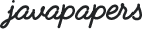
This tutorial is to create a java web service and client using Eclipse IDE. We will go by lazy way and use Eclipse wizard to generate the web service and client. Previously I wrote a tutorial to create a java web service using NetBeans and based on popular request I am writing this tutorial.
There are two ways to develop a web service namely top-down approach and bottom-up approach. To know about these approaches and in general about web service refer my introduction to web service tutorial posted earlier. We will be using bottom-up development approach in this tutorial.
Eclipse has many different versions and remember to use the Java EE IDE. It comes bundled with necessary plugins.
Just for your information, Eclipse by default uses Apache Axis to implement the web service and it provides option to use our choice of web service engine. I decided to go with the default bundled Apache Axis.
Use the new project from menu and open project wizard. Select ‘Dynamic Web Project’ and click next. Then give a project name and select a target runtime (I have Apache Tomcat v7.0) and leave all other default values and click next to finish.
If the run time is not already defined, then click New Runtime and select the version of Tomcat you have installed (already) then click next. Now browse the path of Tomcat home directory and click finish.
Create a new package under ‘Java Resources – src’ named com.javapapers.jee Then, create a new java class under that package. This is the web service’s service provider class.
package com.javapapers.jee; public class AnimalTypeService { public String animalType(String animal) { String animalType = ""; if ("Lion".equals(animal)) { animalType = "Wild"; } else if ("Dog".equals(animal)) { animalType = "Domestic"; } else { animalType = "I don't know!"; } return animalType; } }
I have used the above for my service. This is a simple service which will return type of animal based on argument passed.
With this step everything is over. If you have Eclipse it will take care of all the remaining work like creating web service, generating wsdl, skeleton, service client, stub and etc etc. Imagine how it would be if we have an Eclipse to help us in living our daily life. Eclipse for bathing, eating, … just sit and click eating is done. Crazy isn’t it? That is how the remainder of web service creation is going to be, just click click. IDE is helping us to do our job better but sometimes I think it makes us lazy. If you are a beginner, you must know what is happening behind the screens for every click in the Eclipse wizard.
Now the service class is ready and we need to create a web service using this java class.
Right click on ‘Java Resources’ -> New and select ‘Web Service’ under ‘Web Services’ folder from the wizard. Click Next button.
In this Web Service wizard, use the browse button and select the java class written earlier. Which is our service implementation java class.
Then, drag the slider bar to upper most in both service and client part.
Then, enable the Publish the Web service check box.
Added to configuring the service implementation, we are instructing Eclipse to generate a web service client also. This will create a dynamic web java project and create a web service client for the web service created. Instead we can also create a java project and write a client to access the web service.
Click Next till the Server Starup wizard and then click Start Server. This step will start the associated runtime Tomcat.
We can test the web service using a web service client. For this we need to create web service client application. In our previous configuration we moved the slider above in service configuration wizard which will create a web service client application.
There is one more way, Eclipse provides a Web Service Explorer. It is a nice client that will help us test a web service client.
Then click Next to reach ‘Test Web Service’ wizard and then click Launch button.
Then click next next and we will see the web service client application generated. See in the image below, EclipseWebServiceClient is a web application generated by Eclipse.
Do not stop with this. Open the EclipseWebServiceClient application and go through the code and generated Stub, Proxy and all the files. It will really help to understand more.
Download Eclipse based Web Service Source Code
Download Eclipse based Web Service Client Source Code
Comments are closed for "Java Web Service using Eclipse".
Nice Example
So simple. Gr8 n thanx for the information.
Crispy and clear. Thanks for your info.
Hi Joe you make everything easier and simple. Continue! :D
Too Good !!! Very Simple to understand !!! Many Thanks !! Keep it up !!
Eclipse does everything for us..without eclipse developers life wud have been hell..
Nice tutorial..!!
Thanks for the clear crispy and summary tutorial. It is really helpful for me because I don’t like to read big detailed books so I have chosen your tutorial. thanks for your posts.
