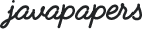
NullPointerException: An attempt was made to use a null reference in a case where an object reference was required.
NullPointerException is more famous to Java programmers, than fashion to Paris. When you start breaking the beurette and Pipette in Java lab, first thing you will get is NullPointerException. In this tutorial lets discuss about, do we need NullPointerExceptions and why does it comes and how to solve a NullPointerException (NPE). Also we need to study about null, which causes the NullPointerException.
Before going into detail, is NullPointerException named correctly? i think it should have beed name as NullReferenceException as correctly done in dotNet. Hey, I heard there are no pointers in Java atleast for namesake! then how come it is NullPointerException. You might appreciate this after reading the complete story.
Error description for NullPointerException doesn’t tells the name of the null variable, which caused the exception. The programmer, mostly beginner spends lot of time to figure out where the error is. Also it happens so frequently that you get annoyed. Error reported just says the line number at which it occurred. NullPointerException is a RuntimeException.
In a code block, if there is a possibility of null pointer exception it should be checked and reported at compile time. Yes there is language ‘Nice’ which does it and there is no chance of getting a NullPointerException.
Java API documentation says that NullPointerException can be thrown, when there is an attempt to use null where an object is required. That is you are trying to access a reference where there is no value. Main scenarios are when, calling the instance method of a null object and accessing or modifying the field of a null object. When you pass null to a method when it expects a real value. It means to say that null object is used illegally.
So how do we get a null object in place? When you create an object and not initializing it. When you access a method and it returns a null object.
It is simple, put a null check! Surround your object with if statement like
Object mayBeNullObj = getTheObjectItMayReturnNull();
if (mayBeNullObj != null) { // to avoid NullPointerException
mayBeNullObj.workOnIt();
}
If you think it is so easy and the subject is done, then you are caught wrong. I think the above method is ugly! Imagine a situation where you work using many third party components (methods), how many if conditions will you put to solve one NullPointerException? It will pollute the code. When you deal with third party components and it is supplied with poor javadoc (it always happens!), there is no other option available but to use this ugly way. If not, you can avoid this by using a better design of software. A simple example is to have proper ‘required field’ check at GUI level, when your business logic expects an operation on a given object. Then you can depend on your design and omit the if-null-check which beefs up your code.
There is a default value for all primitives in Java and it is initialized with it. Why not have something like that for objects? So that null will never exist and the whole world’s NullPointerException problem is solved. Why do we have null in Java? We need it to use in places where there is absense of information. We are depicting real world information in Java programming using OOPS. Zero is absense of information, can I use zero as a replacement for null. No! In mathematics itself zero can be used when there is absense of information, only when you are dealing with numbers. For example when you are working in real algebra, you have a null set not number zero. Of course the size of that is zero ;-) So in real world 0 and absense of existense are not same. Therefore one cannot use empty Sting “” or zero in place of null in java.
Too much techie information for the academics, you may omit this paragraph without loosing integrity of information.
Java Virtual Machine (JVM) specification gives 24 operations that throws NullPointerException. They are aaload, aastore, arraylength, athrow, baload, bastore, caload, castore, daload, dastore, faload, fastore, getfield, iaload, iastore, invokeinterface, invokespecial, laload, lastore, monitorenter, monitorexit, putfield, saload, sastore. Of those 21 would be supplemented by versions that would not throw NullPointerException. The 3 that wouldn’t be supplemented are athrow, monitorenter and monitorexit. 16 of the 21 involve loading or storing the primitive types from or in arrays; 2 involve the same with object references; 2 more: getfield & putfield; 2 for method invocation; and 1 for the length of the array.
Comments are closed for "Java null and NullPointerException".
Hi
Good article, what happens when we compare and object with null i.e
String obj = null;
if (obj == null)
{
}
How does JVM compare them.
Thanks
Simi
Can’t we handle NullPointerException by putting our code within try-catch block
i have tried my coding using try catch black but i have null pointer exception
public static void main(String args[]) {
String str = null;
try{
System.out.println(str.length());
}
catch(NullPointerException ne)
{
System.out.println(“nullpointerexception”);
}
}
}
//output : nullpointerexception
Hi, thanks for the post. Another possibility is to have a look at the Null object GoF pattern? It may be a good step to prevent from Null pointers.
there are some point to check null pointer exceptions
1# Instance level object reference (non-primitive) implicitly initialize with null so no need to initialize these references.
