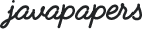
This web services tutorial is to learn about Java JAX-RS using the reference implementation Jersey. For this tutorial I will be using Eclipse (Kepler Version), Java JDK 1.8, Tomcat 7, JAX-RS 2.0 and Jersey 2.15. We will go through a step by step instruction to create an example RESTful web service application.
REST stands for Representational State Transfer. REST was a term coined by Roy Fielding in his doctoral dissertation. It is an architecture style for for creating network based applications. Key properties of REST are client-server communication, stateless protocol, cacheable, layered implementation and uniform interface. REST is sometimes seen as an alternate for SOAP. Refer the earlier written tutorial to understand the difference between REST and SOAP.
In REST architecture resources are accessed using an interface over HTTP or similar protocols. Mostly HTTP is used over methods GET, PUT, POST, DELETE wherein we can use other protocols also in REST architecture. Resources are uniquely identified using URI. Web services that conforms to the constraints of REST are called RESTful web services. To know about web service in general refer the web service introduction tutorial.
JAX-RS Specification is the Java API for RESTful web services. JAX-RS specification is the outcome of the Java Specification Request (JSR) 311, 339. JAX-RS uses the declarative style of programming using annotations. JAX-RS provides high level simpler API to write RESTful web services that can run on Java EE and SE platforms.
Jersey is the open source reference implementation of Java JAX-RS specification. It provides a Java library using which we can easily create RESTful web services in Java platform. JAX-RS / Jersey supports JAXB based XML bindings. JAXB provides API to access and process XML documents, to know more refer JAXB tutorial.
Download the Jersey distribution bundle from Jersey download page.
Let us now dive into the example RESTful web services project. It is a simple example, we will create a RESTful web service with a resource that will respond with hello world text.
I have used Eclipse WTP and Tomcat container. If you have an Eclipse with JEE perspective, then you are good to go.
Create a new dynamic web project using the Eclipse WTP wizard.
Just Click Next.
Just Click Next.
Enable the “Generate web.xml deployment descriptor” checkbox so that Eclipse will generate a web.xml.
Download the Jersey bundle from its website. It has a .zip file and it contains javax.ws.rs-api.jar then its dependencies and external dependencies. Remember to add all those three set of JARs to the lib folder in project as shown below.
Create the resource file as shown below in the Java sources.
package com.javapapers.webservices.rest.jersey; import javax.ws.rs.GET; import javax.ws.rs.Path; import javax.ws.rs.Produces; import javax.ws.rs.core.MediaType; @Path("/helloworld") public class HelloWorld { @GET @Produces(MediaType.TEXT_PLAIN) public String sayPlainTextHello() { return "Hello World RESTful Jersey!"; } @GET @Produces(MediaType.TEXT_XML) public String sayXMLHello() { return "<?xml version=\"1.0\"?>" + "<hello> Hello World RESTful Jersey" + "</hello>"; } @GET @Produces(MediaType.TEXT_HTML) public String sayHtmlHello() { return "<html> " + "<title>" + "Hello World RESTful Jersey" + "</title>" + "<body><h1>" + "Hello World RESTful Jersey" + "</body></h1>" + "</html> "; } }
Servlet mapping should be updated in the web.xml to point to our web service resource.
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0"> <display-name>RESTful Jersey Hello World</display-name> <welcome-file-list> <welcome-file>index.html</welcome-file> <welcome-file>index.htm</welcome-file> <welcome-file>index.jsp</welcome-file> <welcome-file>default.html</welcome-file> <welcome-file>default.htm</welcome-file> <welcome-file>default.jsp</welcome-file> </welcome-file-list> <servlet> <servlet-name>RESTful Jersey Hello World Service</servlet-name> <servlet-class>org.glassfish.jersey.servlet.ServletContainer</servlet-class> <init-param> <param-name>jersey.config.server.provider.packages</param-name> <param-value>com.javapapers.webservices.rest.jersey</param-value> </init-param> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>RESTful Jersey Hello World Service</servlet-name> <url-pattern>/rest/*</url-pattern> </servlet-mapping> </web-app>
“Run on Server” the web service application. The RESTful web service resource we created can be accessed from a browser as below,
As a add-on, lets have a look at how to consume this RESTful webservice using a Java Jersey client. Jersey provides a RESTful client library and using it we can consume the above web service.
