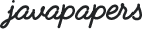
Annotation is code about the code, that is metadata about the program itself. In other words, organized data about the code, embedded within the code itself. It can be parsed by the compiler, annotation processing tools and can also be made available at run-time too.
We have basic java comments infrastructure using which we add information about the code / logic so that in future, another programmer or the same programmer can understand the code in a better way. Javadoc is an additional step over it, where we add information about the class, methods, variables in the source code. The way we need to add is organized using a syntax. Therefore, we can use a tool and parse those comments and prepare a javadoc document which can be distributed separately.
Javadoc facility gives option for understanding the code in an external way, instead of opening the code the javadoc document can be used separately. IDE benefits using this javadoc as it is able to render information about the code as we develop. Annotations were introduced in JDK 1.5
Annotations are far more powerful than java comments and javadoc comments. One main difference with annotation is it can be carried over to runtime and the other two stops with compilation level. Annotations are not only comments, it brings in new possibilities in terms of automated processing.
In java we have been passing information to compiler for long. For example take serialization, we have the keyword transient to tell that this field is not serializable. Now instead of having such keywords decorating an attribute annotations provide a generic way of adding information to class/method/field/variable. This is information is meant for programmers, automated tools, java compiler and runtime. Transient is a modifier and annotations are also a kind of modifiers.
More than passing information, we can generate code using these annotations. Take webservices where we need to adhere by the service interface contract. The skeleton can be generated using annotations automatically by a annotation parser. This avoids human errors and decreases development time as always with automation.
Frameworks like Hibernate, Spring, Axis make heavy use of annotations. When a language needs to be made popular one of the best thing to do is support development of frameworks based on the language. Annotation is a good step towards that and will help grow Java.
There are two main components in annotations. First is annotation type and the next is the annotation itself which we use in the code to add meaning. Every annotation belongs to a annotation type.
Annotation Type:
@interface{ method declaration; }
Annotation type is very similar to an interface with little difference.
Annotations itself is meta information then what is meta annotations? As you have rightly guessed, it is information about annotation. When we annotate a annotation type then it is called meta annotation. For example, we say that this annotation can be used only for methods.
@Target(ElementType.METHOD) public @interface MethodInfo { }
@Retention(RetentionPolicy.RUNTIME) public @interface Developer { String value(); }
@Target(ElementType.FIELD) public @interface FieldInfo { }
@Documented, @Inherited, @Retention and @Target are the four available meta annotations that are built-in with Java.
Apart from these meta annotations we have the following annotations.
@Override
When we want to override a method, we can use this annotation to say to the compiler we are overriding an existing method. If the compiler finds that there is no matching method found in super class then generates a warning. This is not mandatory to use @Override when we override a method. But I have seen Eclipse IDE automatically adding this @Override annotation. Though it is not mandatory, it is considered as a best practice.
@Deprecated
When we want to inform the compiler that a method is deprecated we can use this. So, when a method is annotated with @Deprecated and that method is found used in some place, then the compiler generates a warning.
@SuppressWarnings
This is like saying, “I know what I am doing, so please shut up!” We want the compiler not to raise any warnings and then we use this annotation.
We can create our own annotations and use it. We need to declare a annotation type and then use the respective annotation is java classes.
Following is an example of custom annotation, where this annotation can be used on any element by giving values. Note that I have used @Documented meta-annotation here to say that this annotation should be parsed by javadoc.
/* * Describes the team which wrote the code */ @Documented public @interface Team { int teamId(); String teamName(); String teamLead() default "[unassigned]"; String writeDate(); default "[unimplemented]"; }
... a java class ... @Team( teamId = 73, teamName = "Rambo Mambo", teamLead = "Yo Man", writeDate = "3/1/2012" ) public static void readCSV(File inputFile) { ... } ... java class continues ...
We know what a marker interface is. Marker annotations are similar to marker interfaces, yes they don’t have methods / elements.
/** * Code annotated by this team is supreme and need * not be unit tested - just for fun! */ public @interface SuperTeam { }
... a java class ... @SuperTeam public static void readCSV(File inputFile) { ... } ... java class continues ...
In the above see how this annotation is used. It will look like one of the modifiers for this method and also note that the parenthesis () from annotation type is omitted. As there are no elements for this annotation, the parenthesis can be optionally omitted.
There is a chance that an annotation can have only one element. In such a case that element should be named value.
