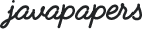
Recently I have been writing introductory tutorials about web services in Java. We saw about SOAP based hello world web services and how to create them using Eclipse and Netbeans. Now this tutorial is about getting started with Axis2 based webservice using Eclipse.
There are different web service implementation engines available for Java. Axis2 from Apache is the popular web service stack. In this hello world tutorial, we will use Axis2 for implementing a simple web service using Eclipse wizards. Eclipse does not come with pre-configure Axis2 setup. So the key to creating a web service using Eclipse for Axis2 is setting up the Eclipse.
See when we are lazy and want all the job to be done by an IDE, we should have the right setup and configuration. To create a RESTful webservice with Axis 2 using Eclipse wizard ensure the following.
If you are in a hurry, just download Axis2 Web Service and Client project and execute it. You should add Axis2 jars in lib folder.
We all know how to create a dynamic web project for Java in Eclipse as it is what we do daily in our office.
To create a web service using Axis2 we should add a step. Just do not go with the default runtime (server) configuration. We need to add Axis2 Web Services in Project Facets. Click Modify button for server runtime configuration while creating the dynamic web project and select the Axis2 Web Services Project Facet.
Then click next, next, next … done. A brand new Java dynamic web project is created with runtime support for Axis2 web services.
It is going to be simpler than expected. We will use the bottom up style for web service creation. First we will create the Java class and generate the WSDL out of it. Create a new Java class with a method that will serve as the heart of web service.
package com.javapapers.webservice; public class Axis2HelloWorld { public String helloWorld() { return "Hello World"; } }
Just the wizard job is remaining. Now use the create new web service wizard. Choose the service class that was created in the previous step and click next to finish. If you choose the web service client generation, then we will get a separate web application generated by Eclipse. Thats it, we have created a hello world Axis2 web service and client using Eclipse.
Download Axis2 Web Service and Client project. You should add Axis2 jars in lib folder.
Comments are closed for "Axis2 Web Service using Eclipse".
nice tutorial joe..
Hi Joe,
What i thought was, axis is one of the possible ways to implement SOAP based (XML request & Response) communication between the client and the webserver. Also in this case , There could be a stub client generated from the WSDL. Please correct me if i am wrong.
Thanks,
Arun
Nice Article…
Thanks for posts like this. Useful
If you really want to understand soap web services, do try using JAX-WS. Using axis and Eclipse hides important points required for developing real world applications
good job joe
Hi,
Very nice article.
cheers
Hi Joe,
Been a long time follower of your site. Thanks so much for all the articles.
I liked this one. I am beginner in Webservice and am not sure how to test this webservice. Can you add a small note on the same?
It would be very useful
Thanks!
Gayathri
Respected Sir,
I use Tomcat server 5.5 version i have installed but i cant able work and run in any program. i use google chrome browser. how to run a jsp program? and also any setting to set class path, login path,startup path… please tell me sir immediately sir
i guess u can use SoapUI for testing
Yes sure and thats a nice tool.
I get an error “Selection must be wsdl” while doing the last step.
Joe can you also explain what is going behind the scenes. that would really help or provide some links which helps us in understanding this.
Thanks for the tutorial.
when i send request using ajax to tomcat, it does no invoke the servlet for request processing it gives the previous response(i use the same uri for first request and for second request)Plz healp me.
Can we send an array list or collection through web services?
I’ve to send a large amount of data. How it is possible to me?
this is very good java tutorial for all the learners joy which is in easy understandable language joy
I wanted to know about the code generation of web service ?
There are
adb,axios,xmlbeans and other methods are available to generate code wsdl2java which one is good any bug free ?
Advantage and disadvantage of each one ?
and This can be done by eclipse plug-in and Axis2 wsdl2java.bat file as well ? wht is deffrnt between these code generation ?
Thanks and Regards,
Vishal Bhalani
how to hide the attribute of the web service..while the generation…
thanks in advance
First, you can set Axis2 as default for Eclipse
Secondly, you don’t need to set the Facet manually, Eclipse will do that for you. Just start with a Dynamic Web Application.
Thirdly, there are hard dependencies between Axis2 versions and Eclipse versions. So if you have problems, it might be because you have the wrong versions.
Fourth, if you mail me I will give you the address to a great Greece site which demonstrate this.
Hi Joe,
First of all, thank you for your post. My problem is this, I’m working on a Tomcat v7, which have a Dynamic Web Module version 3.0 .Then in Project Facets appears that the Axis2 version is 1.1 (when I installed correctly the Axis2 version 1.6) and says that required a Dynamic Web Module “2.2,2.3,2.4,2.5”.
I found one solution in a website which says to change somethings in one file (org.eclipse.wst.common.project.facet.core.xml ), but I would like to know if there’s another solution.
Why appear my Axis2 version like 1.1 when actually I installed the version 1.6?
Why is not compatible with Dynamic Web Module Version 3.0?
Thank you for your time, and sorry for my english (I’m from Spain)
Sorry, Joe
I forget to tell you, that when I tried to change the Dynamic Web Module to 2.5, Eclipse dont let me do it, appears a message which says: Cannot change version of project facet Dynamic Web Module to 2.5
Hello can u help me to implement the security issue in web service
HI Joe,
Thanks for these kind of nice article.
