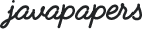
Spring is the most popular open source java application framework as of current moment. It is one of the best job enablers too (at least in Indian software services companies).
Before starting on spring framework, I recommend you to become strong in core-java first, then remember to study servlets, jsp and then start on Spring framework.
Spring framework provides multiple modules wherein MVC and Inversion of Control container are popular among them. In this article I will present a spring MVC based Hello World web application.
I am using Spring version 3 latest available now. SpringSource provides a tool suite (STS) IDE which is based on Eclipse to develop Spring based applications. But, my personal choice for now is to continue with Eclipse JEE IDE.
Model view controller is a software architecture design pattern. It provides solution to layer an application by separating three concerns business, presentation and control flow. Model contains business logic, controller takes care of the interaction between view and model. Controller gets input from view and coverts it in preferable format for the model and passes to it. Then gets the response and forwards to view. View contains the presentation part of the application.
Spring MVC is a module for enabling us to implement the MVC pattern. Following image shows the Spring’s MVC architecture
This class is a front controller that acts as central dispatcher for HTTP web requests. Based on the handler mappings we provide in spring configuration, it routes control from view to other controllers and gets processed result back and routes it back to view.
Software versions used to run the sample code:
Use Java EE perspective and create a new dynamic web project. Let us start with web.xml
In web.xml primarily we are doing servlet mapping to give configuration for DispatcherServlet to load-on-startup and defining spring configuration path.
<?xml version="1.0" encoding="UTF-8"?> <web-app xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://java.sun.com/xml/ns/javaee" xmlns:web="http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" xsi:schemaLocation="http://java.sun.com/xml/ns/javaee http://java.sun.com/xml/ns/javaee/web-app_2_5.xsd" id="WebApp_ID" version="2.5"> <display-name>Spring Hello World</display-name> <welcome-file-list> <welcome-file>/</welcome-file> </welcome-file-list> <servlet> <servlet-name>springDispatcher</servlet-name> <servlet-class> org.springframework.web.servlet.DispatcherServlet </servlet-class> <init-param> <param-name>contextConfigLocation</param-name> <param-value>/WEB-INF/config/spring-context.xml</param-value> </init-param> <load-on-startup>1</load-on-startup> </servlet> <servlet-mapping> <servlet-name>springDispatcher</servlet-name> <url-pattern>/</url-pattern> </servlet-mapping> </web-app>
This spring configuration file provides context information to spring container. In our case,
The tag mvc:annotation-driven says that we are using annotation based configurations. context:component-scan says that the annotated components like Controller, Service are to be scanned automatically by Spring container starting from the given package.
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns="http://www.springframework.org/schema/beans" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns:mvc="http://www.springframework.org/schema/mvc" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation=" http://www.springframework.org/schema/mvc http://www.springframework.org/schema/mvc/spring-mvc-3.0.xsd http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans-3.0.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context-3.0.xsd"> <context:component-scan base-package="com.javapapers.spring.mvc" /> <mvc:annotation-driven /> <bean class="org.springframework.web.servlet.view.InternalResourceViewResolver"> <property name="prefix" value="/WEB-INF/view/" /> <property name="suffix" value=".jsp" /> </bean> </beans>
These jars are part of standard spring framework download except the jstl.
Following controller class has got methods. First one hello maps to the url ‘/’. Annotation has made our job easier by just declaring the annotation we order Spring container to invoke this method whenever this url is called. return “hello” means this is used for selecting the view. In spring configuration we have declared InternalResourceViewResolver and have given a prefix and suffix. Based on this, the prefix and suffix is added to the returned word “hello” thus making it as “/WEB-INF/view/hello.jsp”. So on invoking of “/” the control gets forwarded to the hello.jsp. In hello.jsp we just print “Hello World”.
org.springframework.web.servlet.view.InternalResourceViewResolver
Added to printing Hello World I wanted to give you a small bonus with this sample. Just get an input from user and send a response back based on it. The second method “hi” serves that purpose in controller. It gets the user input and concatenates “Hi” to it and sets the value in model object. ‘model’ is a special hashmap passed by spring container while invoking this method. When we set a key-value pair, it becomes available in the view. We can use the key to get the value and display it in the view which is our hi.jsp. As explained above InternalResourceViewResolver is used to resole the view.
package com.javapapers.spring.mvc; import org.springframework.stereotype.Controller; import org.springframework.ui.Model; import org.springframework.web.bind.annotation.RequestMapping; import org.springframework.web.bind.annotation.RequestMethod; import org.springframework.web.bind.annotation.RequestParam; @Controller public class HelloWorldController { @RequestMapping("/") public String hello() { return "hello"; } @RequestMapping(value = "/hi", method = RequestMethod.GET) public String hi(@RequestParam("name") String name, Model model) { String message = "Hi " + name + "!"; model.addAttribute("message", message); return "hi"; } }
<html> <head> <title>Home</title> </head> <body> <h1>Hello World!</h1> <hr/> <form action="hi"> Name: <input type="text" name="name"> <input type="submit" value="Submit"> </form> </body> </html>
Use key “message” in model to get the value and print it.
