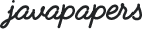
A factory method pattern is a creational pattern. It is used to instantiate an object from one among a set of classes based on a logic.
Assume that you have a set of classes which extends a common super class or interface. Now you will create a concrete class with a method which accepts one or more arguments. This method is our factory method. What it does is, based on the arguments passed factory method does logical operations and decides on which sub class to instantiate. This factory method will have the super class as its return type. So that, you can program for the interface and not for the implementation. This is all about factory method design pattern.
For a reference of how the factory method design pattern is implemented in Java, you can have a look at SAXParserFactory. It is a factory class which can be used to intantiate SAX based parsers to pares XML. The method newInstance is the factory method which instantiates the sax parsers based on some predefined logic.
Based on comments received from users, I try to keep my sample java source code as simple as possible for a novice to understand.
Base class:
package com.javapapers.sample.designpattern.factorymethod; //super class that serves as type to be instantiated for factory method pattern public interface Pet { public String speak(); }
First subclass:
package com.javapapers.sample.designpattern.factorymethod; //sub class 1 that might get instantiated by a factory method pattern public class Dog implements Pet { public String speak() { return "Bark bark..."; } }
Second subclass:
package com.javapapers.sample.designpattern.factorymethod; //sub class 2 that might get instantiated by a factory method pattern public class Duck implements Pet { public String speak() { return "Quack quack..."; } }
Factory class:
package com.javapapers.sample.designpattern.factorymethod; //Factory method pattern implementation that instantiates objects based on logic public class PetFactory { public Pet getPet(String petType) { Pet pet = null; // based on logic factory instantiates an object if ("bark".equals(petType)) pet = new Dog(); else if ("quack".equals(petType)) pet = new Duck(); return pet; } }
Using the factory method to instantiate
package com.javapapers.sample.designpattern.factorymethod; //using the factory method pattern public class SampleFactoryMethod { public static void main(String args[]){ //creating the factory PetFactory petFactory = new PetFactory(); //factory instantiates an object Pet pet = petFactory.getPet("bark"); //you don't know which object factory created System.out.println(pet.speak()); } }
Bark bark
Download Java Source Code For Factory Method Pattern
Comments are closed for "Factory Method Design Pattern".
Nice!!. Though I am using all but when i try to related to design pattern, normally it looks me like big sky infront of me.
But you explined neatly.
Thanks for your effort and time for writing this ariticle.
Thanks
Thamayanthi
Factory is one of the widely used design patterns. Happy that you are able to relate it. Good luck Thamayanthi!
Tutorials and articles available on internet try to be comprehensive and consequently confuses the reader. I try to keep my definitions as simple as possible for users to get the fundamental understanding right. So that, over experience they can build on top of it.
Design patterns are not a complex subject. With appropriate tutorials it will be interesting to learn and use.
actualy i was using this design pattern only for creating object but not for return the object of super class based on logic.
so it is now clear to use this design pattern
thanks
Best wishes Rampratap.
[…] To keep things simple you can understand it like, you have a set of ‘related’ factory method design pattern. Then you will put all those set of simple factories inside a factory pattern. So in turn you need […]
This is good article. explained in very clear understanding way.
Thanks for your post.
Thanks
Simple and Nice explanation. Able to understand the basic defenition of the Factory methods.
Nice and simplified explaination of a good design pattern..Good effort
Really good. I have understood it now. Thanks a lot
The example is good. but to make it more apparent with the definition put the public Pet getPet(String petType) in an interface and let PetFactory inplement this interface. Reason any conventional definition tell you that there are four aspects to Factory Method.
1. Product — in your case it is Pet. The interface of objects created by the factory
2. Concrete Product — in your case it is Dog and Duck .The implementing class of Product. Object of this class is created by ConcreteCreator.
3. Creator — Which is the interface i am talking about. This interface defines the factory methods
4. Concrete Creator — PetFactory in your case. This class that extends Creator and that provides an implementation for the factoryMethod. This can return any object that implements Product interface.
