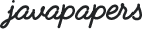
“Java generics” gives the ability to parameterize a type or method and use it in a generic way. A Java class can be defined using a parameter type and the defined parameter can be used inside that class scope as a formal type. For example, in Java collections ArrayList will serve as an apt example for generics.
public class ArrayListextends AbstractList implements List , RandomAccess, Cloneable, Serializable
Initially, Java generics was implemented in order to make the Java collections framework even better. Generics was introduced in J2SE 5.0. Java collections’ classes were not designed to be used for any particular object. For example ArrayList can hold objects of any type. Because it was designed as a container for Java’s base class type Object. Any Java class’ base class is Object and so we can store instance of any class in ArrayList. That is, an ArrayList object can hold a String or Integer or any Java type.
We had problems because of this. When we want to use the collection container in a restrictive way, we are not able to use it. For example, if we wanted to create an ArrayList of Strings, we cannot explicitly force in that way. There is no separate collection type for this and it is not possible to have individual collection types for all these restrictions like ArrayList of Integer, ArrayList of Float etc.
Since we are not able to restrict a collection type (like ArrayList) to hold only a specific type (like Integer), there is a possibility that accidentally a different type may get stored. Code will be written in an assumption that, the collection container will have Integer. If a String is found at that place, we will get Run time exceptions.
package com.javapapers.java; import java.util.ArrayList; public class GenericsIntro { public static void main(String args[]) { ArrayList marksList = init(); ArrayList resultList = add(marksList, 5); } public static ArrayList init() { ArrayList marksList = new ArrayList(); marksList.add(new Integer(94)); marksList.add(new String("Hundred")); marksList.add(new Integer(90)); return marksList; } public static ArrayList add(ArrayList marksList, int increment) { ArrayList resultList = new ArrayList(); for (Object mark : marksList) { int newMark = ((Integer) mark).intValue() + increment; resultList.add(new Integer(newMark)); } return resultList; } }
Exception in thread "main" java.lang.ClassCastException: java.lang.String cannot be cast to java.lang.Integer at com.javapapers.java.GenericsIntro.add(GenericsIntro.java:25) at com.javapapers.java.GenericsIntro.main(GenericsIntro.java:9)
The above example program shows that we will not have any issues at compile time and when someone accidentally stores a type that is not intended and we will get a run time Java exception, ClassCastException.
Java generics solved the above issue by providing compile-time type safety. After generics, when we create a parameterized type we explicitly specify that this object is to be used only with this specified type. So the compiler knows it and at compile time it throws error when other types are attempted to be stored.
Let us come back to our ArrayList and make it generic ArrayList. When we create marksList we should explicitly state that only Integer objects can be stored here using the parameterized type ability provided by Java generics.
package com.javapapers.java; import java.util.ArrayList; public class GenericsTypeSafety { public static void main(String args[]) { ArrayListmarksList = init(); ArrayList resultList = add(marksList, 5); System.out.println(resultList); } public static ArrayList init() { ArrayList marksList = new ArrayList (); marksList.add(new Integer(94)); marksList.add(new String("Hundred")); marksList.add(new Integer(90)); return marksList; } public static ArrayList add(ArrayList marksList, int increment) { ArrayList resultList = new ArrayList (); for (Integer mark : marksList) { int newMark = mark.intValue() + increment; resultList.add(new Integer(newMark)); } return resultList; } }
In the above code, ArrayList object is instantiated by saying explicitly that this can store only objects of type Integer.
ArrayList
The Java compiler is able to identify that an unexpected type’s value is being stored and hence an error is thrown as,
The method add(Integer) in the type ArrayList
Did you notice a change in the above code from the first example. At line number 26 in the for loop where the marksList is iterated, the explicit cast to Integer from Object is removed. In first code we have done explicit typecast from Object to Integer.
Before Generics:
for (Object mark : marksList) { int newMark = ((Integer) mark).intValue() + increment; resultList.add(new Integer(newMark)); }
After Generics:
for (Integer mark : marksList) { int newMark = mark.intValue() + increment; resultList.add(new Integer(newMark)); }
Now generics have enabled us to simplified and readable code. Since the Java compiler knows that the value stored in the ArrayList is only Integer because of parameterize type, the explicit typecast is not required when reading values from the ArrayList.
We have seen only the tip of an iceberg now. Java generics and its features are very large to contain in one tutorial. In continuation to this generics introduction, I will be writing a series of tutorials for Java generics, keep watching.
Comments are closed for "Java Generics Introduction".
Good one! :)
Simple though very important article !
Nice article !!! It helps a lot!!
Wow, Superb
waiting for your next tutorial on this series.
I learned a lot from javapapers thanks Joe for that.
This is really a very good approach to write a series on a topic, so that every one from beginners to advance learner can learn.
Your simplified and point to point way of writing impressed me a lot. I also suggest javapapers to my colleagues for clear understanding of topic.
Again thanks Joe for teaching me Java. You are doing a very good work keep it up Thanks.
Hi Joe,
Good to see your blog on the generics.
Hope i could add up some more. I could see that Generics concept can be applied to any model which could act as container of object alias a box concept, Sometimes Box of Boxes, Secret Box like Map which requires a key to open it, Linked boxes by memory like arraylist or by reference like linked list. Any Data structure of course seems to be a good choice for the application of generics concept.
Eariler we can put anything into a box, now we classify, this box is for toys, the next box is for pens etc.,
Earlier when we expect a toy, we get pens as someone has put it and we wonder why we got it. Now during creation of the box we state what it is supposed to hold and we avoid the unexpected :)
Thanks,
Naren
Simply Superb!!!
Thanks Vijay.
Thanks Gopal.
Sure, this generics series is going to be a rocking one.
Narendran, excellent.
Nice understanding on the generics concept and great way of putting it.
Thanks Karthik.
‘”Java generics” gives the ability to parameterize a type or method’. How does a method can be parameterized using generics.
NIce article , as usual u write..i am crazy about ur blog writing. I feel more confident in technology after reading ur blog.
thanks :)
Very nice sir….
Sir please send me the RESTFull web services material for – Creating webservices
– publishing services
– Creation of client.
With Regards,
Bhimaraya
Waiting for Iceberg :)
:-) Sure, we will see it soon in the coming weeks.
Topic with “Generics” must cover “Type Erasure” – that is the most tricky part to swallow !!
nice explanation..i like the image hen and cock ..! :)
Nice
very very nice explanation………….superb
what is generics
Vishal, more to comeup on this Generics series. I will write tutorials for all these with examples in the coming weeks.
Welcome Atmprakash.
I have written a tutorial on Type Erasure earlier, please refer that:
https://javapapers.com/core-java/type-erasure/
Thanks Muralidhar.
[…] detail let us see an example for generics in Java. This is to recall and continue from our previous tutorial for introduction to generics. In the following example we are using the generics type implemented for collections. This shows […]
you are rock joe..please send ur mail id to my mail.i want subject help from a genious like you
Great article Joe. Thanks
I liked the 2nd image and the article too.
you are doing an excellent work !!
Possible if you send me material on web services .it would be very helpful .. thanks
Hi Joe Your blog Template is very nice. Which website to download this template?
This is custom developed for Javapapers :-)
ok Thanks Bro Your Site Looking Great.Thanks You
I think in the second example with Generics, I think array list should have been created with parameter.
Now I see there is a issue with display of text between symbols.
Hi Joe ,
In GenericsTypeSafety I donot see any code confirming below line.
“ArrayList object is instantiated by saying explicitly that this can store only objects of type Integer.”
Can you please update the program.
Thanks for sharing your knowledge.