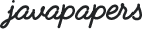
System.gc() is used to invoke the garbage collector and on invocation garbage collector will run to reclaim the unused memory space. It will attempt to free the memory that are occupied by the discarded objects. However System.gc() call comes with a disclaimer that invocation of the garbage collector is not guaranteed. We have got used to the ‘conditions apply’ with our experiences from the real world. Everything comes with a disclaimer!
It is the choice of JVM implementer to decide the behavior of JVM on System.gc() invocation. In general we do not think about memory management when writing our Java code and leave it to the JVM. In some special cases like when we are writing a performance benchmark we may call System.gc() in between runs. Following is another example where invoking gc makes a difference.
MappedByteBuffer is used at places where we need the best IO performance. MappedByteBuffer provides a direct memory mapping to the underlying File.
API Javadoc says,
Many of the details of memory-mapped files are inherently dependent upon the underlying operating system and are therefore unspecified. The behavior of this method when the requested region is not completely contained within this channel’s file is unspecified. Whether changes made to the content or size of the underlying file, by this program or another, are propagated to the buffer is unspecified. The rate at which changes to the buffer are propagated to the file is unspecified.
Almost everything is unspecified and left to the underlying OS. Let us look at the following code,
package com.javapapers.java; import java.io.File; import java.io.IOException; import java.io.RandomAccessFile; import java.nio.MappedByteBuffer; import java.nio.channels.FileChannel; public class MappedFileGc { public static void main(String[] args) throws IOException { File tempFile = File.createTempFile("Temp", null); tempFile.deleteOnExit(); RandomAccessFile raTempFile = new RandomAccessFile(tempFile, "rw"); FileChannel fChannel = raTempFile.getChannel(); MappedByteBuffer mappedBuffer = fChannel.map( FileChannel.MapMode.READ_WRITE, 0, 512); fChannel.close(); raTempFile.close(); mappedBuffer = null; System.gc(); if (tempFile.delete()) System.out.println("Successfully deleted: " + tempFile); else System.out.println("Unable to delete: " + tempFile); } }
In the above code, line number 24 System.gc() is commented. When I run this program now, always I get “Unable to delete: … “. Now lets uncomment the System.gc() and re-run it multiple times to understand the behavior. Now we get “Successfully deleted: …” most of the times. I tested the same with just ByteBuffer and garbage collection happens nice and immediate.
‘Looks like’ since the MappedByteBuffer is heavily OS dependent, the garbage collector is not instantaneous to reclaim. But when we invoke System.gc() garbage collector releases the handle and we are able to delete the file. Shall we make an inference that, “while using MappedByteBuffer and if we are working with memory sensitive program, then its better to call System.gc()”. Note that this behavior is JVM dependent.
Wishing you a happy and prosperous 2014. Thank you for your support to Javapapers all these years and looking forward to your continued patronage. I am feeling fresh and you can expect better articles than ever. Java 8 is coming up and lot more to discover.
Comments are closed for "System.gc() Invocation – A Suitable Scenario".
Hey Joe,
Wishing you a happy new year 2014!
Thanks for this wonderful blog, keep rocking!
Hi Joe,
Wish you a Very Happy and Prosperous New Year 2014!!!
Nice Article.
Happy new Year to you too.
Hey Joe,
I am a Regular reader of your Blog,
I am Java and android Faculty and Regularly Sharing Your Ideas to my Students.
.
Very Thankful to you…
.
Wish you many many Happy New Year. Keep continue your Java, Android MAGIC
I am a regular reader of your every article..
Thanks for your all effort …
Wish u a Happy New year Joe..
Hi Joe,
Wish you happy and Prosperous new year 2014!
Keep up good work!
Hi Joe,
Wish you happy new year 2014!
You are doing wonderful job, Keep it up!
Thanks Joe. Wish you the same and keep sharing :)
Hi Joe
A very happy new year and please keep up the great work.
wish you very happy new year…..
Thanks for that wonderful blog… :)
Hi joe
A very happy new year to you.Thank you for sharing your knowledge.I am a regular reader of your blog and i really like it .
Happy New year Joe :) .. Nice Article !!
Hi Joe,
I am regular reader of your blogs and I like them. They have helped me to improve my knowledge.
Thanks and keep up this work.
Wish you a very Happy New Year!
Regards,
Rupali
Hi
Good Stuff…..Keep it up!!!!!!!!!!!!
i wish you Happy New Year.
Happy new year.
Do you please share your ideas on spring security and Spring MVC.
Thanks
Arup
Hey Joe, Wishing you a very happy new year!! Thanks a lot for sharing your knowledge and magical tips which is really helpful to us!!
Hey Joe, Wishing you Happy and Prosperous New Year-2014
Your every article is a source of deep knowledge. Thanks a lot for your effort and sharing with us.
Happy new year joe
I am a regular reader of your website. Today I learnt something new !!
Thanks Joe :)
Hi Joe,
I m too late but I want to know whether the file will get deleted instantly if it shows “Successfully Deleted” or not. As I ran the program but the file was still there with the name Temp*************.tmp
…
[…] invoking System.gc() makes sense. Just go through this article to know about this corner case when System.gc() invocation is applicable. (adsbygoogle = window.adsbygoogle || […]