<%@ taglib uri="http://java.sun.com/jsp/jstl/core" prefix="c" %> <%@ page session="false" %> <html> <head> <title>Result</title> </head> <body> <h1><c:out value="${message}"></c:out></h1> </body> </html>
Download SpringMVC Sample Code and Run it!
Voila! I have started on Spring!
Comments are closed for "Spring MVC Hello World".
Hi Joe…
Thank.
Simple and great document.
hey joy.. you always writes awesome post.. i really love to read them.
But why you jumped directly on Spring MVC. you should first start Spring Basics, DI, AOP and other topics.
I wish you will do it soon. I am really eagar to read those topics on Javapapers.com as i know you will again write something good on those topics.
Hello Joe,
Nice to learn Spring framework from your blog, it is easy and more understandable.
Thank you so much Joe and one more thing you told to learn servlet and jsp before starting Spring MVC, Can you please tell me what are the concepts should be known in Servlet and jsp.
Hey Joe….It really help me a lot to understand the flow…and you explain it in very simply way….I have one doubt on Spring Tiles…So if it is possible then can you post the tutorial of Spring Tiles with your explanation(that is your touch)….
Thanks a lot
Hi Joe!
Thanks for initiating with Spring framework, really a useful article to head-start with Spring MVC.
It would be great if you help us with the Spring Expression Language instead of JSTL.
—
Sandeep
Thanks for nice article..! Keep going..:)
spring can be annotation based and xml based.
Was actually hoping you would write on this exact same topic. I looked around on the internet. I couldn’t find anything so simply explained.
Thanks a lot. U r my hero.
very nice explanation and example.
thanks.
Hi Joe,
it was a nice article. but i am somewhat confused about the Model. as you in first section you said that it contain business logic and afterwards you said that it will have data to used on the view layer.
which is corret.
Thanks you are doing very good job. :)
– Mayur Kumar
use full explanation.
Very good explanation ,It is best for beginners..Thanks alot
Its a awesome article I must say.Thank you very much.But would you please share more on Spring framework. Specially, please include IOC. Please please please. It will be very helpful to us. Thak you again.
Hi Joe,
I am trying to run the application but still facing some issues.
I don’t see any errors in tomcat logs, server start up perfectely but still webpage is saying that Resoruce is not available.
Please help me where i might be going wrong.
Thanks
Shailesh
Sailesh, check your jsp page path
Hi Joe!
I am working in spring application but I dont have this much of understanding regarding controller. Now i got it. Thanks!!!
Shailesh,
have you added all the required jars?
Hi Joe!
If we are familiar with struts framework can we work on springs or anything else to be known in advance.
Is there any similarities between struts and spring frameworks
Thanks Joe
IAM GETTING THE FOLLOWING EXCEPTION…
exception
javax.servlet.ServletException: Servlet.init() for servlet springDispatcher threw exception
org.apache.catalina.valves.ErrorReportValve.invoke(ErrorReportValve.java:102)
org.apache.catalina.connector.CoyoteAdapter.service(CoyoteAdapter.java:298)
org.apache.coyote.http11.Http11Processor.process(Http11Processor.java:857)
org.apache.coyote.http11.Http11Protocol$Http11ConnectionHandler.process(Http11Protocol.java:588)
org.apache.tomcat.util.net.JIoEndpoint$Worker.run(JIoEndpoint.java:489)
java.lang.Thread.run(Unknown Source)
root cause
java.lang.IllegalArgumentException: Method must not be null
org.springframework.util.Assert.notNull(Assert.java:112)
org.springframework.core.BridgeMethodResolver.findBridgedMethod(BridgeMethodResolver.java:65)
org.springframework.beans.GenericTypeAwarePropertyDescriptor.(GenericTypeAwarePropertyDescriptor.java:67)
org.springframework.beans.CachedIntrospectionResults.(CachedIntrospectionResults.java:251)
org.springframework.beans.CachedIntrospectionResults.forClass(CachedIntrospectionResults.java:145)
org.springframework.beans.BeanWrapperImpl.getCachedIntrospectionResults(BeanWrapperImpl.java:296)
org.springframework.beans.BeanWrapperImpl.setPropertyValue(BeanWrapperImpl.java:1003)
org.springframework.beans.BeanWrapperImpl.setPropertyValue(BeanWrapperImpl.java:857)
org.springframework.beans.AbstractPropertyAccessor.setPropertyValues(AbstractPropertyAccessor.java:76)
org.springframework.beans.AbstractPropertyAccessor.setPropertyValues(AbstractPropertyAccessor.java:62)
org.springframework.web.servlet.HttpServletBean.init(HttpServletBean.java:119)
javax.servlet.GenericServlet.init(GenericServlet.java:212)
org.apache.catalina.valves.ErrorReportValve.invoke(ErrorReportValve.java:102)
org.apache.catalina.connector.CoyoteAdapter.service(CoyoteAdapter.java:298)
org.apache.coyote.http11.Http11Processor.process(Http11Processor.java:857)
org.apache.coyote.http11.Http11Protocol$Http11ConnectionHandler.process(Http11Protocol.java:588)
org.apache.tomcat.util.net.JIoEndpoint$Worker.run(JIoEndpoint.java:489)
java.lang.Thread.run(Unknown Source)
PLEASE HELP ME..