/** * Developer */ public @interface Developer { String value(); }
... a java class ... @Developer("Popeye") public static void readCSV(File inputFile) { ... } ... java class continues ...
We can use reflection package to read annotations. It is useful when we develop tools to automate a certain process based on annotation.
Example:
package com.javapapers.annotations; import java.lang.annotation.Retention; import java.lang.annotation.RetentionPolicy; @Retention(RetentionPolicy.RUNTIME) public @interface Developer { String value(); }
package com.javapapers.annotations; public class BuildHouse { @Developer ("Alice") public void aliceMethod() { System.out.println("This method is written by Alice"); } @Developer ("Popeye") public void buildHouse() { System.out.println("This method is written by Popeye"); } }
package com.javapapers.annotations; import java.lang.annotation.Annotation; import java.lang.reflect.Method; public class TestAnnotation { public static void main(String args[]) throws SecurityException, ClassNotFoundException { for (Method method : Class.forName( "com.javapapers.annotations.BuildHouse").getMethods()) { // checks if there is annotation present of the given type Developer if (method .isAnnotationPresent(com.javapapers.annotations.Developer.class)) { try { // iterates all the annotations available in the method for (Annotation anno : method.getDeclaredAnnotations()) { System.out.println("Annotation in Method '" + method + "' : " + anno); Developer a = method.getAnnotation(Developer.class); if ("Popeye".equals(a.value())) { System.out.println("Popeye the sailor man! " + method); } } } catch (Throwable ex) { ex.printStackTrace(); } } } } }
Output:
Annotation in Method 'public void com.javapapers.annotations.BuildHouse.aliceMethod()' : @com.javapapers.annotations.Developer(value=Alice) Annotation in Method 'public void com.javapapers.annotations.BuildHouse.buildHouse()' : @com.javapapers.annotations.Developer(value=Popeye) Popeye the sailor man! public void com.javapapers.annotations.BuildHouse.buildHouse()
In previous version of JDK apt was introduced as a tool for annotation processing. Later apt was deprecated and the capabilities are included in javac compiler. javax.annotation.processing and javax.lang.model contains the api for processing.
Comments are closed for "Java Annotations".
Very well narrated Annotation in detail. Specially to mention the Runtime Reflection example tops it all. Keep going..!!
Thanks
Thaks .It is very useful for me
Nice one Joe.It is very use full to me.
Joe, you are Excellent……….
Nice article.
Joe,
It was a great surprise to me seeing ur blog. Your learning is great!
Your attitude to share it is greater!
Sharing it in a way even a layman would understand is the greatest of all!
Keep rocking. :)
Cool stuff..great refresher ..
thanks!
santosh
Nice explaination
really great!!!! keep up doing the good work for us..
Hi Joe. The things posted here are very much useful to everyone. Thanks..
Thala,
How abt including the Like button in the end of article…??
Nice post Joe!
Good example Joe…..
Fantastic information. I didnt know what was this concept, but now I am comfortable to play with it.
Thank you.
great, keep going
it’s amazing u r blog.I am first watch this blog it was good. wondonful
gr8 , thank you,
it is very help full for me…
Given inDetailed Annotation explanations. Very very Thanks.
Thank you . . ;)
Very nicely compiled article. You should write tech books :) Thanks.
Thanks……
Nice information to learn annotations
multivalue annotation is not exmplained
Thanks. It’s very helpful for me after all searching through google, finally i got good note.
Great Stuff.Thanks
Pretty good article!
Keep going, your blog is great.
Simple and Super!!
Good One Joe keep going……….
Great stuff Joe. Thanks
Good introduction on annotations exactly what I looked for.
Dear Joe, I am a newbie and I have become your fan. Whenever I google for some topics in Java and I see your picture, I go straight for your link.
You explanations are simple and straight forward. Please keep up the good work.
And thanks again!
Mast Narrated Explanation bhai
Good work. Thanks.
Hi Joe – i have read almost all your articles..u explain every concept so well.. keep it up :)
I have one very basic question for Annotation. I have googled this at so many places but not got satisfactory ans.
Just i want to know
a) WHY I NEED ANNOTATION,
b) Annotation is for JVM or for compiler or for other tools.
c)If it is for some specific tool, where i define it.
For eg i created any class for caching purpose and i made annotation for it like cacheable.. but what use of doing it.. M i really achieving anything?