This will give the readers exact implementation for Factory Method.
hi Joe, thanks for the great explanation…
I have a question:
If the getPet() method in PetFactory class only determines whether to create a new Dog() or new Duck() based on String petType, then can we not make this getPet() method a static method.. and therefore in our main() method we wont have to create an instance of the PetFactory type… and instead we would be able to use the following statement
Pet pet = PetFactory.getPet(“bark”);
Which is the better way… instantiate PetFactory like you did… or don’t instantiate like pointed out in this comment… ?
Thank you for your time
Hi Joe,
Very good articles
– Senthil
very nice explanation,now I understand factory pattern.
Thanks,
Very nice way to explain. I am always feeling that design pattern are very difficult to understood.
Now you made it very simple and easy way to learn all design patterns.
Thank you very much.
Regards
Sreeni
No doubt ,its very simply explained ….But what about Abhay ‘s question? I am agree with him….
Thanks Joe…. u explained the concepts in a pristine manner…i became ur fan..
Thanks Joe…. u explained the concepts in a pristine manner…i became ur fan..
[…] If you want to provide a handle / method to deliver a copy of the current instance write a kind of factory method and provide it with a good documentation. When you are in a situation to use a third party […]
Could you please give an example about combining strategy & factory patterns ?
hi,
Please move the search button in your page to the top. It will be more user friendly.
Regards,
Balaji A
The example you have given more suits to Fatory Pattern not factory method pattern.
The exact definition for factory method pattern is to “define an interface for creating an object, but let subclasses decide which class to instantiate. Lets a class defer instantiation to subclasses”.
Joe nice explanation, but according to the definition of Factory Method ” Define an interface for creating an object, but let subclasses decide which class to instantiate. Factory Method lets a class defer instantiation to subclasses.” But here how the subclasses deciding which clas to instantiate?
Thanka Lot Joe.. you know I never seen such a simple and easy explanation to understand the factory method design pattern.. you rock it. Keep up the good work buddy…
-Lokesh
Nice Example for this design patterns
i like it
I’m a regular visitor to your site now…luv d way u explain things so tersely & easy 2 comprehend..gr8 work man…u rock !!!..
Pls keep posting more such JAVA stuff…:-)
Hi joe,
Thanks for explaining the concepts so clearly.
good and simple presentation and useful to plenty of students for references
great job
Neat And clean Explanation ,thank god u provided the download file otherwise it would b mystery for me.. Finally understood..
Thanks for your effort and time for writing this ariticle.
Nice article on Design pattern, Thanks Joe.
Thanks a lot…..i was just getting frustrated reading a hard written book
You have a great skill of presentation and making things easy
Good one! Short and crisp!!
super Joe, awesome no words to describe,I’m a new Java developer having just 1.5 years experience, luckily got here, i thought of leaving Java coz of poor understanding,now i realized that its damn easy if we understand things.
plz put the sequence diagram for this pattern
I red almost all the design patterns.It was a wonderful presentation.I am now capable of designing my classes with patterns.Thanks a lot!
Good Website Joe.
I have also read your articles on Core java, JSP and Servlets. The way you explain concepts is really good and easy to understand.
Is there any difference between Factory Design Pattern and Factory Method Design Pattern
Good one! Short and crisp!!
Hi,
Thanks for such a nice article …
Just have a doubt ….
what if the Factory method is static ?
Will it violate the definition of this design pattern ?
Very Nice and easy language.You finally achieved to make me understand the patterns.
Thanks :)
simple words, simple examples, which make complex concept clear. reading your acticle, is kind of a happy journey.
Thanks very much for sharing!!!
Really a useful article. Great work.
Keep on sharing such a useful articles.
Janakiraman
Hi Joe,
thanks to your Nice works? In some explanation and example i found the abstract class instead interface, so is there any specific reason/cause to use interface/abstract class one another ?
Good Explaination. Is there any difference between Factory Pattern and Factory method pattern?
Excellent
Hi Joe ,this is very good article i have ever read on design patterns
Great work Joe..very neat example…
Hey Joe.. I have been passionate about Design Patterns , but always get confused at some points..! Yours is a great attempt to clarify all confusions..!