[…] week i started my first tutorial on Spring MVC and got good reception from you. Thanks and lot more is coming on Spring! PREV POST: Spring […]
Hi Jeo,
Thank u so much for starting Springs MVC, as i am very interested to learn Springs, please provide a full flow and project Hierarchic so that we(Readers) can develop a simple application on springs MVC through your blog.
Hi Jeo….
Thank you so much to introduce about Spring MVC please also some libraries link to download it…….
Thanks for sharing the valuable knowledge with us on spring. It is easy to understand for a beginner.
Hi Joe!
Thank You for sharing your knowledge, please provide some articles on JSF.
Awesome tutorial on MVC, you can find the basics tutorials of spring except MVC in java4s.com
Getting this error, when i tried to run the program:
org.springframework.web.servlet.DispatcherServlet noHandlerFound
WARNING: No mapping found for HTTP request with URI [/SpringMVC/] in DispatcherServlet with name ‘springDispatcher’
I am new to Spring MVC, please help me out
The easiest tutorial on Spring…I ever saw!
good job dude. BLESS
[…] this implementation. We have seen about these controller annotations at high level in a previous tutorial on spring MVC. I will be using the same code available in that tutorial to explain the […]
Hi, I am looking for good and simple example to learn spring, Here is the answer….
Nice post.
Any one can give link of org.springframework.asm-3.1.2.RELEASE.jar
hi joe, i am your regular follower.i have just started to learn spring framework.and i m facing many difficulties.please post some tutorials about spring framework for begginer like me.It would be very helpful for us.
hi,
Your way of explanation is very helpful for
freshers.your pasion is great. thanks a lot……….
krishna chaitanya
Hi Joe,
Good one
thanks…
dear sir, am a regular follower of ur articles and it helps me a lot. Now, I want to learn spring framework and ur this article only my start up. Thanks a lot! Kindly suggest me some good books (or web references) to dig more about spring just as a beginner pls..
Hi Joe,
Your articles are wonderful. Looking for articles on Hibernate , Spring , EJB from you soon.
Keep up the good work :)
HI Joe,
In my jsp there are 15 fields are there,
in this case how capture these fields in servered. like
@RequestMapping(value = “/login”, method = RequestMethod.GET)
public String login(@RequestParam(“name”) String name, ModelMap model) {
model.addAttribute(“message”, “Spring 3 MVC Hello World” + runtimeService.toString());
return “login”;
}
But i don’t want to use @RequestParam(“name”). Is there any other option to bind as bean Object. like Login object.
hope you got what i’am excepting.
thxs in advanced.
Hi joe i want a spring jdbc web application that is to insert some bulk records into mysql database can u explain me the example
good job joy…thank so much for giving this example…its really helpfull for a beginer
thanks a lot for your great tuto
Are there a way to use it with maven?
Do you have a recomandation to configure a pom.xml
Unfortunately, i tested to makes it work but i’ve this error:
org.springframework.web.servlet.DispatcherServlet noHandlerFound
WARNING: No mapping found for HTTP request with URI [/helloWorldSpring/hi] in DispatcherServlet with name ‘springDispatcher’
if any one have spring web service pls send the whole code
hi i am shivani and i m very confused in return type in handler methods plz describe each return type with its use abd example,,,
I only know about the names of return type and before the method name we have to mention it,besides this i don’t know anything that how exactly a controller decides to choose one type from all of them.
Return type:::
1.ModelAndView
2.String
3.View
4.A Model object
return new Products(1245);
5.void
6.A specific string:
“redirect:/products”
“forward:/products”
Hi Joe, I tried your sample its nice. But when I passed the form name “hi” in JSP as below is not passed to servlet it passing to default @RequestMapping(“/”) in servlet:
There is no mapping controller method for the url springDispatcher.htm
Simply nice Document
Nice tutorial. Can you Please give some tutorial on java’s role in cloud technologies.
Thats a nice idea. Will write on it soon.
Hi Joe,
Its Easy to learn Spring framework from your blog,its good for beginners,and pls provide more examples in spring (in every sites they are providing only Hello World example ).So can u please provide some complex exp it will be more useful for beginners to work in it and understand deeply about spring.
Thanks
Midhun
Mail Id:mids1989@gmail.com
Hi Joe
Thanks for this tutorial. it’s really helps me a lot.
Great work…proceed on…
hi
i am new to spring mvc
in my project i need to display a child (jsp) from parent(jsp) with a dynamic list of data
can you please help in this
Thanks for this tutorial
Hi Joe
Your site is really superb .
The first thing i open in my system is javapapers.com.
thanks joe.
