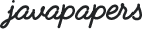
In this tutorial let us discuss about different aspects of type cast with respect to Java generics. Generics was initially idealized for collections framework. In collections there were lots of cast done. In a way, one of the main objective of Java generics is to help programmer reduce explicit type cast and provide type-safety.
Before diving into detail it is better for you to go through the fundamentals of cast in Java. This is tutorial is a part of multi-part series on Java generics. Hope you are comfortable on Java generics basics.
In Java during compilation, the compiler translates the generics type information to raw types and this process is called type erasure. That is the type parameter like <T> are replaced by the left most bound type and in case if there is no bound, then the type parameter is replaced by Object type.
So once this replacement is done, when a method call returns a type parameter, it will obviously return a supertype wherein the expectation will be a specific subtype. So in such places, compiler will explicitly add a cast. Have a look at the following example code and it will help understand this.
package com.javapapers.java; public class Node{ private L leftNode; private R rightNode; public Node(L left, R right) { leftNode = left; rightNode = right; } public L getLeftNode() { return leftNode; } public void setLeftNode(L leftNode) { this.leftNode = leftNode; } public R getRightNode() { return rightNode; } public void setRightNode(R rightNode) { this.rightNode = rightNode; } } class GenericsCast { public static void main(String[] args) { Node node = new Node ("Russel", new Integer(10)); String name = node.getLeftNode(); Integer rank = node.getRightNode(); Object nameAsObj = node.getLeftNode(); System.out.println(name + " " + rank + " " + nameAsObj); } }
Above generic type and the class that uses it will be transformed as below by the Java compiler during type erasure.
So how to identify where the Java compiler will add a cast. During type erasure, if the return type of a method is changed, then mark those methods. Then every place where those methods are invoked should have a cast added. If the argument of a method is changed by type erasure, then it does not matter. Since the argument will be a super type and it can always accept its subtype.
Comments are closed for "Cast in Java Generics".
Simply and Clearly explained :)
Nice work!
Nice as always…
Well done Joe, thanks..
Thanks Vinuraj.
Nicely explained Joe. I have always found the concept of bounded wildcards in Generics. Could you explain how to use lower bounded wildcards with an example?
Hi Joe,
Can you tell me that is there any tool available which can be used as an object spy for java controls?
Means by using any tool will i be able to get the properties of java controls?
Thanks