package com.javapapers.webservices.rest.jersey; import java.net.URI; import javax.ws.rs.client.Client; import javax.ws.rs.client.ClientBuilder; import javax.ws.rs.client.WebTarget; import javax.ws.rs.core.MediaType; import javax.ws.rs.core.Response; import javax.ws.rs.core.UriBuilder; import org.glassfish.jersey.client.ClientConfig; public class RESTfulJerseyClient { private static final String webServiceURI = "http://localhost:8080/RESTful_Jersey_Hello_World"; public static void main(String[] args) { ClientConfig clientConfig = new ClientConfig(); Client client = ClientBuilder.newClient(clientConfig); URI serviceURI = UriBuilder.fromUri(webServiceURI).build(); WebTarget webTarget = client.target(serviceURI); // response System.out.println(webTarget.path("rest").path("helloworld").request() .accept(MediaType.TEXT_PLAIN).get(Response.class).toString()); // text System.out.println(webTarget.path("rest").path("helloworld").request() .accept(MediaType.TEXT_PLAIN).get(String.class)); // xml System.out.println(webTarget.path("rest").path("helloworld").request() .accept(MediaType.TEXT_XML).get(String.class)); // html System.out.println(webTarget.path("rest").path("helloworld").request() .accept(MediaType.TEXT_HTML).get(String.class)); } }
InboundJaxrsResponse{ClientResponse{method=GET, uri=http://localhost:8080/RESTful_Jersey_Hello_World/rest/helloworld, status=200, reason=OK}} Hello World RESTful Jersey! <?xml version="1.0"?><hello> Hello World RESTful Jersey</hello> <html> <title>Hello World RESTful Jersey</title><body><h1>Hello World RESTful Jersey</body></h1></html>
Comments are closed for "RESTful Web Services with Java JAX-RS using Jersey".
Joe, this is excellent. Please write more on RESTful web services.
Good tutorial
Easy to understand. Thanks
Hi Joe, This is simply awesome. Easy to understand a beginners.
Hi Joe, this is a good tutorial. It would be good if you give some examples about how to can secure the messages, like SOAP we have WS security.
3’S : Short-Simple-Sweet
Please write on HTTP POST method in Rest as well as Rest security
[…] REST stands for Representational State Transfer and it’s an architecture style for for creating network based applications. In my previous tutorial, we saw about a creating a hello world application on RESTful web services using Java JAX-RS in Jersey. […]
Hi Joe , it’s a nice tutorial, and it will be great if you write on other methods also( POST , DELETE, PUT )
You actually need this line to see the body of the request in the REST client:
System.out.println(r.readEntity(String.class));
The rest of the tutorial is just awesome. Congrats!
[…] the earlier written tutorial, RESTful Web Services with Java JAX-RS using Jersey to setup a basic hello world RESTful […]
Can you also include the step how to run the Client in order to consume the created web service
I downloaded the source code imported in eclipse and my tomcat is also working fine.. i am getting the error as
HTTP Status 404 – /RESTful_Jersey_Hello_World/rest/helloworld/
i even added all the jar files (Jersey)
need help !!!!
I downloaded the source code imported in eclipse and my tomcat is also working fine.. i am getting the error as
HTTP Status 404 – /RESTful_Jersey_Hello_World/rest/helloworld/
i even added all the jar files (Jersey)
need help !!!!
I think You need to give more explanation about org.glassfish.jersey.servlet.ServletContainer in web.xml and jercy client ,server.
Hi,
I am regularly following your web site. Thank You for contribution. I have created this project in Eclipse Luna. When i run this project, I got an error 404. I am using Apache Tomcat 8.
I have another doubt in Glass Fish Server in Netbean. When try to start GlassFish server, it keep on starting only.. not started. Looking forward your response.
Excellent
Please also write for JSON response using Webservices.
Dear Sir ,
I had done same development what you mentioned in the mail ,and included all jar’s what you are mentioned ,but when i am starting the apache tomact ,it is throwing the exception like java.lang.ClassNotFoundException: org.glassfish.jersey.servlet.ServletContainer. Can you suggest what is the exact issue please.