I would like to know how spring mvc is more better than struts2.I know abt structs 2 but very little abt spring
Hello Sir,
I tried to run given demo application (SpringMVC) but it showing HTTP Status 404 – /SpringMVC/
Additionally there is the red(i.e error) mark at first line of hi.jsp
Please suggest……
Hello,
I also had a error on the first line of hi.jsp, telling me ‘Can not find the tag library descriptor’.
i found the solution that helped me: install another jar jstl-1.2.jar in your WEB-INF/lib folder, clean up the project and the error disappears.
Good luck, Mike
Thanks a lot!!!!!!!!!!!
Its very helpful and running successful, awesome.
Thank you very much!
Hi Joe!
Thanks a ton for such a wonderful explanation on Spring MVC. Its very Crisp and Simplified.
Bless you. :)
i’m using jboss server so what changes should i make in downloading jar file
Hi I am getting the same error please let me know what was the solution
I am trying to integrate spring with seam framework, can anybody help me with configuration for integrating.
i can able to create spring service separately, i need help to integrate spring with seam.
Thanks in advance
Balaji
Hey Joe….I new to your blog…
the way you are explaining things and providing the example for same is amazing and very easy to get it….Its really nice material…
Thanks and keep it up with good job…
Now onwards i will be regular on it…
Cheers
:)
Thnx…!!
really good tutorial
For a purchased domain name, where/how would I configure it to post to it rather than …localhost:8080… ?
Superb Explanations !
Looking for Spring MVC with Spring Security
Thanks Joe
Nicely drafted… great work. It really helped me alot :)
Hi…. I am a new joinee to this blog.
I tried with this demo MVC app but getting below error.
javax.servlet.ServletException: Servlet.init() for servlet HelloWeb threw exception
com.springsource.insight.collection.tcserver.request.HttpRequestOperationCollectionValve.traceNextValve(HttpRequestOperationCollectionValve.java:90)
com.springsource.insight.collection.tcserver.request.HttpRequestOperationCollectionValve.invoke(HttpRequestOperationCollectionValve.java:76)
org.apache.catalina.valves.ErrorReportValve.invoke(ErrorReportValve.java:102)
org.apache.catalina.connector.CoyoteAdapter.service(CoyoteAdapter.java:298)
org.apache.coyote.http11.Http11Processor.process(Http11Processor.java:857)
org.apache.coyote.http11.Http11Protocol$Http11ConnectionHandler.process(Http11Protocol.java:588)
org.apache.tomcat.util.net.JIoEndpoint$SocketProcessor.run(JIoEndpoint.java:409)
java.util.concurrent.ThreadPoolExecutor$Worker.runTask(ThreadPoolExecutor.java:886)
java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:908)
java.lang.Thread.run(Thread.java:662)
root cause
java.lang.NoSuchMethodError: org.springframework.web.context.ConfigurableWebApplicationContext.setId(Ljava/lang/String;)V
org.springframework.web.servlet.FrameworkServlet.createWebApplicationContext(FrameworkServlet.java:430)
org.springframework.web.servlet.FrameworkServlet.createWebApplicationContext(FrameworkServlet.java:458)
org.springframework.web.servlet.FrameworkServlet.initWebApplicationContext(FrameworkServlet.java:339)
org.springframework.web.servlet.FrameworkServlet.initServletBean(FrameworkServlet.java:306)
org.springframework.web.servlet.HttpServletBean.init(HttpServletBean.java:127)
javax.servlet.GenericServlet.init(GenericServlet.java:212)
com.springsource.insight.collection.tcserver.request.HttpRequestOperationCollectionValve.traceNextValve(HttpRequestOperationCollectionValve.java:90)
com.springsource.insight.collection.tcserver.request.HttpRequestOperationCollectionValve.invoke(HttpRequestOperationCollectionValve.java:76)
org.apache.catalina.valves.ErrorReportValve.invoke(ErrorReportValve.java:102)
org.apache.catalina.connector.CoyoteAdapter.service(CoyoteAdapter.java:298)
org.apache.coyote.http11.Http11Processor.process(Http11Processor.java:857)
org.apache.coyote.http11.Http11Protocol$Http11ConnectionHandler.process(Http11Protocol.java:588)
org.apache.tomcat.util.net.JIoEndpoint$SocketProcessor.run(JIoEndpoint.java:409)
java.util.concurrent.ThreadPoolExecutor$Worker.runTask(ThreadPoolExecutor.java:886)
java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:908)
java.lang.Thread.run(Thread.java:662)
Kindly help….
Thanks.
supperrrvvv
y r right Amila this tutorial is so good…
I am getting this error in hi.jsp
Can not find the tag library descriptor for “http://java.sun.com/jsp/jstl/core”
i have the jar in lib folder for jstl..still it happens
any clue ??
works fine, except a small change…
hi as hello
&
hello as hi (return param)
in that java file!
Thnx joe ;)
download jstl.jar file and put it in ur lib folder.
goto ‘configure build path’ add ‘external jar’ and add jstl.jar
really it is very helpful ,
thank u joe
hi rally thnx a lot………its too good and clear in concepts.
hi joe,
i am getting the error that i have shown u below , please help me to solve the error.