Regards,
Mouli K
I think here we used JAX-RPC(I donot know why we have to use JAX-RPC, do we have any other option which will work for any technology), Would you able to explain how JAS-WS make calls to java service the methods, I am bit confused with, how really xml to java object initiation happens.
Hi sorry, I am keep on asking questions. When I try with list of objects to return my webservice I am getting below error.
“java.util.List”, that is not supported by the JAX-RPC specification. Instances of the type may not serialize or deserialize correctly. Loss of data or complete failure of the Web service may result.
nice and clear explanation.This tutorial saves a lot of time.looking forward for further topics..
thnks
This is a nice tutorial and easy to understand.But I’m looking for Webservice using Eclipse and AXIS2.Need the tutorial of the same.
Thanks for your efforts..
Good work Joe.. keep it up.
What is the use by implementing the above service as a web service rather than implementing it as a simple website by creating a html input tag and then submit the request to a php or jsp page where the server sends you a response saying it is wild or domestic or what ever ..PLEASE COMMENT/REPLY
if possible please explain the code and generated Stub, Proxy and all the files.
we should understand the underlying technology and whats happening at the background.we should not rely or depend on IDEs. Today u work in one IDE but tomorrow another and again another..u should not learn the procedure in IDE ..u need to understand the underlying technology and architecture.PLEASE EXPLAIN
Nice sirji!!!
Thanks a lot !!!
Create my first WebService
Nice sirji!!!
Thanks a lot !!!
Created my first WebService
when i create the web service it gives mw message “Unable to add the follwing facets to project TestWebServices: Axis2 Web Services Extensions, Axis2 Web Services Core.”
Really nice and greate toturial for beginner. I expecting toturials from EJB 3.
GREAT WORK !!
Hi Joe,
when I am trying to “select service implementation”, I am getting the error “IWAB0489E Error when deploying web service to Axis runtime”.
I am using the same eclipse version and release you are using in this tutorial. Kindly provide any pointer;its urgent!!
TIA!
now getting the error in Web Service Explorer:
IWAB0379E Unable to open http://localhost:9090/soapTest/services/AnimalTypeService?wsdl.
IWAB0135E An unexpected error has occurred.
WSDLException
WSDLException: faultCode=OTHER_ERROR: http://localhost:9090/soapTest/services/AnimalTypeService?wsdl
Hello dear How to “go through the code and generated Stub, Proxy and all the files”.
I did the same and i get the Result as
com.javapapers.jee.AnimalTypeSoapBindingStub@11650d6
I want to know input the class,method or Dog or Lion.. pls expain it….
i want to pass json object from webservice csn u suggest me plzzzz
Hi Joe,
Thanks for the post.. It is very simple and easy to understand.
I got it. But when I am trying to “select service implementation”, I am getting the error “IWAB0489E Error when deploying web service to Axis runtime”.
could you please suggest the solution for this problem..
Thanks in advance.
Thanks Joe for a very wonderful explanation and demonstration.
Can you please execute some more example on database driven web services using axis2?
Sure, will write more on this soon.
Hi Joe,
following are the issues which i am facing…
1.I have created the AnimalTypeService.
I am facing the issuew while running the next step as follows.
While starting the server as follows.
IWAB0489E Error when deploying Web service to Axis runtime
axis-admin failed with {http://xml.apache.org/axis/}HTTP (404)/Proj_jp_web/services/AdminService
The error on the console is as follows.
21:42:02,025 ERROR [MainDeployer] Could not initialise deployment: file:/C:/jbos
s-4.2.3.GA/server/default/deploy/Proj_jp_webEAR.ear
org.jboss.deployment.DeploymentException: No META-INF/application.xml found
2.How the wsdl find is generated in the background
Thanks in advance….
Hi Joe,
It would be more useful if you reply for the doubts, people asked.
Thanks.
Very nice, with the eclipse juno after choose the class AnimalTypyeService and set the bar both in Test, It put you in last window for test.
