExample fileVisitor = new SimpleFileVisitorExample(); Files.walkFileTree(path, fileVisitor);
walkFileTree(Path, Set<FileVisitOption>, int, FileVisitor)
– this method adds two more argument to the above method. One is to specify the number of levels to walk in the tree and another is to specify a set of options to customize the walking behavior. For example, we can specify option to visit symbolic link uing FileVisitOption
enum FOLLOW_LINKS
.
Path path = Paths.get("temp.txt"); EnumSetfileVisitOptions = EnumSet.of(FOLLOW_LINKS); Finder finder = new Finder(pattern); Files.walkFileTree(path, fileVisitOptions, Integer.MAX_VALUE, finder);
FOLLOW_LINKS
there is a possibility for circular link reference. If the walk gets trapped in loop then it will get reported in visitFileFailed
method by FileSystemLoopException
.We have good control over the file tree navigation. Every method returns FileVisitResult
and we can set the value to control the further action on the tree navigation using it. FileVisitResult
has the following values,
CONTINUE
– File tree walking should continue.
TERMINATE
– Abort the file tree walking.
SKIP_SUBTREE
– The directory and its subdirectories skipped when this value is returned by preVisitDirectory
.
SKIP_SIBLINGS
– When returned from preVisitDirectory
, it is same as previous option and postVisitDirectory
method also not called. If returned from postVisitDirectory, then no other directories in the same level are visited.Following example demonstrates the usage of above option. When a file named data.log is located, then the file walk is terminated.
@Override public FileVisitResult postVisitDirectory(Path path, IOException ioe) throws IOException { Path destinationPath = Paths.get("data.log"); if (path.getFileName().equals(destinationPath)) { System.out.println("File found: " + path); return FileVisitResult.TERMINATE; } return FileVisitResult.CONTINUE; }
Following file visitor just walks through the file tree starting from the path passed and prints the type of file. It CONTINUEs till the end of file tree.
package com.javapapers.java.nio; import static java.nio.file.FileVisitResult.CONTINUE; import java.io.IOException; import java.nio.file.FileVisitResult; import java.nio.file.Path; import java.nio.file.SimpleFileVisitor; import java.nio.file.attribute.BasicFileAttributes; public class SimpleFileVisitorExample extends SimpleFileVisitor<Path> { @Override public FileVisitResult visitFile(Path path, BasicFileAttributes basicFileAttributes) { if (basicFileAttributes.isRegularFile()) { System.out.println(path + " is a regular file with size " + basicFileAttributes.size()); } else if (basicFileAttributes.isSymbolicLink()) { System.out.println(path + " is a symbolic link."); } else { System.out.println(path + " is not a regular file or symbolic link."); } return CONTINUE; } @Override public FileVisitResult postVisitDirectory(Path path, IOException ioException) { System.out.println(path + " visited."); return CONTINUE; } @Override public FileVisitResult visitFileFailed(Path path, IOException ioException) { System.err.println(ioException); return CONTINUE; } }
Comments are closed for "Walk File Tree with Java NIO".
Perhaps you want to walk the file tree looking for a particular directory and, when found, you want the process to terminate. Perhaps you want to skip specific directories.