Is it right that the methods of the abstract factory patterns that create the concrete product objects, are factory methods? It seems like..
very nice… well Explained.. Thanks
Waiting for a simple but an explainatory example for Singleton Design pattern also.
Thanks :)
Thanks a lot Joe.
Awesome artical , great & simple description of Factory pattern……
thanks a lot joe for putting such a nice explanation .
I have a question for you ??
Till now i knew that factory method is used to create instance of those classes which have a private constructor from other classes.
Would you like to explain the concept from this angle .
Hi Joe,
Could you please post documents on Behavioral Patterns as well.
Sure Suresh. I am planning on behavioral patterns. You will get that soon.
Thank you for such a neat example
Abhay had asked a question that, we use a static method instead of using member funtion in PetFactory class. So that it is not required to be instantiate the PetFactoy.
We all know that, static members are not related to instance scope where as its scope to class level. Static members should be loaded whenever the class loads. Joe, can you please exaplin why it is not recommonded to use a static in this scenario.
Thank you
Hi Joe,
Given example is not Factory Method Pattern. It is plain Simple Factory which just encapsulates object creation.
Hi Joe
The way you explained factory pattern is simple and understandable.You nicely related it with real life example.
Other tutorials on net had really confused me and I could have never used it as it was not clear to me.
really nice sample for factory design pattern
That’s very good article.
I have a question:
If I will add another class extend pet, do I will have to modify the factory method?
This article explains design pattern with simple examples. Great help Joe!
Excellant explanations!
So superbly simplified!! Excellent
I agree with your valid points.
Joe,
Simplicity is good.However enough information about each design patters is more important.
Great thoughts!
Factory pattern is used for addressing problem on object creation.
As you rightly mentioned, method could be static (Static Factory method). So that client side no need to create object of Factory class.
Keep it up!
Joe,
Please add section for Pre Request ( situations/conditions) and solution to the problems for each design pattern.
Your effort is appreciated.
You are right when suppose you have classes in family hierarchy that time is your idea will rightly fit.
Here, the object creation problem is in single interface and multiple implementations.
We may not required to implement completely which design pattern says…
See the problem
For Simple Problem , solution should be so simple.
For complex problem, solution should be so so simple :)
I agree Joe need to explain a lot about various problems on object creation with proposed solution.
Hope Joe may like to Super Star!
No worries!
It may not violate the definition of design pattern sir!
It will become static factory design pattern and that is really good solution when object creation for single interface with multiple implementations.
Some extend we could twist the design pattern for exactly fitting your current requirement and problems may come in future.
100 % pure gold may not help us boss!
Choosing right design pattern is more important rather than implementation (it can change as needed)
Good Question.
You may required to move to next level for understanding about Object Creation patterns what it gives solution to the object creation problems?
Just ask you
what is the problem on object creation?
Since jave provides three ways to create objects 1) new,2)Class.forname(),3) Clone !!!
then why we need to have these many patterns ? is my question valid? if yes, go ahead!
Best wishes :)
Your assumption may right at your angle
When you put constructor as private.then that class must be provide static factory method for getting object from other classes.
However Factory pattern is not only for this one exactly.
keep your thought process until for next week !!!
I agree Abhay point. its really sounds good.
Please see my reply to Abhay.
For your doubts, I could say
Static method is going to be used.
What is the need of variable (directly return statement is enough)
when we used to have variable that may required to hold for future purpose.
Factory is not going to do any logic just simply object creation and returned to client.
hope you got my explanation.
I really appreciate you since you raised questions from posted comments. that is really will help us to have good brain storming within us. Lets try to share our ideas.
That is the reason I have replied around 5 to 6 members doubt today
Always my thanks to Joe :)
If you share the UML diagrams of the example, it will be more understandable. Thanx for your study.
That was simple & precise explanation.
Very helpful.
We create object Using 3 ways…
new (Compile Time)
Class.forName (Dynamic)
Clone (Backup Object)
The purpose of Object creation is different here.
Design Pattern described here Factory Pattern, actually uses “New”
So Its Just a way to encapsulate details of creation of objects depending on Logic.
thanks. very useful! :)
I have set javapaers.com as my home page. Its really really good.