HTTP Status 404 – /SpringMVC/
type Status report
message /SpringMVC/
description The requested resource (/SpringMVC/) is not available.
Superb example joe, thanks a lot…. keep it up.
Can u post Spring step be step project creation in NetBeans IDE
Keep going !!!
Great work
I don’t know how so many people got this to work. I’ve been fighting with it for over three hours – time to give up an move on.
I created everything exactly as the instructions indicate, copying and pasting the code. But Tomcat can’t find the org.springframework.web.servlet.DispatcherServlet. The dependencies noted above for the Spring web.servlet – well it does not exist. The DispatcherServlet is in the webmvc module. I included that, but it still can’t find it.
Hello joe,
I am getting the following error, any kind of help to sort it out is appreciated.
Sep 04, 2013 4:55:15 PM org.apache.catalina.loader.WebappClassLoader modified
SEVERE: Resource ‘/WEB-INF/lib/antlr-2.7.6.jar’ is missing
Sep 04, 2013 4:55:15 PM org.apache.catalina.core.StandardContext reload
INFO: Reloading Context with name [/HelloWorld] has started
Sep 04, 2013 4:55:15 PM org.apache.catalina.core.ApplicationContext log
INFO: Destroying Spring FrameworkServlet ‘springDispatcher’
Sep 04, 2013 4:55:15 PM org.apache.catalina.core.ApplicationContext log
SEVERE: Servlet springDispatcher threw unload() exception
javax.servlet.ServletException: Servlet.destroy() for servlet springDispatcher threw exception
at org.apache.catalina.core.StandardWrapper.unload(StandardWrapper.java:1485)
at org.apache.catalina.core.StandardWrapper.stopInternal(StandardWrapper.java:1822)
at org.apache.catalina.util.LifecycleBase.stop(LifecycleBase.java:232)
at org.apache.catalina.core.StandardContext.stopInternal(StandardContext.java:5463)
at org.apache.catalina.util.LifecycleBase.stop(LifecycleBase.java:232)
at org.apache.catalina.core.StandardContext.reload(StandardContext.java:3913)
at org.apache.catalina.loader.WebappLoader.backgroundProcess(WebappLoader.java:426)
at org.apache.catalina.core.ContainerBase.backgroundProcess(ContainerBase.java:1345)
at org.apache.catalina.core.ContainerBase$ContainerBackgroundProcessor.processChildren(ContainerBase.java:1530)
at org.apache.catalina.core.ContainerBase$ContainerBackgroundProcessor.processChildren(ContainerBase.java:1540)
at org.apache.catalina.core.ContainerBase$ContainerBackgroundProcessor.processChildren(ContainerBase.java:1540)
at org.apache.catalina.core.ContainerBase$ContainerBackgroundProcessor.run(ContainerBase.java:1519)
at java.lang.Thread.run(Unknown Source)
Caused by: java.lang.NoClassDefFoundError: org/apache/log4j/spi/ThrowableInformation
at org.apache.log4j.spi.LoggingEvent.(LoggingEvent.java:145)
at org.apache.log4j.Category.forcedLog(Category.java:372)
at org.apache.log4j.Category.log(Category.java:864)
at org.apache.commons.logging.impl.Log4JLogger.error(Log4JLogger.java:251)
at org.springframework.beans.factory.support.DefaultSingletonBeanRegistry.destroyBean(DefaultSingletonBeanRegistry.java:490)
at org.springframework.beans.factory.support.DefaultSingletonBeanRegistry.destroySingleton(DefaultSingletonBeanRegistry.java:463)
at org.springframework.beans.factory.support.DefaultSingletonBeanRegistry.destroySingletons(DefaultSingletonBeanRegistry.java:431)
at org.springframework.context.support.AbstractApplicationContext.destroyBeans(AbstractApplicationContext.java:1048)
at org.springframework.context.support.AbstractApplicationContext.doClose(AbstractApplicationContext.java:1022)
at org.springframework.context.support.AbstractApplicationContext.close(AbstractApplicationContext.java:970)
at org.springframework.web.servlet.FrameworkServlet.destroy(FrameworkServlet.java:737)
at org.apache.catalina.core.StandardWrapper.unload(StandardWrapper.java:1464)
… 12 more
Caused by: java.lang.ClassNotFoundException: org.apache.log4j.spi.ThrowableInformation
at org.apache.catalina.loader.WebappClassLoader.loadClass(WebappClassLoader.java:1714)
at org.apache.catalina.loader.WebappClassLoader.loadClass(WebappClassLoader.java:1559)
… 24 more
Sep 04, 2013 4:55:16 PM org.apache.catalina.core.StandardContext resourcesStart
SEVERE: Error starting static Resources
java.lang.IllegalArgumentException: Document base E:\ViniWorkSpace\pooja\performance\.metadata\.plugins\org.eclipse.wst.server.core\tmp1\wtpwebapps\HelloWorld does not exist or is not a readable directory
at org.apache.naming.resources.FileDirContext.setDocBase(FileDirContext.java:138)
at org.apache.catalina.core.StandardContext.resourcesStart(StandardContext.java:4906)
at org.apache.catalina.core.StandardContext.startInternal(StandardContext.java:5086)
at org.apache.catalina.util.LifecycleBase.start(LifecycleBase.java:150)
at org.apache.catalina.core.StandardContext.reload(StandardContext.java:3920)
at org.apache.catalina.loader.WebappLoader.backgroundProcess(WebappLoader.java:426)
at org.apache.catalina.core.ContainerBase.backgroundProcess(ContainerBase.java:1345)
at org.apache.catalina.core.ContainerBase$ContainerBackgroundProcessor.processChildren(ContainerBase.java:1530)
at org.apache.catalina.core.ContainerBase$ContainerBackgroundProcessor.processChildren(ContainerBase.java:1540)