My question might sounds you little too basic or dumb but would appreciate if you will answer.
Thanks in advance
Joe you have mentioned that don’t use annotations for data base related things, but in hibernate annotations are used for mapping.
Can you clarify my confusion?
Hi Joe,
Thanks for the wonderful information on annotations.
It will be really helpful, if you provide the followings
1. Advantage of annotations? How annotations helps the developers?
2. When should we go for annotations?
3. How the annotations affects the behavior of the class @ run-time? if yes, please explain.
Hi Joe, Thanks for the nice explanation about basics on Annotation.
Can you please clarify… is it possible to pass as annotation as Parameter to a method
Thanks
Purush
Hi,
Why do we go for annotations? for ex: @Inherited used for inherit the super class to derived class. that can be done through the ‘extends keyword’ right?, then what is the need of Annotaions?
Hi, is there a way to remove annotation of a class runtime ?
Thanks for that wonderful tutorial
adiply…designing
Hai
i didn’t get the clear idea about the @inherited
i want more explanation
For Example @Test class it has been inherit the properties of the class Test.How can i do that one.I need a One Example
Thanks …..
Please write a tutorial on Spring annotations..
thanks a lot
This is the best tutorial on annotations. Thanks
https://javapapers.com/spring/spring-annotation-based-controllers/
Hiiiiiii,
This is Suresh Arkati..
Than q for u r Material….
Excellent Joe, It is very useful. Thanks
Great work Joe. This was an elusive topic but your narration hugged me to the topic and made me confident. Your Great attitude to share is appreciated.
Thanks for the good tutorial
Beautiful Explanation.. Immaculate.
can i create an annotation where i can use the logger
eg:
@Logger
class Test{
LOGGER.info(“this is test method”);
}
means i want to just add @Logger above the class,it will use the respective class name and LOGGER instance.
how can i do the same
Regards
sal
@Team(
teamId = 73,
teamName = “Rambo Mambo”,
teamLead = “Yo Man”,
writeDate = “3/1/2012″
)
in this case we will see same code in generated javadoc. I mean
@Team(teamId=73,teamName=”Rambo Mambo”,…
Is anyone knows how to put only values in javadoc. For exaple
@Documented
public @interface MyAnnotation {
String description() default “none”;
}
I need to put ONLY! String value of description.
use @Test annotation
i m out of this field, bt i think sharing s so good, thanks,keep going!!!!
explanation was good…
sir i m new in industry and in which company i m there is work of springs,hibernate. can u plz tell me from where should i read these things…
Thanks in advance.
But what is the effect the Annotation can have on the method . i.e how the method is associated with Annotation.
@Documented
@Target(value={ElementType.TYPE,ElementType.METHOD})
@Retention(RetentionPolicy.RUNTIME)
@interface myAnnotation1{
public String name() default “Abhay”;
public int age() default 35;
}
class Annotated {
@myAnnotation1(age=21)
public void foo(String myParam) {
System.out.println(“This is ” + myParam);
}
What effect the @myAnnotation is having on foo() and how can we access the values of @myAnnotation in foo() method..
Your blog has been very userful
Hi Joe,
Thanks for giving glimpse on annotations :)
site design is super
The explanation on the topic here is the best as compared to other blogs. I would appreciate if you could explain “How to Parse Annotation” a bit more so that even a beginner can understand (actually i couldn’t understand what’s going on in the code).
Thanks!
Hi Joe ,
What i a trying to do is that
Step 3: Create Axis2 Web Service
I am trying to keep the server runtime and web services runtime same.
As i dont have the axis client with me right now.
I am using jboss for the same.
for this purpose i have created there are two jboss folders i.e. one is the jboss and another is copy of it.
I have given different paths for the server runtime and web server runtime.
server runtime —-path of the jboss folder
web server runtime —path of the copy of the jboss folder
Now the issue is
—>when i click on the next button
—>check the option as Generate wsdl file
—->and then again click on the next button.
the error which i am getting is system can not find the path specified.
I am still puzzled, which path the system is looking for.
Can you pls guide.
Thanks,
Abhishek J
Nice explanation!
One quick question.
What if I want to have dynamic argument value in argument
eg.
@Retention(RetentionPolicy.RUNTIME)
public @interface Developer {
String merID();
}
package com.javapapers.annotations;
public class BuildHouse {
@Developer (merID = merID)
public void aliceMethod(String merID) {
System.out.println(“This method is written by Alice”);
}
}
here I want to set that argument ‘merID’ value to be the same as value of argument I’m passing in method ‘aliceMethod(String merID)’.
