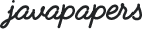
In this tutorial we will learn about AES symmetric encryption decryption using Java Cryptography Extension (JCE). In the previous tutorial we saw about encryption decryption using DES symmetric key algorithm. “Data Encryption Standard (DES)” is prone to brute-force attacks. It is a old way of encrypting data. It is replaced by “Advanced Encryption Standard (AES)”.
Main issue with DES was the short encryption key size. Shorter the key, it is easier to break it with brute force attack. AES can use 128, 192 or 256 bit encryption. The block size used by DES is 64 bits and by AES is 128 bits. Third difference is AES uses permutation substitution over the Feistel network used by DES.
If you are looking to choose between DES or AES for your real time application, AES is the way to go.
In comparison from the previous tutorial there are only two changes in the example program. One is the AES encryption and another is Base64 encoding.
If it were before Java 8, I would have used the Apache commons-code bundle for Base64 encoding. In Java 8 we have got new classes in java.util
package for Base64 encoding and decoding. It is important to encode the binary data with Base64 to ensure it to be intact without modification when it is stored or transferred.
package com.javapapers.java.security; import java.util.Base64; import javax.crypto.Cipher; import javax.crypto.KeyGenerator; import javax.crypto.SecretKey; public class EncryptionDecryptionAES { static Cipher cipher; public static void main(String[] args) throws Exception { KeyGenerator keyGenerator = KeyGenerator.getInstance("AES"); keyGenerator.init(128); SecretKey secretKey = keyGenerator.generateKey(); cipher = Cipher.getInstance("AES"); String plainText = "AES Symmetric Encryption Decryption"; System.out.println("Plain Text Before Encryption: " + plainText); String encryptedText = encrypt(plainText, secretKey); System.out.println("Encrypted Text After Encryption: " + encryptedText); String decryptedText = decrypt(encryptedText, secretKey); System.out.println("Decrypted Text After Decryption: " + decryptedText); } public static String encrypt(String plainText, SecretKey secretKey) throws Exception { byte[] plainTextByte = plainText.getBytes(); cipher.init(Cipher.ENCRYPT_MODE, secretKey); byte[] encryptedByte = cipher.doFinal(plainTextByte); Base64.Encoder encoder = Base64.getEncoder(); String encryptedText = encoder.encodeToString(encryptedByte); return encryptedText; } public static String decrypt(String encryptedText, SecretKey secretKey) throws Exception { Base64.Decoder decoder = Base64.getDecoder(); byte[] encryptedTextByte = decoder.decode(encryptedText); cipher.init(Cipher.DECRYPT_MODE, secretKey); byte[] decryptedByte = cipher.doFinal(encryptedTextByte); String decryptedText = new String(decryptedByte); return decryptedText; } }
Plain Text Before Encryption: AES Symmetric Encryption Decryption Encrypted Text After Encryption: sY6vkQrWRg0fvRzbqSAYxepeBIXg4AySj7Xh3x4vDv8TBTkNiTfca7wW/dxiMMJl Decrypted Text After Decryption: AES Symmetric Encryption Decryption
Comments are closed for "Java Symmetric AES Encryption Decryption using JCE".
[…] is the latest encryption standard over the DES. You can refer the encryption decryption with AES symmetric algorithm using JCE tutorial. All the above given steps and concept are same, we just replace the DES with […]
[…] This tutorial is a continuation of our Java security series. In the previous tutorial we saw about symmetric AES encryption and decryption using Java Cryptography Extension (JCE). […]
when i change keyGenerator.init(128); to keyGenerator.init(192); or keyGenerator.init(256);
it throws exception
Exception in thread “main” java.security.InvalidKeyException: Illegal key size or default parameters
at javax.crypto.Cipher.checkCryptoPerm(Cipher.java:1021)
at javax.crypto.Cipher.implInit(Cipher.java:796)
at javax.crypto.Cipher.chooseProvider(Cipher.java:859)
at javax.crypto.Cipher.init(Cipher.java:1229)
at javax.crypto.Cipher.init(Cipher.java:1166)
at algotesting.AlgoTesting.encrypt(AlgoTesting.java:52)
at algotesting.AlgoTesting.main(AlgoTesting.java:37)
i used netbean ide7, but theres an arror here Base64.Encoder encoder = Base64.getEncoder();
would you help me?thank you