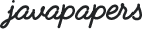
This tutorial is to understand basics of cryptography using modulo 26 polyalphabetic cipher. We will not be using Java Cryptography Extension (JCE) but just core Java. So that it will help us understand the fundamentals of symmetric key cryptography. If you are looking for a safe cryptography implementation for a real time project use, refer Java symmetric AES encryption decryption using JCE tutorial.
A cipher based on substitution using multiple substitution alphabets is polyalphabetic cipher. There are many variations available for polyalphabetic cipher like Vigener cipher. We will use a simple substitution based on a secret key and modulo 26.
For each text in the message there is a corresponding text in the key. So the secret key is equal or more in length than the message to be communicated. We map the alphabets to number as A->1, B->2
etc. Each character from the message and its corresponding character in key is added converted to modulo 26. Then the resulting number is mapped back to a character. For decryption, reverse of the same technique is used. Why modulo 26 as it restricts the character set from A to Z.
package com.javapapers.java.security; public class Modulo26Crypto { public static void main(String[] args) { String plainText = "DROPIT"; String secretKey = "SECRETKEY"; System.out.println("Plain Text Before Encryption: " + plainText); String encryptedText = encrypt(plainText, secretKey); System.out.println("Encrypted Text After Encryption: " + encryptedText); String decryptedText = decrypt(encryptedText, secretKey); System.out.println("Decrypted Text After Decryption: " + decryptedText); } private static String encrypt(String plainText, String secretKey) { StringBuffer encryptedString = new StringBuffer(); int encryptedInt; for (int i = 0; i < plainText.length(); i++) { int plainTextInt = (int) (plainText.charAt(i) - 'A'); int secretKeyInt = (int) (secretKey.charAt(i) - 'A'); encryptedInt = (plainTextInt + secretKeyInt) % 26; encryptedString.append((char) ((encryptedInt) + (int) 'A')); } return encryptedString.toString(); } private static String decrypt(String decryptedText, String secretKey) { StringBuffer decryptedString = new StringBuffer(); int decryptedInt; for (int i = 0; i < decryptedText.length(); i++) { int decryptedTextInt = (int) (decryptedText.charAt(i) - 'A'); int secretKeyInt = (int) (secretKey.charAt(i) - 'A'); decryptedInt = decryptedTextInt - secretKeyInt; if (decryptedInt < 1) decryptedInt += 26; decryptedString.append((char) ((decryptedInt) + (int) 'A')); } return decryptedString.toString(); } }
Plain Text Before Encryption: DROPIT Encrypted Text After Encryption: VVQGMM Decrypted Text After Decryption: DROPIT
Comments are closed for "Simple Encryption Decryption with Modulo 26 Polyalphabetic Cipher".
nice…Thank you keep it up.
plainText.charAt(i) – ‘A’
What does this mean..?
Plain Text Before Encryption: DROPPED
Encrypted Text After Encryption: VVQGTXN
Decrypted Text After Decryption: NZSXXQX
This is what I got the result
I am using jdk1.7 for Compiling
ANy Update onthis
I didn’t get the exact output of above sample program
please explained me above sample program.