I want to validate user input using axis2.
Can you provide to me for same
Hi. Thanks for the tutotrial. I have the next error when i click in finish button in the web service wizard:
IWAB0489E Error when deploying Web service to Axis runtime
axis-admin failed with {http://schemas.xmlsoap.org/soap/envelope/}Client The service cannot be found for the endpoint reference (EPR) http://localhost:8080/Axis2WebService/services/AdminService
Hi,
Every nice this article , Can you please provide the Axis2 Security implementation and HTTPS also
Me too got the same problem.Did you get any answer?If so can you please help me also.
hi joi
thanks alot
I coudn’t understand the point
“If you are in a hurry, just download Axis2 Web Service and Client project and execute it. You should add Axis2 jars in lib folder.”
And i have downloaded and updated axis2 preferences runtime with the extracted folder location, even then facets are not showing up when we create webservice project. When i tried to add axis2 from properties of project, i am getting a error as below “failed while installing Axis2 Web Services”.
Please help me on this, i am running Eclipse Version: Juno Service Release 2
Build id: 20130225-0426
and Axis2 1.2
Thanks in advance
Dhanush M
Brief, simple, easy and understandable explanation. Very nice
thank you joe is this very much useful to me to learn axis2
I have different operation name but same response object for 2 WSDL operations. Eclipse throws an error. Is there any way that I could overcome this kind of error?
I am getting error while i try to do apache axis2 webservices.
Exception java.lang.ClassCastException: org.apache.cxf.calculator.CalculatorServiceSoapBindingStub cannot be cast to javax.xml.ws.BindingProvider.
Kindly provide me the solution JOE
yours friendly Vijay
Hey Joe! Its really a nice job done by you. I have a question here. What if our web service class depends on some other class in some other package for its implementation. How do we publish it on Tomcat then
I am trying a sample web service program for adding two numbers..
The server program is:
———————
package mypack;
public class AddProg {
public int add(int a,int b)
{
return a+b;
}
public static void main(String[] args) {
// TODO Auto-generated method stub
AddProg ap=new AddProg();
ap.add(3, 5);
//System.out.println(x);
}
}
The Client program is:
———————-
package mypack;
import java.rmi.RemoteException;
import org.apache.axis2.AxisFault;
import mypack.AddProgStub.AddResponse;
import mypack.AddProgStub.Add;;
public class AddClient {
public static void main(String[] args) throws RemoteException, AxisFault {
AddProgStub stub=new AddProgStub();
Add r=new Add();
r.getA();//getting data
r.getB();
AddResponse res=stub.add(r);
System.out.println(res.get_return());
}
}
The problem is I can set the data like
r.setA();//setting data
r.setB();
and I am getting the output of addition of two numbers..
But while I am gettin the data like
r.getA();//getting data
r.getB();
I am getting the following error..
Error:
——
Exception in thread “main” org.apache.axis2.AxisFault: unknown
at org.apache.axis2.util.Utils.getInboundFaultFromMessageContext(Utils.java:536)
at org.apache.axis2.description.OutInAxisOperationClient.handleResponse(OutInAxisOperation.java:375)
at org.apache.axis2.description.OutInAxisOperationClient.send(OutInAxisOperation.java:421)
at org.apache.axis2.description.OutInAxisOperationClient.executeImpl(OutInAxisOperation.java:229)
at org.apache.axis2.client.OperationClient.execute(OperationClient.java:165)
at mypack.AddProgStub.add(AddProgStub.java:204)
at mypack.AddClient.main(AddClient.java:18)
———————————————–
please help me why am having this error.. i tried more but i can’t come out with the answer..
Hi joe,
if i want to create web service using Axis2 and in tht i used some jsp and servlet. in jsp i am giving some input which i wanted in servlet and printing it on console.this simple thing is not working in it but same thing when i am doing in simple dynamic project without including axis2 it is working.
i want to know reason why its not working thr?
Hi joe,
if i want to create web service using Axis2 and in tht i used some jsp and servlet. in jsp i am giving some input which i wanted in servlet and printing it on console.this simple thing is not working in it but same thing when i am doing in simple dynamic project without including axis2 it is working.
i want to know reason why its not working thr?
I am also getting the same error, but I am not using the CXF web service runtime, I am using the Axis2 Web Service runtime. Any idea/suggestion?
Sir,
I have created a dynamic project and created a web service client from a wsdl. But after that i cannot create the client. Can you help me sir ?
Hi sir,
I have created a two Service classes , when i set the value in one service class and trying to get the value in the another service class for a single session , i got value as null in the another service class .may i know how to get that value in the another service class.
Hi sir
I have created a webservice(producer) by using axis1.x in my local system. I want to move this code in other server(Development Server).For it I need any cange in code(webervice).Please give me reply .
Hi ,
I have created my web service from WSDL2java axis2 code generator and got deployed in tomcat + ecplise. But i noticed there is difference between the WSDL file which has been published in the URL and the the WSDL which has been used for code generation.
Please let me know how can we get the same WSDL as used for code generation.