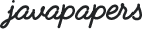
This Java tutorial is part of our Java concurrent collections tutorial series. In this tutorial we will see about Java ConcurrentHashMap.
ConcurrentHashMap is a hash table which supports complete concurrency for retrievals and updates. ConcurrentHashMap
follows the specifications of a Hashtable. ConcurrentHashMap does not lock the entire collection for synchronization. ConcurrentHashMap is a suited candidate collection when there are high number of updates and less number of read concurrently.
ConcurrentHashMap implements ConcurrentMap
which lays the blue print for the concurrent operations. This was introduced part of the JDK 1.5 in the Java collections framework.
ConcurrentHashMap(int initialCapacity, float loadFactor, int concurrencyLevel)
ConcurrentHashMap comes with multiple constructors and above being one among them. initialCapacity is to fix the internal size of the collection. Hash table density is decided based on the loadFactor. Now this is new, concurrencyLevel. By default ConcurrentHashMap allows 16 number of concurrent threads. We can change this number using the concurrencyLevl argument. Most of the times 16 should be sufficient and playing with these number may cause undesirable performance issues. Know before you set these arguments.
ConcurrentHashMap does not throw ConcurrentModificationException
if the underlying collection is modified during an iteration is in progress. Iterators may not reflect the exact state of the collection if it is being modified concurrently. It may reflect the state when it was created and at some moment later. The fail-safe property is given a guarantee based on this.
ConcurrentHashMap can be considered as an alternative to Hashtable. It is a better in comparison with a Hashtable and a synchronized Map. ConcurrentHashMap blocks only the parts as required and provides ultimate concurrency. ConcurrentHashMap by default is separated into 16 regions and locks are applied. This default number can be set while initializing a ConcurrentHashMap instance.
null
is not allowed as a key or value in ConcurrentHashMap.package com.javapapers.java.collections; import java.util.Map; import java.util.concurrent.ConcurrentHashMap; public class ConcurrentHashMapExample { public static void main(String[] args) { ConcurrentHashMapconcurrentHashMap = new ConcurrentHashMap (); concurrentHashMap.put("A","Apple"); concurrentHashMap.put("B","Blackberry"); for (Map.Entry e : concurrentHashMap.entrySet()) { System.out.println(e.getKey() + " = " + e.getValue()); } } }
Comments are closed for "Java ConcurrentHashMap".
[…] with all the above operations of a NavigableMap it also extends the ConcurrentMap interface. So this is a super-dooper Map we […]
Hi,
I am still not clear whether get method of concurrenthashmap is blocking or non-blocking.
If it is non-blocking then why?
Can you please explain this part?
Thanks!!
Amit.
Hi Joe,
Can you explain why null is not allowed as a key or value in ConcurrentHashMap?
Thanks,
Sreenath