2# NullPonter occurs when we use variable (reference) before initialize it, So avoid calling before initialize.
3# First check the reference of the variable and then method.
for example :
ArrayList arr = new ArrayList();
/*
* business code here
*/
if(null != arrr && arr.size() > 0)
{//some code}
4# always use null in left side
it will increase your code performance.
Example:
AA aa = new AA();
if(null!= aa)
5# avoid use of size() method in collections just use isEmpty() method.
ArrayList arr = new ArrayList();
/*
* business code here
*/
if(null != arrr && !arr.isEmpty())
{//some code}
6# if you need to check multiple methods
check for each one
Example
if(null != aa && null != aa.method1() && null != aa.method1().method2())
//and so on
[…] NullPointerException, one exception that is very popular is ClassNotFoundException. At least in your beginner stage you […]
Good explanation had been provided which is very useful and curious to learn for freshers. But, it had batter if you could have explained with sample codes.
But, it’s excellent.
When we create a reference and it’s not initialized will cause NPE. But you mentioned that ‘When you create an object and not initializing it’ is misleading. correct me if I am wrong.Thank you
@Raghva
Technically you are right. Will fix it.
very bad nobody understood
such a nice thoughts of you joe , I am happy I got lot from you.It is awesome.
Hi Joe,
Is is possible to resovled null pointer exception in java using try catch block??
Hi joe
What is the difference these both if stmts and which one is best to check null….
Code:
void m1(Object obj ) {
if(null == obj)
or if(obj == null) ? Why ?
}
How to get LineNumber from NullPointerException if any one knows please post the code…
thanks
………..
what is difference between
if(null==objRef) and
if(objRef==null)
hai, u command is running but i didn’t got output
Respected Sir,
I Learn Java through your sites. i am very proud of you sir. And also i read Nageshwara Rao (Core JavaBook) this book useful for every beginner. i expect from you JSP, Servlet,Struts, Spring EJB,RMI,JavaBEans,Spring these topics cover any single book with real time example. I humble request for you publish a New Book. this my small suggestion that all. thank you sir.
[…] extends Dictionary and implements Map. Objects with non-null value can be used as a key or value. Key of the Hashtable must implement hashcode() and equals() methods. […]
how to write a test class in java
hi
I used
String src;
src=getSrc();
If(src!=null){
//code comes here
}
What is the difference between src != null and null!= src ?
Which is better ? why ?
Please reply
if (this.uwsCatalogContext == null) {
var contextObj = new Context(“UWSContext”);
theCatalog.setCatalogContext(contextObj);
this.uwsCatalogContext = contextObj;
}
in the above context i am getting null pointer exception….
getting null pointer exception in second line.
WriteToFile wt = new WriteToFile();
wt.getTableData();
may i know how to avoid it.?
String s[]={“in”,”go};
s=null;
s[1]=”ok” //line 2
why line 2 is giving NULL POINTEREXCEPTION? not ARRAY INDEXOUTOFBOUNDEXCEPTION
hi suraj
s is null when you do the operations on null .we are getting NULLPointerException
In-depth and Interesting..Its Very helpful Sir !!
yeah..we can do it by using try-catch
TRY THIS ON YOUR CODE:
try
{
———–
}
catch(NullPointerException e)
{
System.out.println(“Exception caught”+e);
}
If you want to check null for obj from many places then you can choose first one.
You can call this method from the place then based on the condition , proceed with the rest of the thing
Hi Joe sir..all of your articles are excellent.Thank you very much.
Krishna (JavaDev, Hyd)
[…] java exception based breakpoint. For example we want the program to halt and allow to debug when a NullPointerException is thrown we can add a breakpoint using […]
What is the difference between src != null and null!= src ?
Which is better ? why ?
[…] a negative number or with a number greater than or equal to its size. This stands second next to NullPointerException in java for […]
if(ca.equals(c2.getpassengerCapacity()))
{
t[i]=t2;
i++;
}