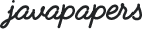
In this Java tutorial we will see about what PBE is and how we can use it in Java to encrypt and decrypt a file. In Password based encryption (PBE), a password is chosen and it is used along with a generated salt (key) to encrypt. Then the same password is used along with the salt again to decrypt the file.
The chosen password is exchanged between the parties. This is a type of symmetric key encryption and decryption technique.
This tutorial is a continuation of our Java security series. In the previous tutorial we saw about symmetric AES encryption and decryption using Java Cryptography Extension (JCE).
In the following example, we will have a simple text file for example and use it to encrypt and decrypt it. Any type of file can be used. We use a password phrase as “javapapers” and a salt is generated then "PBEWithMD5AndTripleDES” used for key generation, hashing and encryption. The iteration count used in the example is 100 and larger the number it increases the key space. Salt is written in the file itself.
package com.javapapers.java.security; import java.io.FileInputStream; import java.io.FileOutputStream; import java.util.Random; import javax.crypto.Cipher; import javax.crypto.SecretKey; import javax.crypto.SecretKeyFactory; import javax.crypto.spec.PBEKeySpec; import javax.crypto.spec.PBEParameterSpec; public class FileEncryption { public static void main(String[] args) throws Exception { FileInputStream inFile = new FileInputStream("plainfile.txt"); FileOutputStream outFile = new FileOutputStream("plainfile.des"); String password = "javapapers"; PBEKeySpec pbeKeySpec = new PBEKeySpec(password.toCharArray()); SecretKeyFactory secretKeyFactory = SecretKeyFactory .getInstance("PBEWithMD5AndTripleDES"); SecretKey secretKey = secretKeyFactory.generateSecret(pbeKeySpec); byte[] salt = new byte[8]; Random random = new Random(); random.nextBytes(salt); PBEParameterSpec pbeParameterSpec = new PBEParameterSpec(salt, 100); Cipher cipher = Cipher.getInstance("PBEWithMD5AndTripleDES"); cipher.init(Cipher.ENCRYPT_MODE, secretKey, pbeParameterSpec); outFile.write(salt); byte[] input = new byte[64]; int bytesRead; while ((bytesRead = inFile.read(input)) != -1) { byte[] output = cipher.update(input, 0, bytesRead); if (output != null) outFile.write(output); } byte[] output = cipher.doFinal(); if (output != null) outFile.write(output); inFile.close(); outFile.flush(); outFile.close(); } }
package com.javapapers.java.security; import java.io.FileInputStream; import java.io.FileOutputStream; import javax.crypto.Cipher; import javax.crypto.SecretKey; import javax.crypto.SecretKeyFactory; import javax.crypto.spec.PBEKeySpec; import javax.crypto.spec.PBEParameterSpec; public class FileDecryption { public static void main(String[] args) throws Exception { String password = "javapapers"; PBEKeySpec pbeKeySpec = new PBEKeySpec(password.toCharArray()); SecretKeyFactory secretKeyFactory = SecretKeyFactory .getInstance("PBEWithMD5AndTripleDES"); SecretKey secretKey = secretKeyFactory.generateSecret(pbeKeySpec); FileInputStream fis = new FileInputStream("plainfile.des"); byte[] salt = new byte[8]; fis.read(salt); PBEParameterSpec pbeParameterSpec = new PBEParameterSpec(salt, 100); Cipher cipher = Cipher.getInstance("PBEWithMD5AndTripleDES"); cipher.init(Cipher.DECRYPT_MODE, secretKey, pbeParameterSpec); FileOutputStream fos = new FileOutputStream("plainfile_decrypted.txt"); byte[] in = new byte[64]; int read; while ((read = fis.read(in)) != -1) { byte[] output = cipher.update(in, 0, read); if (output != null) fos.write(output); } byte[] output = cipher.doFinal(); if (output != null) fos.write(output); fis.close(); fos.flush(); fos.close(); } }
When you run these programs, you should first create a file named "plainfile.txt" at the project root classpath. One successfully run, the first program will create an encrypted version of this input file and the second program will create the decrypted version of the encrypted file.
I am using Java SE 8 and have installed the “Java Cryptography Extension (JCE) Unlimited Strength Jurisdiction Policy Files 8 Download”. Illegal key size message error (“Wrong key size”) can be avoided by installing it.
Comments are closed for "Java File Encryption Decryption using Password Based Encryption (PBE)".
[…] based encryption (PBE) to encrypt and decrypt a file. In the previous tutorial we saw about using TripleDES PBE to encrypt and decrypt a file. AES is more advanced and secure than […]
I have a problem. i have encrypted some pic in my mobile. after some times the app was deleted and not found in the app store.. so i could not decrypt my pics.
pleas help in decryption of .fpenc file..
This works good for files but could you help me in pbe based encryption and decryption for a text/String in java.Thanks in advance.
Its not supported for multiple file with different extension
First I encrypt the .class file and .ser file. After encryption. I decrypt the .class file. its not work. throws following error.
com.ppts.torq.concrete.PropUtil$CryptoException: Error encrypting/decrypting file
at com.ppts.torq.concrete.PropUtil.doDeCrypto(PropUtil.java:155)
at com.ppts.torq.controller.CreateScriptNByPassFiles.buildScriptFile(CreateScriptNByPassFiles.java:57)
at com.ppts.torq.controller.CreateScriptNByPassFiles.main(CreateScriptNByPassFiles.java:24)
Caused by: javax.crypto.BadPaddingException: Given final block not properly padded
at com.sun.crypto.provider.CipherCore.doFinal(CipherCore.java:966)
at com.sun.crypto.provider.CipherCore.doFinal(CipherCore.java:824)
at com.sun.crypto.provider.AESCipher.engineDoFinal(AESCipher.java:436)
at javax.crypto.Cipher.doFinal(Cipher.java:2004)
at com.ppts.torq.concrete.PropUtil.doDeCrypto(PropUtil.java:146)
… 2 more