at org.apache.catalina.core.ContainerBase$ContainerBackgroundProcessor.processChildren(ContainerBase.java:1540)
at org.apache.catalina.core.ContainerBase$ContainerBackgroundProcessor.run(ContainerBase.java:1519)
at java.lang.Thread.run(Unknown Source)
Sep 04, 2013 4:55:16 PM org.apache.catalina.core.StandardContext startInternal
SEVERE: Error in resourceStart()
Sep 04, 2013 4:55:16 PM org.apache.catalina.core.StandardContext startInternal
SEVERE: Error getConfigured
Sep 04, 2013 4:55:16 PM org.apache.catalina.core.StandardContext startInternal
SEVERE: Context [/HelloWorld] startup failed due to previous errors
Sep 04, 2013 4:55:16 PM org.apache.catalina.util.LifecycleBase stop
INFO: The stop() method was called on component [WebappLoader[/HelloWorld]] after stop() had already been called. The second call will be ignored.
Sep 04, 2013 4:55:16 PM org.apache.catalina.core.StandardContext reload
INFO: Reloading Context with name [/HelloWorld] is completed
Hi joe,
I have sorted it out, the problem was with the server port numbers…!
Thank you
Really good Explanation
Thank you sir. It is easy to understand and very good explaining.
very brief.
You rock man.Easy explanation to any complex thing.
Thanks alot. Keep going.
Hi Joe
I ve tried to do the examples. Everything seems to work well, and I deployed it on Tomcat 7. The deployment also went well
But I got the HTTP 404 error when requesting the link from a browser. Do I made something wrong
Extra info: I m using Eclipse juno with Spring plugins, Apache Tomcat 7
The requested link in the browser is:
http://localhost:8080/SpringMVC
Thank You very much…it helped me alot
Hi Joe , Now i want to run my application in Browser not in eclipse how can i do it ?
found the answer..we have to export the project into WAR file .using tomcat manager-gui we can deploy the WAr file andn our application in ready to use.
[…] about export as excel feature using Spring MVC framework. If you are a beginner, go through the Spring MVC tutorial before taking this. We will be using Spring 3 annotation based approach for the web […]
thank you
[…] Spring’s interceptor can be configured for all the requests (for any URI’s requested) or for a group of URI’s (may be for a set of modules, etc.). Just remember controller and handler are the same. If you are a beginner in Spring, to better understand interceptor, please go through the Spring 3 MVC tutorial. […]
Your every example are so simple!
I appreciate all the time you take to make we all understand the concepts.
very nice…..
INFO: Loading XML bean definitions from ServletContext resource [/WEB-INF/dispatcher-servlet.xml]
Nov 13, 2013 9:30:09 AM org.springframework.web.servlet.FrameworkServlet initServletBean
SEVERE: Context initialization failed
org.springframework.beans.factory.BeanDefinitionStoreException: Unexpected exception parsing XML document from ServletContext resource [/WEB-INF/dispatcher-servlet.xml]; nested exception is java.security.AccessControlException: access denied (“java.lang.RuntimePermission” “accessClassInPackage.org.apache.catalina.loader”)
at org.springframework.beans.factory.xml.XmlBeanDefinitionReader.doLoadBeanDefinitions(XmlBeanDefinitionReader.java:420)
at org.springframework.beans.factory.xml.XmlBeanDefinitionReader.loadBeanDefinitions(XmlBeanDefinitionReader.java:342)
at org.springframework.beans.factory.xml.XmlBeanDefinitionReader.loadBeanDefinitions(XmlBeanDefinitionReader.java:310)
at org.springframework.beans.factory.support.AbstractBeanDefinitionReader.loadBeanDefinitions(AbstractBeanDefinitionReader.java:143)
at org.springframework.beans.factory.support.AbstractBeanDefinitionReader.loadBeanDefinitions(AbstractBeanDefinitionReader.java:178)
at org.springframework.beans.factory.support.AbstractBeanDefinitionReader.loadBeanDefinitions(AbstractBeanDefinitionReader.java:149)
at org.springframework.web.context.support.XmlWebApplicationContext.loadBeanDefinitions(XmlWebApplicationContext.java:124)
at org.springframework.web.context.support.XmlWebApplicationContext.loadBeanDefinitions(XmlWebApplicationContext.java:92)
at org.springframework.context.support.AbstractRefreshableApplicationContext.refreshBeanFactory(AbstractRefreshableApplicationContext.java:123)
at org.springframework.context.support.AbstractApplicationContext.obtainFreshBeanFactory(AbstractApplicationContext.java:422)
at org.springframework.context.support.AbstractApplicationContext.refresh(AbstractApplicationContext.java:352)
at org.springframework.web.servlet.FrameworkServlet.createWebApplicationContext(FrameworkServlet.java:402)
at org.springframework.web.servlet.FrameworkServlet.initWebApplicationContext(FrameworkServlet.java:316)
at org.springframework.web.servlet.FrameworkServlet.initServletBean(FrameworkServlet.java:282)
at org.springframework.web.servlet.HttpServletBean.init(HttpServletBean.java:126)
at javax.servlet.GenericServlet.init(GenericServlet.java:160)
…
Caused by: java.security.AccessControlException: access denied (“java.lang.RuntimePermission” “accessClassInPackage.org.apache.catalina.loader”)
at java.security.AccessControlContext.checkPermission(AccessControlContext.java:366)
while i am running the tomcat i a getting this error i am using spring security
I tried this but i am facing the following problem……….