Hi Joe,
With this step, we can develop a big web service where access database
This is great tutorial for the beginner likes me.!!
but please describe more about how it’s working behind of IDE implementation,how I can do all process in webservice creating such as wsdl ,coding stub etc. And I want to know how can I use json files with the webservice .
Thank’s a lot Joe. You’re my great teacher!!
Hi,
Ur tutorial is so useful.Can u say me How to Create a Web services in distributed environment
We are created web services url using eclipse axis 2 IDE and also deployed into jboss server.
Every thing working fine, but server logs are getting warnings like
”
WARN[org.apache.axis2.classloader.BeanInfoCache] Unable to locate a BeanInfo cache for class webService.greenPages.Category (stopClass=class java.lang.Object). This will negatively affect performance!
”
Any body idea on this.
Good explanation. Keep it up
Sir, Is there any possibility to connect two web service in eclipse. If so,how can we do it?
Hi joe,
I tried a lot . But i am not able to create
a webservice . In start server page when i
am trying to start the server it is not able to start properly .
next button is not enabling .serverconfig.xml also
Not generating . I am using jboss 4.0.2
Plz help
Hi both JAX-RPC,JAX_WS are same ,the differ is JAX_RPC is deprecate one from JDK1.5 so if you are using jdk1.5 or 6.0 then you have to use JAX_WS
This helpful but suppose the i m going to write a web method in webservice containing a log4j logger object then it is not working it is throwing exception like …
soapenv:Server.userException
java.lang.reflect.InvocationTargetException
Can u hep me in this
Hi Joe,:)
I found this blog really useful, was wondering if you will be interested in teaching online too?
Thanks
Very Nice Example….
Thanks a lot
excellent example by JOE..it will be usefull for all newbies in webservices
Joe,
I am getting this error
“IWAB0489E Error when deploying Web service to Axis runtime”
I am using JBoss 4.2 server.
I am getting this error “IWAB0489E Error when deploying Web service to Axis runtime”
I am using Jboss 4.2 server
I have dynamic web project created by WSDL file using AXIS2 facet. Now i want to integrate this project to other project as a jar file. So that i can add this jar file into lib of other project.
Appreciate any help… thanks
Regs
Laxman
how to invoke web service from html?
nice and simple to understand
Thanks Joe. You have explained in simple and crispy manner.
Hi Joe,
This tutorial is very nice and helpful.
Thanks a lot.
Keep it on..
simple and good example..
Thank you
Iam very happy to learn how can i create a yummy web service from this good example
thank u very much my friend
[…] web services in Java. We saw about SOAP based hello world web services and how to create them using Eclipse and Netbeans. Now this tutorial is about getting started with Axis2 based webservice using […]
nice example :D .. thanks
Could you please tell me how to use the web service thus created in javascript?
Hi joe, This is really helpful.
Thanks,
Above post is helpfull..thanks..Keep going.
I’m trying to create WSDL using MyEclipse 10 taking JAX-WS(Websphere)Version 2.1
Here in my code, I’m passing File as a input parameter but when I try to generate WSDL I get the following error, Please help me if there is a way to pass input as file(java.io.File)
The following warnings were generated while running WSDL2Java. Press Cancel to abort the generation process.
Jun 4, 2013 10:46:25 AM com.ibm.ws.webservices.wsdl.fromJava.BeanWriter isBeanCompatible
WARNING:
WSWS3292W: Warning: The class java.io.File is defined in a java or javax package and cannot be converted into an xml schema type. An xml schema anyType will be used to define this class in the wsdl file.
WSWS3292W: Warning: The class java.io.File is defined in a java or javax package and cannot be converted into an xml schema type. An xml schema anyType will be used to define this class in the wsdl file.
WSVR0615W: The user.install.root system property is not set. Some product classes might not be found.
WSWS3752I: (C) COPYRIGHT International Business Machines Corp. 1997, 2008.
