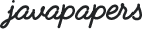
When we want to represent part-whole hierarchy, use tree structure and compose objects. We know tree structure what a tree structure is and some of us don’t know what a part-whole hierarchy is. A system consists of subsystems or components. Components can further be divided into smaller components. Further smaller components can be divided into smaller elements. This is a part-whole hierarchy.
Everything around us can be a candidate for part-whole hierarchy. Human body, a car, a computer, lego structure, etc. A car is made up of engine, tyre, … Engine is made up of electrical components, valves, … Electrical components is made up of chips, transistor, … Like this a component is part of a whole system. This hierarchy can be represented as a tree structure using composite design pattern.
“Compose objects into tree structures to represent part-whole hierarchies. Composite lets clients treat individual objects and compositions of objects uniformly.” is the intent by GoF.
In this article, let us take a real world example of part-whole hierarchy and use composite design pattern using java. As a kid, I have spent huge amount of time with lego building blocks. Last week I bought my son an assorted kit lego and we spent the whole weekend together building structures.
Let us consider the game of building blocks to practice composite pattern. Assume that our kit has only three unique pieces ( 1, 2 and 4 blocks) and let us call these as primitive blocks as they will be the end nodes in the tree structure. Objective is to build a house and it will be a step by step process. First using primitive blocks, we should construct multiple windows, doors, walls, floor and let us call these structures. Then use all these structure to create a house.
Primitive blocks combined together gives a structure. Multiple structures assembled together gives a house.
When we get a recursive structure the obvious choice for implementation is a tree. In composite design pattern, the part-whole hierarchy can be represented as a tree. Leaves (end nodes) of a tree being the primitive elements and the tree being the composite structure.
Component: (structure)
Leaf: (primitive blocks)
Composite: (group)
package com.javapapers.designpattern.composite; public class Block implements Group { public void assemble() { System.out.println("Block"); } }
package com.javapapers.designpattern.composite; public interface Group { public void assemble(); }
package com.javapapers.designpattern.composite; import java.util.ArrayList; import java.util.List; public class Structure implements Group { // Collection of child groups. private Listgroups = new ArrayList (); public void assemble() { for (Group group : groups) { group.assemble(); } } // Adds the group to the structure. public void add(Group group) { groups.add(group); } // Removes the group from the structure. public void remove(Group group) { groups.remove(group); } }
package com.javapapers.designpattern.composite; public class ImplementComposite { public static void main(String[] args) { //Initialize three blocks Block block1 = new Block(); Block block2 = new Block(); Block block3 = new Block(); //Initialize three structure Structure structure = new Structure(); Structure structure1 = new Structure(); Structure structure2 = new Structure(); //Composes the groups structure1.add(block1); structure1.add(block2); structure2.add(block3); structure.add(structure1); structure.add(structure2); structure.assemble(); } }
Comments are closed for "Composite Design Pattern".
Thanks joe..
really great explanation.
can you specify when to use this pattern?
super Joe……Excelent
Can you, if sometime, to apply this pattern to JTree in next your post, or I did not understand the essence of this pattern.Thanks Joe.
Thanks Joe
excellent information for a genuine java developers….thanks mate and pls carry on and give some more information….good night buddy….
Keep up the good work joe
Awesome ………. great explanation
hi joe its good articles and also I am expecting scenarios like where we can implent this type of Design pattern
Hi Joe, Great Explanation and good Presentation.Keep it up Excellent…Thank a lot Joe
Awesome example & explanation. Thanks Joe i m a regular reader of your blogs and every time i came to know a new thing even though i m familiar with it.
Awesome example & explanation. Thanks Joe i m a regular reader of your blogs and every time i came to know a new thing even though i m familiar with it.
Thanks Joe…
I really did not understand what flaw are we trying to eradicate here. Don’t we do coding like this even if we are not aware of this design pattern. I mean why do we call it a pattern and not just a requirement that anyhow will be done that way . What was the repeated mistake that this particular approach has been suggested ,documented and recommended for?
Thanks for the article, but does your structure diagram and the implementation of the code match?
Thanks Joe ,
You are really good in explanation . Using simple language and good examples …
Helped me a lot for tomorrow’s exam.
Keep it up
hai joe…
what is the difference between POJO and java bean… i know some what basic difference but i am not satisfied with that please explain briefly…..
thank you….
its really nice explanation to understand the pattern
Thanks Joe :)
Thankx joe
Nice one.. :)
Wonderful blog. Thanks Joe !!
Hi Joe,
if you run your example, what do you excpect to have in output?
Joe, what do you expect to have in output after you run ImplementComposit example?
Nice article
Good one !!
nice one
I have a question. We are creating a beverage vending machine as an assignment. In this, there might be 2 different kinds of coffees. Each one , I would think would be a composite, whose leafs might be a cup, sugar, cream etc. So you might have a regular coffee with sugar cream and a decaf composite coffee with sugar and cream but in a tree, that would be duplicating sugar units, for example, several times over, which I would think is not what you would want. Can the same leaf branch off of different components?
really nice explanation
Clear and concise. :-)
Thanks. It helps to see different applications for these design patterns.
can you pls give some couple of scenarios where exactly this can be implemented.You have been silent for the above comments, can I expect your response soon? it would be helpful to us
Nice but example chosen is not so good and practical with composite
Great Job :)
I can’t quite get what’s happening in the definition of the Structure class where you make a list of “Group” items “private List groups = new ArrayList();”?
Could you please explain what’s happening and if it is fine to define a list of an interface type without specifying which implementation to use?
Hello!
you said in the beginning that Component is the structure and Composite is the group, but I think in the example you do it the otherway round, when you write the group is the interface?
nice blog..
waiting for behavioral patterns..
Lovely
Very Nice explanation indeed !.
I have a question with regards to the UML diagram show,if you inherit the Composite from a Component ,then why has the sign of aggregation(diamond)been put next to “Composite”.
Ideally the sign of aggregation comes next to the parent class and in this case Component being the parent class .
Please explain.
Thanks.
Regards….
Nice example,But As per GOF., Component class (in our case Group interface ) should have methods add() and remove(). If not client can not access add or remove methods. In the above example Client can access only operation () method. Let me know if I misunderstand it.
Nice example,But As per GOF., Component class (in our case Group interface ) should have methods add() and remove(). If not client can not access add or remove methods. In the above example Client can access only operation () method. Let me know if I misunderstand it.
Will it be recursive if
structure s = new structure();
s.add(s); ?
Thanks Joe
Simple and clear. thank you.
Thanks joe,for clear understanding of composite view….
Excellent ! Very well explined in layman terms.