I’m looking for the same thing. Any ideas?
really helpful .. great work done
by explaining so neatly .. thank U
really helpful .. great work done
by explaining so neatly .. thank U
Very nice explanation. Good Work! Tks
I believe that we can create annotations with Retention Policy SOURCE (or) CLASS.
But I could not imagine the real time scenarios. If Retention Policy is CLASS, how can I instruct compiler to do particular task?
If it’s SOURCE, can we create some applications like javadoc ?
What is most important widely used design pattern in java?
can u briefly explain? What is Observer design pattern?
Very clean explanation. Keep it up :)
Hi , Great post
Can you please tell how we can invoke methods also ,so that will get an idea how exactly JUnit ,TestNG
If I am not wrong these tools work on Reflection and Annotation ?
Great discussion. Very Helpful. Thank You
anyone help me to know how to define values while creating annotation and how to call and compare that values in annotation
Very Nice article… Helpful to understand Annotations.
very well organised tutorial, thanks
Replace ‘Annotation Types’ with ‘Meta Annotation Types’ in the heading under the ‘Meta Annotation’ section. Very well written nevertheless. Keep up the good work ! Appreciate it !
Hi Joe,
All your articles are easy to understand. Thanks for such a explanation. I suggest you to describe further in detail where ever required.
My question to you is:
step 1) I have created Customize annotation
public @interface ExecuteBeforeMethod{
//Some attributes over here
String methodName();
String className();
}
step 2) Creat Class where i use above annotation
Class Test{
@ExecuteBeforeMethod(className=”xyz”, methodName=”abc”)
public method(){
//Some logic over here
}
}
But how does JVM comes to know at runtime to call method which i mentioned in my annotation? or How does dynamic binding of annotation and for method to which i written annotation is related to?
Parsing i know and you written it in main() method in your example.
But for my application how do i do?
When my code call “method()” before that how method which i mentioned in annotation is called? or How and where parser is linked in this case?
Interesting.
Nice Stuff
Good one :)
Good Stuff …………
Superb explanation….thanks
‘If the compiler finds that there is no matching method found in super class then generates a warning.’
Please verify the above line under @Override.
I think it creates compilation error…
Anyways thanks for writing the blog.It was helpful.
Godavari.M
Its really good, after red this i impressed a lot, Thank you Joe for this Tremendous effort. Keep it up
Rambo Mambo was good.
Dear Joe,
First of all thanks for the article.It’s really very useful. However i have one query. Are you sure that we can’t use parameters in methods?
Because i have seen methods like following
public String Joe(@RequestParam(“name”) String name, Model model)
{
String message = “Hi” + name + “!”;
model.addAttribute(“message”, message);
return “Joe” ;
}
Hi Joe,
First of all thanks a lot for presenting annotations in detail. It’s quite helpful
But one thing I am very much eager to know is,
Will it cause any issue if the annotations got compiled with one version of jdk (say jdk1.5) and the classes that use these annotations are complied with a different version of jdk (say jdk1.7) ?
Thanks!!
Very good presentation, go ahead….
Thanks for Annotation KISS.(Keep it simple sir)
Very informative, simple and easy to understand tutorial.. great work.. Keep it up joe..
I enjoyed this reading . Thank you.
Welcome Piyush.
Thankyou Joe
Please let me know,if you come across such thing .
Keep up the good work!
good and clear explanation… Would be great if Joe can answer the questions posted by viewers on the comments. I know it needs moretime, but found many unanswered.
Such a wounderful articale
How can i get the field name while creating our own annotation?
This is very clear and good presentative.somany things i got it aboput annotaion.
Sir, We want annotation list .
Sir,
Please can you provide the annotation list on this email id..
Thanking you
Siddique
[…] Configuration for dependency are done using Spring-configuration XML or using annotations. Annotations are mostly preferred for their ease of use. Spring manages these objects using BeanFactory. When an […]
which technologies are used for building java papers
which of the following keyword is used to create an user defined annotation?
a)class
b)enum
c)interface
d)NOne of the above
which of the following is used only in the case of Inheritance?
a) @Depricated
b)@Supresswarnigs
c)@Override
great work joe ,specially parsing the annotation
what is usages in real time senariao of annations