WSWS3753I: IBM WebSphere Application Server Release 7.0
WSWS3754I: Web services Java2WSDL emitter.
Sorry here I’m using JAX-RPC(Websphere)
really good !!
Great.. Saved my lot of time ..Thanks a lot !!
it’s very useful for beginner’s
it’s simple and Awesome..Thank you
Thanks JOE,it is really good explanation for beginners.
i need the result in xml…and the invocation by typing url in the address bar..how can it be done..???
can anyone please help me..
I got the same error: “Error when deploying Web service to Axis runtime”.
The issue was…the default tomcat port 8080 is already in use, when i changed that…i could directly execute the example….thanks joe
Thanks for the clear crispy and summary tutorial.
just one question, when the web service created, why i get a JSR-109 Web Services project in the Project Explorer? and even if I delete my web service project, the JSR-109 Web Services still exist and can not do anything on it(delete, close ), would You have time helping me to solve this question?
Hi,
I have tried to create the web service, but as you said after creating the service class I have tried to create the web service but it’s not showing the service class in the browsing pop can any one suggest.
How to check the web service without creating client application? I tried to check using Web Services Explorer. I got the following error in status
IWAB0135E An unexpected error has occurred.
WSDLException
WSDLException: faultCode=INVALID_WSDL: : The end-tag for element type “FRAME
Please help me…….
Thanks a lot joe its really very full for me….
Great!!! Thank you very much for this. It’s really very much useful for the beginners.
Hi,
I’m having a problem while create the web service.After my server start to run I’m getting Error like ‘IWAB0489E Error when deploying Web service to Axis runtime ‘ .Can you please tell me how to fix this?
Hi,
I am using Juno Version, but when i try to deploy the webService.
Getting a Error:
IWAB0489E Error when deploying Web service to Axis runtime.
Please need your help regarding this issue.
it’s really superb
Thank for this tutorial.
I have created a web service using the same but when I am trying to run it another time after closing it, get an error of 404. So do I need to overwrite the created web service or any other thing(s) needed to be done in order to run again that web service.
Thanks a lot! This is very helpfull
Hi Joe,
What I need to do to return JSON instead of XML?
Andrew
I created a web service but i donot see any WSDL. Please help
you have not given completely instruction about creating webclient …i am not getting how to creat webclient application for testing webservices
Thanks Ankit.
Yes I have plans to teach online. I will announce that soon in javapapers. Please wait for that.
Hello Joe, I am new to web service creation with Eclipse and your blog was very useful.
I have the following concerns and I need your help:
1. What is the difference between a dynamic project, webservice project and a web application project in Eclipse?
2. Which kind of project is used when/where?
3. When and Where should I use JAX-WS? because, the dynamic project in your tutorial dint need that.
4. To modify/check SOAP headers, do I need servlets and handlers and a whole different configuration of webservice?
Eclipse has automated everything that I am not able to understand when what happens where.
5. Could you please provide such a tutorial (or link to one similar) for creating a (common) webservice to send push notifications to GCM/APNS/BB depending upon a set of parameters from client app?
1. Those are all example project templates. It comes with default folder structure and support for deployment/debug.
2. dynamic web project – when you create a web application, webservice – its in the name.
3. Yes, to learn it is better not to use the IDE support. Just handcode every file by yourself.
4. Sure, added to my to do list.
Thanks guys.
Welcome Chakri.
good one!!!
Perfect example… NO issues in creating this if we follow the steps mentioned.. Thanks a lot
Hello
I have to make a web service on java plateform, but I had no idea how to do it; this article is awesome.
can you tell me how to run web service without using a server.
thanks
Very good joe,
it will be better if you explain clearly even the background process as well.
It will be very helpful for beginners
Awesome
Hi Joe,
when following the above steps, I am getting the below error when Typing Lion in the animal textbox.
Exception: org.apache.axis.InternalException: java.lang.Exception: Couldn’t find a matching Java operation for WSDD operation “animalType” (1 args) Message: org.apache.axis.InternalException: java.lang.Exception: Couldn’t find a matching Java operation for WSDD operation “animalType” (1 args) .
