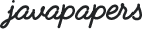
REST stands for Representational State Transfer and it’s an architecture style for for creating network based applications. In my previous tutorial, we saw about a creating a hello world application on RESTful web services using Java JAX-RS in Jersey.
JAX-RS is the Java API specification and Jersey is its reference implementation. This tutorial is a continuation in that series. We will see how to create a CRUD (Create, Read, Update and Delete) application in RESTful services with Java JAX-RS using Jersey. To know about web services in general, read the introduction to web services tutorial.
Create a Dynamic web application. I am using Eclipse EE edition. Refer the previous tutorial to do this step by step. Then copy all the Jersey JAR files into the lib folder. Jersey and its dependent JAR files are available in its download bundle from its official site.
Following is the data model for this RESTful CRUD services.
package com.javapapers.webservices.rest.jersey; import javax.xml.bind.annotation.XmlRootElement; @XmlRootElement public class Animal { private String id; private String name; private String type; public Animal() { } public Animal(String id, String name, String type) { super(); this.id = id; this.name = name; this.type = type; } public String getId() { return id; } public void setId(String id) { this.id = id; } public String getName() { return name; } public void setName(String name) { this.name = name; } public String getType() { return type; } public void setType(String type) { this.type = type; } }
Following is a RESTful web service resource file. This resource demonstrates the basic GET, PUT and DELETE operations. Jersey annotations are used to mark each method for its type and content response media-type.
package com.javapapers.webservices.rest.jersey; import javax.ws.rs.Consumes; import javax.ws.rs.DELETE; import javax.ws.rs.GET; import javax.ws.rs.PUT; import javax.ws.rs.Produces; import javax.ws.rs.core.Context; import javax.ws.rs.core.MediaType; import javax.ws.rs.core.Request; import javax.ws.rs.core.Response; import javax.ws.rs.core.UriInfo; import javax.xml.bind.JAXBElement; public class AnimalResource { @Context UriInfo uriInfo; @Context Request request; String id; AnimalService animalService; public AnimalResource(UriInfo uriInfo, Request request, String id) { this.uriInfo = uriInfo; this.request = request; this.id = id; animalService = new AnimalService(); } @GET @Produces({ MediaType.APPLICATION_XML, MediaType.APPLICATION_JSON }) public Animal getAnimal() { Animal animal = animalService.getAnimal(id); return animal; } @GET @Produces(MediaType.TEXT_XML) public Animal getAnimalAsHtml() { Animal animal = animalService.getAnimal(id); return animal; } @PUT @Consumes(MediaType.APPLICATION_XML) public Response putAnimal(JAXBElement<Animal> animalElement) { Animal animal = animalElement.getValue(); Response response; if (animalService.getAnimals().containsKey(animal.getId())) { response = Response.noContent().build(); } else { response = Response.created(uriInfo.getAbsolutePath()).build(); } animalService.createAnimal(animal); return response; } @DELETE public void deleteAnimal() { animalService.deleteAnimal(id); } }
Method | Description |
---|---|
GET | Is used to read from a REST resource. |
PUT | Is used for Create/Updating a REST resource. |
POST | Generally used for new resource creation. |
DELETE | To delete a REST resource. |
This REST resource maps to the path given in annotation “/animals”.