Dear Sir,
Please check my concern one time. Give your valuable suggestion
Hello sir,
Thanks for your coding.
I want to upload images through HTML page and those images store into webserver using Restful.Can you provide this code?
Thank you.
[…] This tutorial is part of the ongoing web services tutorial series. We will see about creating a RESTful web service using Jersey to produce JSON response. A RESTful web service can be configured to produce different types of response like XML, JSON, html, plain text and most of the MIME types. This tutorial will walk you through to setting up mime type to produce JSON response. If you are just starting with RESTful web services, I encourage you to go through my earlier tutorial an introduction to RESTful web services using Jersey. […]
Hi
Where do you take all those jar files?
it’s time-cosuming to downlaod all the dependent java jar files.
Thank you. It was simple and easy
i am getting the error as
HTTP Status 404 – /RESTful_Jersey_Hello_World/rest/helloworld/
Plzz help me resolve this error….
Thanks much, sir.
However, when I am restarting Tomcat by right clicking Server, I am getting this error mentioned below. Naturally I am getting error 404.
Can you please help? Thanks in advance.
SEVERE: Servlet [RESTful Jersey Hello World Service] in web application [/RESTful_Jersey_Hello_World] threw load() exception
java.lang.ClassCastException: com.javapapers.webservices.rest.jersey.HelloWorld cannot be cast to javax.servlet.Servlet
I have tried to generate exatly the same environment with same set of jars. But still am getting below exception
which is ClasNotFoundException.
java.lang.NoClassDefFoundError: org/jvnet/hk2/external/runtime/ServiceLocatorRuntimeBean
at org.glassfish.jersey.server.ApplicationHandler.initialize(ApplicationHandler.java:604)
at org.glassfish.jersey.server.ApplicationHandler.access$500(ApplicationHandler.java:184)
at org.glassfish.jersey.server.ApplicationHandler$3.call(ApplicationHandler.java:350)
at org.glassfish.jersey.server.ApplicationHandler$3.call(ApplicationHandler.java:347)
at org.glassfish.jersey.internal.Errors.process(Errors.java:315)
at org.glassfish.jersey.internal.Errors.process(Errors.java:297)
at org.glassfish.jersey.internal.Errors.processWithException(Errors.java:255)
at org.glassfish.jersey.server.ApplicationHandler.(ApplicationHandler.java:347)
at org.glassfish.jersey.servlet.WebComponent.(WebComponent.java:390)
at org.glassfish.jersey.servlet.ServletContainer.init(ServletContainer.java:172)
at org.glassfish.jersey.servlet.ServletContainer.init(ServletContainer.java:364)
at javax.servlet.GenericServlet.init(GenericServlet.java:158)
at org.apache.catalina.core.StandardWrapper.initServlet(StandardWrapper.java:1284)
at org.apache.catalina.core.StandardWrapper.loadServlet(StandardWrapper.java:1197)
at org.apache.catalina.core.StandardWrapper.load(StandardWrapper.java:1087)
at org.apache.catalina.core.StandardContext.loadOnStartup(StandardContext.java:5266)
at org.apache.catalina.core.StandardContext.startInternal(StandardContext.java:5554)
at org.apache.catalina.util.LifecycleBase.start(LifecycleBase.java:150)
at org.apache.catalina.core.ContainerBase$StartChild.call(ContainerBase.java:1575)
at org.apache.catalina.core.ContainerBase$StartChild.call(ContainerBase.java:1565)
at java.util.concurrent.FutureTask$Sync.innerRun(FutureTask.java:334)
at java.util.concurrent.FutureTask.run(FutureTask.java:166)
at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1145)
at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:615)
at java.lang.Thread.run(Thread.java:722)
Caused by: java.lang.ClassNotFoundException: org.jvnet.hk2.external.runtime.ServiceLocatorRuntimeBean
at org.apache.catalina.loader.WebappClassLoader.loadClass(WebappClassLoader.java:1720)
at org.apache.catalina.loader.WebappClassLoader.loadClass(WebappClassLoader.java:1571)
… 25 more