Could you please explain where the issue is occurring from, whether the server class or client class. Also please explain me how to generate stubs and proxy class.
Thanks
Anil
Awesome tutorial. Very well explained for newbies
pls… help..me how to get last window…
hi sir,
Is it possible to use swing application json data in web service.
i want to do a project on web services in java ….can u plz suggest me project titles on which I can work
Very Very Good example. NO issues in creating this if we follow the steps mentioned.
Thanks a lot
Lava Kumar
Thank you, nice and simple
HiJoe,
Example is nice.Am very new to webservices,here in your example service we have created ,client also created. But to consume that service we need to add some classes in client.So that we can check we can consume the service.
please let me know that procedure
Very Nice !!!!
Thanks you for this simple demo….
Hi, Is it possible to create web service for swing application which possess event listeners and not web methods
Dear Joe,
I have the the same way u explained above but after clicking the start server i receiving this error “IWAB0489E Error when deploying Web service to Axis runtime” kindly assist me with this
Dear Joe,
I have followed the same way u explained above but after clicking the start server i receive the error “IWAB0489E Error when deploying Web service to Axis runtime” kindly assist me with this
nice example thanks for your help.
Chandan
Hi,
This example is really helpful.
can u please give some tutorial on webservices developed using Apache CXF + Spring in Eclipse.
Thanks
Yash
It works like charm! No glitch by following the document.
u can resolve that problem, go to windows ->preferrence and select network connections and disable proxy settings(http)
Hi guys you can resolve that problem, go to windows ->preferrence and select network connections and disable proxy settings(http) or change active provider native to direct like.
Nice example. i like it. can u share more web services examples..
hi sir
i created a web service in java and trying to cal from dot net c#
when i’m adding wsdl reference i’m getting the error in tomcat console.
org.apache.axis.transport.http.AxisServlet getSoapAction
SEVERE: Generating fault class
AxisFault
faultCode: {http://xml.apache.org/axis/}Client.NoSOAPAction
faultSubcode:
faultString: no SOAPAction header!
faultActor:
faultNode:
faultDetail:
{http://xml.apache.org/axis/}stackTrace:no SOAPAction header!
at org.apache.axis.transport.http.AxisServlet.getSoapAction(AxisServlet.java:1013)
at org.apache.axis.transport.http.AxisServlet.doPost(AxisServlet.java:678)
at javax.servlet.http.HttpServlet.service(HttpServlet.java:641)
at org.apache.axis.transport.http.AxisServletBase.service(AxisServletBase.java:327)
at javax.servlet.http.HttpServlet.service(HttpServlet.java:722)
at org.apache.catalina.core.ApplicationFilterChain.internalDoFilter(ApplicationFilterChain.java:305)
at org.apache.catalina.core.ApplicationFilterChain.doFilter(ApplicationFilterChain.java:210)
at org.apache.catalina.core.StandardWrapperValve.invoke(StandardWrapperValve.java:222)
at org.apache.catalina.core.StandardContextValve.invoke(StandardContextValve.java:123)
at org.apache.catalina.authenticator.AuthenticatorBase.invoke(AuthenticatorBase.java:472)
at org.apache.catalina.core.StandardHostValve.invoke(StandardHostValve.java:168)
at org.apache.catalina.valves.ErrorReportValve.invoke(ErrorReportValve.java:99)
at org.apache.catalina.valves.AccessLogValve.invoke(AccessLogValve.java:929)
at org.apache.catalina.core.StandardEngineValve.invoke(StandardEngineValve.java:118)
at org.apache.catalina.connector.CoyoteAdapter.service(CoyoteAdapter.java:407)
at org.apache.coyote.http11.AbstractHttp11Processor.process(AbstractHttp11Processor.java:1002)
at org.apache.coyote.AbstractProtocol$AbstractConnectionHandler.process(AbstractProtocol.java:585)
at org.apache.tomcat.util.net.JIoEndpoint$SocketProcessor.run(JIoEndpoint.java:310)
at java.util.concurrent.ThreadPoolExecutor.runWorker(Unknown Source)
at java.util.concurrent.ThreadPoolExecutor$Worker.run(Unknown Source)
at java.lang.Thread.run(Unknown Source)
{http://xml.apache.org/axis/}hostname:1-PC
no SOAPAction header!