package com.javapapers.webservices.rest.jersey; import java.io.IOException; import java.util.List; import javax.servlet.http.HttpServletResponse; import javax.ws.rs.Consumes; import javax.ws.rs.FormParam; import javax.ws.rs.GET; import javax.ws.rs.POST; import javax.ws.rs.Path; import javax.ws.rs.PathParam; import javax.ws.rs.Produces; import javax.ws.rs.core.Context; import javax.ws.rs.core.MediaType; import javax.ws.rs.core.Request; import javax.ws.rs.core.UriInfo; @Path("/animals") public class AnimalsResource { @Context UriInfo uriInfo; @Context Request request; AnimalService animalService; public AnimalsResource() { animalService = new AnimalService(); } @GET @Produces({ MediaType.APPLICATION_XML, MediaType.APPLICATION_JSON }) public List<Animal> getAnimals() { return animalService.getAnimalAsList(); } @GET @Produces(MediaType.TEXT_XML) public List<Animal> getAnimalsAsHtml() { return animalService.getAnimalAsList(); } // URI: /rest/animals/count @GET @Path("count") @Produces(MediaType.TEXT_PLAIN) public String getCount() { return String.valueOf(animalService.getAnimalsCount()); } @POST @Produces(MediaType.TEXT_HTML) @Consumes(MediaType.APPLICATION_FORM_URLENCODED) public void createAnimal(@FormParam("id") String id, @FormParam("animalname") String name, @FormParam("animaltype") String type, @Context HttpServletResponse servletResponse) throws IOException { Animal animal = new Animal(id, name, type); animalService.createAnimal(animal); servletResponse.sendRedirect("./animals/"); } @Path("{animal}") public AnimalResource getAnimal(@PathParam("animal") String id) { return new AnimalResource(uriInfo, request, id); } }
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_3_0.xsd" id="WebApp_ID" version="3.0"> <display-name>RESTful Services CRUD</display-name> <servlet> <servlet-name>RESTful Jersey CRUD Service</servlet-name> <servlet-class>org.glassfish.jersey.servlet.ServletContainer</servlet-class> <init-param> <param-name>jersey.config.server.provider.packages</param-name> <param-value>com.javapapers.webservices.rest.jersey</param-value> </init-param> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>RESTful Jersey CRUD Service</servlet-name> <url-pattern>/rest/*</url-pattern> </servlet-mapping> </web-app>
package com.javapapers.webservices.rest.jersey; import java.util.ArrayList; import java.util.List; import java.util.Map; public class AnimalService { AnimalDao animalDao; public AnimalService() { animalDao = AnimalDao.instance; } public void createAnimal(Animal animal) { animalDao.getAnimals().put(animal.getId(), animal); } public Animal getAnimal(String id) { return animalDao.getAnimals().get(id); } public Map<String, Animal> getAnimals() { return animalDao.getAnimals(); } public List<Animal> getAnimalAsList() { List<Animal> animalList = new ArrayList<Animal>(); animalList.addAll(animalDao.getAnimals().values()); return animalList; } public int getAnimalsCount() { return animalDao.getAnimals().size(); } public void deleteAnimal(String id) { animalDao.getAnimals().remove(id); } }
package com.javapapers.webservices.rest.jersey; import java.util.HashMap; import java.util.Map; public enum AnimalDao { instance; private Map<String, Animal> animals = new HashMap<String, Animal>(); private AnimalDao() { //pumping-in some default data Animal animal = new Animal("1", "Lion", "Wild"); animals.put("1", animal); animal = new Animal("2", "Crocodile", "Wild"); animals.put("2", animal); } public Map<String, Animal> getAnimals() { return animals; } }
Put this file createanimal.html in WebContent folder.
<!DOCTYPE html> <html> <head> <title>Create Animal</title> </head> <body> <div style="padding-left:100px;font-family: monospace;"> <h2>Create Animal</h2> <form action="rest/animals" method="POST"> <div style="width: 200px; text-align: left;"> <div style="padding:10px;"> Animal ID: <input name="id" /> </div> <div style="padding:10px;"> Animal Name: <input name="animalname" /> </div> <div style="padding:10px;"> Animal Type: <input name="animaltype" /> </div> <div style="padding:10px;text-align:center"> <input type="submit" value="Submit" /> </div> </div> </form> </div> </body> </html>
Comments are closed for "RESTful Services CRUD with Java JAX-RS Jersey".
it will be nice if you give configuration stuff with Tomee / tomee +
Can we get return type as arraylist ? can yuo give some example of it ?
how can i create and insert a value from (jax-rs)webservice into sql without using jersey
[…] a previous tutorial we saw about creating a CRUD RESTful web service using Jersey. We will create an example web service now in line with the example provided in that web service […]
Hi. Every RESTful aplication i try to create, when i run it, it shows me 404… Why the hell does this happen ?
I am unable to do delete operation ..