There’s an issue with definitions here. The example presented is not a “factory method” as defined in the gang of four. A factory method delegates the creation of an object to subclasses of a parent factory or interface. Each subclass creates just one type of object.
The example here is at times called a factory pattern or a simple factory. This type of pattern has one class containing branch instructions that are used to create one of a number of objects, as PetFactory does here.
Super sir….thank you very much and do keep blogging..
Great Post Sir now i understand what the factory pattern is…can u please update your blog with other factory pattern with sample code so that it will help us.
Hi Joe, Can u pls mention Why we are going to Factory Method Design pattern?
Thnx a lot…. Joe.
can u please tell me that what is the exact differences between Factory and Proxy?
Here we are creating 2 objects,
1) Instantiating Factory class.
2) Then asking for Pet object.
If we make factory method as a static method then is it a problem??
Nice explanation. Simple and easy to understand. Thank you.
thanks for your effort joe, patterns really explained with simple and easy to understanding way.
It seems simple factory pattern not the factory method pattern
AS per pic yoru factory class will also have a asuper class , but if i have read it right , your example source code does not have any such class , please help me understanding that why you choosed not to implement that ?
Awesome!
thanks, for easy example of factory design pattern. i know these thing but dont know the specific term in designed pattern.
this tutorial for all begineer,as well as specialist.
Thanks for keeping it simple and yet best. If possible try packaging all the design pattern as a pdf downloadable which should be very handy.
I have an example in my mind when I read the Factory pattern.
It’s like this,
when you go to an auto stand and ask for an auto to go to a certain place, one guy in the stand ( who normally an Auto driver) directs one of the auto drivers in the stand to take the passenger to their destination.
Here the one arranges the right auto for you is equivalent to factory. With respect to the Factory pattern context, we call him the creator who creates the the right instance (auto) and give it to you for (to travel).
Joe – Please comment this one example before it gets into others.
Everything starts with a WHY, a problem that it solves.So that and I don’t see that WHY anywhere.
A solution must solve a generic problem….here it solves the decoupling i.e creation of all composed object from object which composes it thus allowing encapsulation and this way of creation can be changed without the composing object knowing it.
Your explanation seems like FireEntinguisher does this and does that.But then
Why the **** should I Do this.Why not throw water?? or just run away when there is fire?
Everybody doesnot have fire in their houses everyday!!!
very use full
good artice on factory method. Sir can you please provide another real life example for the same
Concise and to the point .. can become an easy deference material.
Could you please also include when to use these patterns. It would be helpful for my kind of people.
Hi Joe , based on your example I can understand the pattern , do u have any other example contains complex type.
What u explained is factory pattern and wht abt factory method and when we add new instance how to get instance…..with out changing
Brilliantly explained. Thanks for the article. :)
Joe,
I dont think this is factory method, but a simple factory pattern.Can you please shed some light on this.
i think it is not for creating objects dynamically
Nice and elegant explanation!
Great stuff! I just have one feedback to say, If you could add class diagram for each design pattern that will help understanding in a better way..
Hi Joe,
Is there any difference between factory and factory method , if yes , explanation on that with an example will be really helpful.
this is just basic factory pattern not a “factory method” patterns… could you please post a correct example joe… m a fan of ur posts, but this post seems to be misguided me.
Good explanation!! Thanks
hey hi,
can u explain me factory method in simple .bcoz im not getting it
Nice article with good explanation.
Hi,
Came across your site while doing a search for sample factory method implementation in Java. But your code looks like Factory pattern and not Factory Method Pattern.
Here your factory class is making the decision on which classes instance to create. But Factory method pattern should be delegating the factory method for the inherited class to implement..!
Thanks man… Your articles are helping a lot.. Keep going…
nice article but i didn’t get it how this pattern gives high cohesion nd loose coupling.Explain me.
It is amazing, though i have studied the same concept for certification, just now realizing that, it is one of the Design Pattern …..
I have searched so many sites for Factory Method Design Pattern, simply we can not understand the way using tricky words like factory and others….
But this is simple and powerful that everyone can understand what it is a 100%….
Thank You and and thanks a lot…keep it up this way of explanation