at org.apache.axis.transport.http.AxisServlet.getSoapAction(AxisServlet.java:1013)
at org.apache.axis.transport.http.AxisServlet.doPost(AxisServlet.java:678)
at javax.servlet.http.HttpServlet.service(HttpServlet.java:641)
at org.apache.axis.transport.http.AxisServletBase.service(AxisServletBase.java:327)
at javax.servlet.http.HttpServlet.service(HttpServlet.java:722)
at org.apache.catalina.core.ApplicationFilterChain.internalDoFilter(ApplicationFilterChain.java:305)
at org.apache.catalina.core.ApplicationFilterChain.doFilter(ApplicationFilterChain.java:210)
at org.apache.catalina.core.StandardWrapperValve.invoke(StandardWrapperValve.java:222)
at org.apache.catalina.core.StandardContextValve.invoke(StandardContextValve.java:123)
at org.apache.catalina.authenticator.AuthenticatorBase.invoke(AuthenticatorBase.java:472)
at org.apache.catalina.core.StandardHostValve.invoke(StandardHostValve.java:168)
at org.apache.catalina.valves.ErrorReportValve.invoke(ErrorReportValve.java:99)
at org.apache.catalina.valves.AccessLogValve.invoke(AccessLogValve.java:929)
at org.apache.catalina.core.StandardEngineValve.invoke(StandardEngineValve.java:118)
at org.apache.catalina.connector.CoyoteAdapter.service(CoyoteAdapter.java:407)
at org.apache.coyote.http11.AbstractHttp11Processor.process(AbstractHttp11Processor.java:1002)
at org.apache.coyote.AbstractProtocol$AbstractConnectionHandler.process(AbstractProtocol.java:585)
at org.apache.tomcat.util.net.JIoEndpoint$SocketProcessor.run(JIoEndpoint.java:310)
at java.util.concurrent.ThreadPoolExecutor.runWorker(Unknown Source)
at java.util.concurrent.ThreadPoolExecutor$Worker.run(Unknown Source)
at java.lang.Thread.run(Unknown Source)
Feb 14, 2014 10:32:53 AM org.apache.axis.transport.http.AxisServlet getSoapAction
SEVERE: Generating fault class
AxisFault
faultCode: {http://xml.apache.org/axis/}Client.NoSOAPAction
faultSubcode:
faultString: no SOAPAction header!
faultActor:
faultNode:
faultDetail:
{http://xml.apache.org/axis/}stackTrace:no SOAPAction header!