org.springframework.web.servlet.DispatcherServlet noHandlerFound
WARNING: No mapping found for HTTP request with URI [/SpringMVC/view/hi] in DispatcherServlet with name ‘springDispatcher’
Can anyone provide solution to this…………
Hi Joe
Its an awesome article.
I have one question in this point
“Response is constructed and control sent back to DispatcherServlet”
my question is who creates a response and send control back to DispatcherServlet?
Worked !!. Thank you. Had tried more than 10 which failed before this.
can you suggest some good book for spring framework. I googled,but not sure.
very nice
Its Really very helpful….Thanks
thanks joe
clear explanation and i like your way explanation
google for it
Hi Joe,
It was nice example, but when i try the same on my eclipse with tomcat 7x i get below error not sure what is missing.
Mar 04, 2014 2:23:52 PM org.apache.catalina.core.ContainerBase startInternal
SEVERE: A child container failed during start
java.util.concurrent.ExecutionException: org.apache.catalina.LifecycleException: Failed to start component [StandardEngine[Catalina].StandardHost[localhost].StandardContext[/JoeTestMVC]]
at java.util.concurrent.FutureTask$Sync.innerGet(FutureTask.java:252)
at java.util.concurrent.FutureTask.get(FutureTask.java:111)
at org.apache.catalina.core.ContainerBase.startInternal(ContainerBase.java:1123)
at org.apache.catalina.core.StandardHost.startInternal(StandardHost.java:799)
at org.apache.catalina.util.LifecycleBase.start(LifecycleBase.java:150)
at org.apache.catalina.core.ContainerBase$StartChild.call(ContainerBase.java:1559)
at org.apache.catalina.core.ContainerBase$StartChild.call(ContainerBase.java:1549)
at java.util.concurrent.FutureTask$Sync.innerRun(FutureTask.java:334)
at java.util.concurrent.FutureTask.run(FutureTask.java:166)
at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1145)
at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:615)
at java.lang.Thread.run(Thread.java:724)
Caused by: org.apache.catalina.LifecycleException: Failed to start component [StandardEngine[Catalina].StandardHost[localhost].StandardContext[/JoeTestMVC]]
at org.apache.catalina.util.LifecycleBase.start(LifecycleBase.java:154)
… 7 more
Caused by: java.lang.NullPointerException
at org.springframework.web.SpringServletContainerInitializer.onStartup(SpringServletContainerInitializer.java:142)
at org.apache.catalina.core.StandardContext.startInternal(StandardContext.java:5456)
at org.apache.catalina.util.LifecycleBase.start(LifecycleBase.java:150)
… 7 more
Mar 04, 2014 2:23:52 PM org.apache.catalina.core.ContainerBase startInternal
SEVERE: A child container failed during start
java.util.concurrent.ExecutionException: org.apache.catalina.LifecycleException: Failed to start component [StandardEngine[Catalina].StandardHost[localhost]]
at java.util.concurrent.FutureTask$Sync.innerGet(FutureTask.java:252)
at java.util.concurrent.FutureTask.get(FutureTask.java:111)
at org.apache.catalina.core.ContainerBase.startInternal(ContainerBase.java:1123)
at org.apache.catalina.core.StandardEngine.startInternal(StandardEngine.java:300)
at org.apache.catalina.util.LifecycleBase.start(LifecycleBase.java:150)
at org.apache.catalina.core.StandardService.startInternal(StandardService.java:443)
at org.apache.catalina.util.LifecycleBase.start(LifecycleBase.java:150)
at org.apache.catalina.core.StandardServer.startInternal(StandardServer.java:731)
at org.apache.catalina.util.LifecycleBase.start(LifecycleBase.java:150)
at org.apache.catalina.startup.Catalina.start(Catalina.java:689)
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57)
at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
at java.lang.reflect.Method.invoke(Method.java:606)
at org.apache.catalina.startup.Bootstrap.start(Bootstrap.java:321)
at org.apache.catalina.startup.Bootstrap.main(Bootstrap.java:455)
Caused by: org.apache.catalina.LifecycleException: Failed to start component [StandardEngine[Catalina].StandardHost[localhost]]
at org.apache.catalina.util.LifecycleBase.start(LifecycleBase.java:154)
