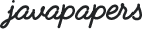
What is a Glob? In this Java NIO tutorial, we will go through what a glob is and how to use it with Java. This tutorial is part of the currently on going Java NIO tutorials series.
In programming, glob is a pattern using wildcards to specify filenames. For example, *.java
is a simple and trivial glob. It specifies all files with extension ‘java’. Two wildcard characters that are widely used are *
and ?
. Asterisk * stands for “any string of characters” and question mark ? stands for “any one character”.
Glob originated from Unix operating system. Unix provided a “global command” and it got abbreviated to glob. This is similar to regular expression but simpler in representation and limited in abilities.
Glob was introduced in Java as part of SE7 more features for NIO. In Java glob is used in FileSystem
class in PathMatcher getPathMatcher(String syntaxAndPattern)
method. A glob can be passed as an argument to get a PathMatcher. Similarly in Files
class glob can be used to iterate over the entries in a directory.
Following are some examples for “glob” pattern that can be used in Java NIO:
Glob | Description |
---|---|
*.txt | Matches all files that has extension as txt. |
*.{html,htm} | Matches all files that has extension as html or htm. { } are used to group patterns and , comma is used to separate patterns. |
?.txt | Matches all files that has any single charcter as name and extension as txt. |
*.* | Matches all files that has . in its name. |
C:\\Users\\* | Matches any files in C: ‘Users’ directory in Windows file system. Backslash is used to escape a special character. |
/home/** | Matches /home/foo and /home/foo/bar on UNIX platforms. ** matches strings of characters corssing directory boundaries. |
[xyz].txt | Matches a file name with single character ‘x’ or ‘y’ or ‘z’ and extension as txt. Square brackets [ ] are used to sepcify a character set. |
[a-c].txt | Matches a file name with single character ‘a’ or ‘b’ or ‘c’ and extension as txt. Hypehen – is used to specify a range and used in [ ] |
[!a].txt | Matches a file name with single character that is not ‘a’. ! is used for negation. |
Following is an example Java program to search for a glob pattern in a given directory and its sub directories. Here in this example we are searching for all files with extension .zip in D: in windows platform. If you are in UNIX or other platforms, you need to specify the path accordingly.
package com.javapapers.java.nio; import java.io.IOException; import java.nio.file.FileSystems; import java.nio.file.FileVisitResult; import java.nio.file.Files; import java.nio.file.Path; import java.nio.file.PathMatcher; import java.nio.file.Paths; import java.nio.file.SimpleFileVisitor; import java.nio.file.attribute.BasicFileAttributes; public class FileGlobNIO { public static void main(String args[]) throws IOException { String glob = "glob:**/*.zip"; String path = "D:/"; match(glob, path); } public static void match(String glob, String location) throws IOException { final PathMatcher pathMatcher = FileSystems.getDefault().getPathMatcher( glob); Files.walkFileTree(Paths.get(location), new SimpleFileVisitor<Path>() { @Override public FileVisitResult visitFile(Path path, BasicFileAttributes attrs) throws IOException { if (pathMatcher.matches(path)) { System.out.println(path); } return FileVisitResult.CONTINUE; } @Override public FileVisitResult visitFileFailed(Path file, IOException exc) throws IOException { return FileVisitResult.CONTINUE; } }); } }
D:\AndroidLocation.zip D:\Eclipse\7dec2014\eclipse-jee-kepler-R-win32-x86_64\workspace\.metadata\.mylyn\.tasks.xml.zip D:\Eclipse\7dec2014\eclipse-jee-kepler-R-win32-x86_64\workspace\.metadata\.mylyn\repositories.xml.zip D:\Eclipse\7dec2014\eclipse-jee-kepler-R-win32-x86_64\workspace\.metadata\.mylyn\tasks.xml.zip D:\mysql-workbench-community-6.2.5-winx64-noinstall.zip D:\workspace\Android\AndroidChatBubbles-master.zip D:\workspace\Android\Google Chat\XMPPChatDemo.zip D:\workspace\Android\Update-Android-UI-from-a-Service-master.zip D:\workspace\Android Chat\AndroidDialog.zip D:\workspace\Android Wear\AndroidWearPreview.zip
Comments are closed for "Glob with Java NIO".
Today only I noticed, my favourite JavaPapers theme design is back. Thanks Joe.
NIO tutorials are excellent. Looking forward to more in this series.
You are the best. So simple and easy to understand. Please write daily. Tnks
Hi joe,
I am happy with allblogs. But if you could assist me to start with practical I would be greateful.
Thanks
Naiya
The best Java blog for beginners! So lucky I found this. Thanks.
[…] Glob with Java NIO […]
[…] and glob pattern matching into practical use. In the present NIO tutorial series we learnt about what is glob and how to use it. Then we saw about how to walk a file tree using NIO FileVisitor. Now let us […]