at org.apache.axis.transport.http.AxisServlet.getSoapAction(AxisServlet.java:1013)
at org.apache.axis.transport.http.AxisServlet.doPost(AxisServlet.java:678)
at javax.servlet.http.HttpServlet.service(HttpServlet.java:641)
at org.apache.axis.transport.http.AxisServletBase.service(AxisServletBase.java:327)
at javax.servlet.http.HttpServlet.service(HttpServlet.java:722)
at org.apache.catalina.core.ApplicationFilterChain.internalDoFilter(ApplicationFilterChain.java:305)
at org.apache.catalina.core.ApplicationFilterChain.doFilter(ApplicationFilterChain.java:210)
at org.apache.catalina.core.StandardWrapperValve.invoke(StandardWrapperValve.java:222)
at org.apache.catalina.core.StandardContextValve.invoke(StandardContextValve.java:123)
at org.apache.catalina.authenticator.AuthenticatorBase.invoke(AuthenticatorBase.java:472)
at org.apache.catalina.core.StandardHostValve.invoke(StandardHostValve.java:168)
at org.apache.catalina.valves.ErrorReportValve.invoke(ErrorReportValve.java:99)
at org.apache.catalina.valves.AccessLogValve.invoke(AccessLogValve.java:929)
at org.apache.catalina.core.StandardEngineValve.invoke(StandardEngineValve.java:118)
at org.apache.catalina.connector.CoyoteAdapter.service(CoyoteAdapter.java:407)
at org.apache.coyote.http11.AbstractHttp11Processor.process(AbstractHttp11Processor.java:1002)
at org.apache.coyote.AbstractProtocol$AbstractConnectionHandler.process(AbstractProtocol.java:585)
at org.apache.tomcat.util.net.JIoEndpoint$SocketProcessor.run(JIoEndpoint.java:312)
at java.util.concurrent.ThreadPoolExecutor.runWorker(Unknown Source)
at java.util.concurrent.ThreadPoolExecutor$Worker.run(Unknown Source)
at java.lang.Thread.run(Unknown Source)
{http://xml.apache.org/axis/}hostname:1-PC
no SOAPAction header!
at org.apache.axis.transport.http.AxisServlet.getSoapAction(AxisServlet.java:1013)
at org.apache.axis.transport.http.AxisServlet.doPost(AxisServlet.java:678)
at javax.servlet.http.HttpServlet.service(HttpServlet.java:641)
at org.apache.axis.transport.http.AxisServletBase.service(AxisServletBase.java:327)
at javax.servlet.http.HttpServlet.service(HttpServlet.java:722)
at org.apache.catalina.core.ApplicationFilterChain.internalDoFilter(ApplicationFilterChain.java:305)
at org.apache.catalina.core.ApplicationFilterChain.doFilter(ApplicationFilterChain.java:210)
at org.apache.catalina.core.StandardWrapperValve.invoke(StandardWrapperValve.java:222)
at org.apache.catalina.core.StandardContextValve.invoke(StandardContextValve.java:123)
at org.apache.catalina.authenticator.AuthenticatorBase.invoke(AuthenticatorBase.java:472)
at org.apache.catalina.core.StandardHostValve.invoke(StandardHostValve.java:168)
at org.apache.catalina.valves.ErrorReportValve.invoke(ErrorReportValve.java:99)
at org.apache.catalina.valves.AccessLogValve.invoke(AccessLogValve.java:929)
at org.apache.catalina.core.StandardEngineValve.invoke(StandardEngineValve.java:118)
at org.apache.catalina.connector.CoyoteAdapter.service(CoyoteAdapter.java:407)
at org.apache.coyote.http11.AbstractHttp11Processor.process(AbstractHttp11Processor.java:1002)
at org.apache.coyote.AbstractProtocol$AbstractConnectionHandler.process(AbstractProtocol.java:585)
at org.apache.tomcat.util.net.JIoEndpoint$SocketProcessor.run(JIoEndpoint.java:312)
at java.util.concurrent.ThreadPoolExecutor.runWorker(Unknown Source)
at java.util.concurrent.ThreadPoolExecutor$Worker.run(Unknown Source)
at java.lang.Thread.run(Unknown Source)
Joe,
Simple example, neatly explained. Easy thing to the starters. Good Work. Please keep it up.
It is not possible to run a service without a server, how can waiter serve you if he is not present there itself for your service
https://geronimo.apache.org/GMOxDOC30/developing-a-simple-calculator-web-service.html#DevelopingasimpleCalculatorWebservice-CreatingtheWebservicesimplementationcode
after doing all the things right when i create a web service im getting error like
“Unable to add the follwing facets to project Ws: Axis2 Web Services Extensions, Axis2 Web Services Core”
I have no idea about it..plz help
hi Joe,
it’s a nice explanation.I developed the web service in netbeans and added more functions.