at org.apache.catalina.core.ContainerBase$StartChild.call(ContainerBase.java:1559)
at org.apache.catalina.core.ContainerBase$StartChild.call(ContainerBase.java:1549)
at java.util.concurrent.FutureTask$Sync.innerRun(FutureTask.java:334)
at java.util.concurrent.FutureTask.run(FutureTask.java:166)
at java.util.concurrent.ThreadPoolExecutor.runWorker(ThreadPoolExecutor.java:1145)
at java.util.concurrent.ThreadPoolExecutor$Worker.run(ThreadPoolExecutor.java:615)
at java.lang.Thread.run(Thread.java:724)
Caused by: org.apache.catalina.LifecycleException: A child container failed during start
at org.apache.catalina.core.ContainerBase.startInternal(ContainerBase.java:1131)
at org.apache.catalina.core.StandardHost.startInternal(StandardHost.java:799)
at org.apache.catalina.util.LifecycleBase.start(LifecycleBase.java:150)
… 7 more
Mar 04, 2014 2:23:52 PM org.apache.catalina.startup.Catalina start
SEVERE: The required Server component failed to start so Tomcat is unable to start.
org.apache.catalina.LifecycleException: Failed to start component [StandardServer[8005]]
at org.apache.catalina.util.LifecycleBase.start(LifecycleBase.java:154)
at org.apache.catalina.startup.Catalina.start(Catalina.java:689)
at sun.reflect.NativeMethodAccessorImpl.invoke0(Native Method)
at sun.reflect.NativeMethodAccessorImpl.invoke(NativeMethodAccessorImpl.java:57)
at sun.reflect.DelegatingMethodAccessorImpl.invoke(DelegatingMethodAccessorImpl.java:43)
at java.lang.reflect.Method.invoke(Method.java:606)
at org.apache.catalina.startup.Bootstrap.start(Bootstrap.java:321)
at org.apache.catalina.startup.Bootstrap.main(Bootstrap.java:455)
Caused by: org.apache.catalina.LifecycleException: Failed to start component [StandardService[Catalina]]
at org.apache.catalina.util.LifecycleBase.start(LifecycleBase.java:154)
at org.apache.catalina.core.StandardServer.startInternal(StandardServer.java:731)
at org.apache.catalina.util.LifecycleBase.start(LifecycleBase.java:150)
… 7 more
Caused by: org.apache.catalina.LifecycleException: Failed to start component [StandardEngine[Catalina]]
at org.apache.catalina.util.LifecycleBase.start(LifecycleBase.java:154)
at org.apache.catalina.core.StandardService.startInternal(StandardService.java:443)
at org.apache.catalina.util.LifecycleBase.start(LifecycleBase.java:150)
… 9 more
Caused by: org.apache.catalina.LifecycleException: A child container failed during start
at org.apache.catalina.core.ContainerBase.startInternal(ContainerBase.java:1131)
at org.apache.catalina.core.StandardEngine.startInternal(StandardEngine.java:300)
at org.apache.catalina.util.LifecycleBase.start(LifecycleBase.java:150)
… 11 more
Mar 04, 2014 2:23:52 PM org.apache.coyote.AbstractProtocol pause
INFO: Pausing ProtocolHandler [“http-bio-8080”]
Mar 04, 2014 2:23:52 PM org.apache.coyote.AbstractProtocol pause
INFO: Pausing ProtocolHandler [“ajp-bio-8009”]
Mar 04, 2014 2:23:52 PM org.apache.catalina.core.StandardService stopInternal
INFO: Stopping service Catalina
Mar 04, 2014 2:23:52 PM org.apache.coyote.AbstractProtocol destroy
INFO: Destroying ProtocolHandler [“http-bio-8080”]
Mar 04, 2014 2:23:52 PM org.apache.coyote.AbstractProtocol destroy
INFO: Destroying ProtocolHandler [“ajp-bio-8009”]
really helpful document.thnx
org.springframework.beans.factory.BeanDefinitionStoreException: Failed to read candidate component class: file [D:\eclipse-jee-kepler-SR1-win32\.metadata\.plugins\org.eclipse.wst.server.core\tmp0\wtpwebapps\Springweb\WEB-INF\classes\com\common\eteam\controllers\GenericController.class]; nested exception is java.lang.IncompatibleClassChangeError: class org.springframework.core.type.classreading.ClassMetadataReadingVisitor has interface org.springframework.asm.ClassVisitor as super class
at org.springframework.context.annotation.ClassPathScanningCandidateComponentProvider.findCandidateComponents(ClassPathScanningCandidateComponentProvider.java:301)
No pom.xml in your project how u run it.. I am using maven .. So plz help me how to work with maven