but it’s the first time I’m developed the web service using eclipse . But dont know how to add new method in same web service and getting targetNamespace and wsdl url. i need targetNamespace and wsdl url because my client is an android phone.
can you please give me a solution to add new method in same web service and how to get wsdl url and name space.
thanks
hi Joe,
it’s a nice explanation.I developed the web service in netbeans and added more functions.
but it’s the first time I’m developed the web service using eclipse . But dont know how to add new method in same web service and getting targetNamespace and wsdl url. i need targetNamespace and wsdl url because my client is an android phone.
can you please give me a solution to add new method in same web service and how to get wsdl url and name space. if u can could u please send the answer to my id : srijinits@gmail.com
thanks
Thanks a lot. it’s a nice explanation.I developed the web service in netbeans and added more functions.
At place of Web Service Explorer, when I am clicking page opens with
HTTP 500: error
and explorer of eclipse sends message
“An error occurred. Unable to launch WSDL test facility.”
I have tried on windows 7 and Ubuntu but no success.
Could you please give me the clue??
Thanks.
Things I forgot to mention are:
I am using
Eclipse 4.3.1 Kepler SR1
JDK 8
Tomcat 7.0.50.
Nicely explained
Joe, Could you please explain how we develop soap webservices(bottom up approach) in weblogic server in eclipse. please help as soon as possible.
I get HTTP ERROR: 500
after I follwed all the steps from your posting. Can you please let me know what my mistake is? Appreciate your kind help.
Thanks
good post dear
anyone can easily learn through this
Having the same problem mayur. Have tried a bunch of examples same results, been workign on this work like a week, real fustrating. Using JDK 1.8, tomcat 7.0.54, eclipse Kepler. It’s looking like a problem with eclipse versions maybe with compatability with dependant SW?
The service does not deploy, I see no WSDL generated and the AAR is the same version.aar from axis2 and seems ot make a copy of it in the project directory and it’s contents are the same from like 2012 when you open the aar.
~/webservices/CrunchifyWS/WebContent$ jar vft ./WEB-INF/services/version.aar
0 Tue Apr 17 18:16:50 EDT 2012 META-INF/
125 Tue Apr 17 18:16:48 EDT 2012 META-INF/MANIFEST.MF
0 Tue Apr 17 18:16:48 EDT 2012 sample/
0 Tue Apr 17 18:16:48 EDT 2012 sample/axisversion/
655 Tue Apr 17 18:16:48 EDT 2012 sample/axisversion/Version.class
1236 Tue Apr 17 18:16:48 EDT 2012 META-INF/NOTICE
1159 Tue Apr 17 18:16:48 EDT 2012 META-INF/services.xml
11359 Tue Apr 17 18:16:48 EDT 2012 META-INF/LICENSE
0 Tue Apr 17 18:16:50 EDT 2012 META-INF/maven/
0 Tue Apr 17 18:16:50 EDT 2012 META-INF/maven/org.apache.axis2/
0 Tue Apr 17 18:16:50 EDT 2012 META-INF/maven/org.apache.axis2/version/
3610 Tue Apr 17 17:29:02 EDT 2012 META-INF/maven/org.apache.axis2/version/pom.xml
108 Tue Apr 17 18:16:48 EDT 2012 META-INF/maven/org.apache.axis2/version/pom.properties
Joe,
Thanks for sharing such an easy tout..
-Guru
I had the same problem. I am using
apache-tomcat-7.0.54
axis2-1.6.2
eclipse kepler service release 2.
Let me know if anybody is able to solve the issue.
Hi Joe,
Your tutorials are really simple and straight forward and I like it.
In your service class you have String animalType = “”; Please assign the animal parameter to the variable in order to get the desired output.
Thanks for the tutorial.
thanks joe..you are doing awesome job for developers and new bie. This is a great tutorial for creating simple web service.
nice and simple; thank you
I followed your instructions, and got a ClassNotFoundException on sampleAnimalTypeProxy.Result.jsp What do I do now?
Can you post that exception trace for more information?