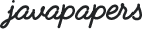
This Android tutorial will walk you through to create an Android application to receive SMS messages in your Android mobile. SMS is popular utility now loosing sheen to Internet based instant messenger services like Whatsapp.
We will be using a BroadcastReceiver
to receive the messages and parse the SMS message out of it and update our UI. We will also show a toast message. In a previous Android tutorial to send SMS we created an app to send SMS messages. These two tutorials combined together will create one complete SMS Android application.
Application uses the basis Android constructs. We should know about using BroadcastReceiver and using Android ListView. Knowing these two is enough to build this Android SMS application.
Couple of things to note in the Android manifest file. Add permission to receive, read and write SMS to the application. Then add the broadcast receiver with an intent-filter to receiving SMS.
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.javapapers.androidreceivesms" > <uses-permission android:name="android.permission.WRITE_SMS" /> <uses-permission android:name="android.permission.READ_SMS" /> <uses-permission android:name="android.permission.RECEIVE_SMS" /> <application android:allowBackup="true" android:icon="@drawable/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name=".SmsActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <receiver android:name=".SmsBroadcastReceiver" android:exported="true" > <intent-filter android:priority="999" > <action android:name="android.provider.Telephony.SMS_RECEIVED" /> </intent-filter> </receiver> </application> </manifest>
This is a BroadcastReceiver
which receives the intent-filtered SMS messages. onReceive
, we extract the SMS message bundle and show a toast message and also update the UI by adding the SMS message to the SMS inbox list. When a SMS message arrives, the inbox is automatically refreshed.
package com.javapapers.androidreceivesms; import android.content.BroadcastReceiver; import android.content.Context; import android.content.Intent; import android.os.Bundle; import android.telephony.SmsMessage; import android.widget.Toast; public class SmsBroadcastReceiver extends BroadcastReceiver { public static final String SMS_BUNDLE = "pdus"; public void onReceive(Context context, Intent intent) { Bundle intentExtras = intent.getExtras(); if (intentExtras != null) { Object[] sms = (Object[]) intentExtras.get(SMS_BUNDLE); String smsMessageStr = ""; for (int i = 0; i < sms.length; ++i) { SmsMessage smsMessage = SmsMessage.createFromPdu((byte[]) sms[i]); String smsBody = smsMessage.getMessageBody().toString(); String address = smsMessage.getOriginatingAddress(); smsMessageStr += "SMS From: " + address + "\n"; smsMessageStr += smsBody + "\n"; } Toast.makeText(context, smsMessageStr, Toast.LENGTH_SHORT).show(); //this will update the UI with message SmsActivity inst = SmsActivity.instance(); inst.updateList(smsMessageStr); } } }
This is the main Android activity of the SMS application. It acts as the SMS inbox. It has a ListView to show the SMS messages. onCreate
we read all the messages present in the internal SMS inbox from its Uri and show them using the ListView
.
package com.javapapers.androidreceivesms; import android.app.Activity; import android.content.ContentResolver; import android.database.Cursor; import android.net.Uri; import android.os.Bundle; import android.view.View; import android.widget.AdapterView; import android.widget.AdapterView.OnItemClickListener; import android.widget.ArrayAdapter; import android.widget.ListView; import android.widget.Toast; import java.util.ArrayList; public class SmsActivity extends Activity implements OnItemClickListener { private static SmsActivity inst; ArrayList<String> smsMessagesList = new ArrayList<String>(); ListView smsListView; ArrayAdapter arrayAdapter; public static SmsActivity instance() { return inst; } @Override public void onStart() { super.onStart(); inst = this; } @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_sms); smsListView = (ListView) findViewById(R.id.SMSList); arrayAdapter = new ArrayAdapter<String>(this, android.R.layout.simple_list_item_1, smsMessagesList); smsListView.setAdapter(arrayAdapter); smsListView.setOnItemClickListener(this); refreshSmsInbox(); } public void refreshSmsInbox() { ContentResolver contentResolver = getContentResolver(); Cursor smsInboxCursor = contentResolver.query(Uri.parse("content://sms/inbox"), null, null, null, null); int indexBody = smsInboxCursor.getColumnIndex("body"); int indexAddress = smsInboxCursor.getColumnIndex("address"); if (indexBody < 0 || !smsInboxCursor.moveToFirst()) return; arrayAdapter.clear(); do { String str = "SMS From: " + smsInboxCursor.getString(indexAddress) + "\n" + smsInboxCursor.getString(indexBody) + "\n"; arrayAdapter.add(str); } while (smsInboxCursor.moveToNext()); } public void updateList(final String smsMessage) { arrayAdapter.insert(smsMessage, 0); arrayAdapter.notifyDataSetChanged(); } public void onItemClick(AdapterView<?> parent, View view, int pos, long id) { try { String[] smsMessages = smsMessagesList.get(pos).split("\n"); String address = smsMessages[0]; String smsMessage = ""; for (int i = 1; i < smsMessages.length; ++i) { smsMessage += smsMessages[i]; } String smsMessageStr = address + "\n"; smsMessageStr += smsMessage; Toast.makeText(this, smsMessageStr, Toast.LENGTH_SHORT).show(); } catch (Exception e) { e.printStackTrace(); } } }
Following is the layout file.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:orientation="vertical" android:layout_width="fill_parent" android:layout_height="fill_parent" android:id="@+id/MainLayout" > <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textAppearance="?android:attr/textAppearanceMedium" android:text="SMS Inbox" android:id="@+id/textView" android:layout_gravity="center_horizontal" /> <ListView android:id="@+id/SMSList" android:layout_height="wrap_content" android:layout_width="match_parent" android:layout_margin="5dp" /> </LinearLayout>
We need not have a real Android mobile to test this Android application. The Android emulator provides the facility to send test SMS messages to the deployed app via the DDMS Emulator Control as shown below.
Comments are closed for "Android Receive SMS Tutorial".
Great Tutorial. Works Fine.
Regards: itechvalley.in
thanks
how can I keep this app running in the background as u can c dat when u send an SMS when the app is closed it will not activate the app on its own.
wow nice bunch of tutorials
lucky to discover this site
This is a great tutorial for a practical use of a broadcast receiver, and having it update the UI.
provide a tutorial on file transfer using nfc and bluetooth and using internet ……….
How can use in fragment
Thank you , this only post that work for me !!
I tried above in Android Studio and despite having uses-permission READ_SMS in manifest file, i keep on getting
java.lang.SecurityException: Permission Denial: reading com.android.providers.telephony.SmsProvider uri content://sms/inbox from pid=6056, uid=10058 requires android.permission.READ_SMS, or grantUriPermission()
tell about the coding for sending and receiving sms by using internet..
How kill this BroadcastReceiver?
Hi this tutorial works but may i know how to open a link that is sent to us through this inbox from someone. I am having difficulty with it since its a list view.
can anybody help me to reply to the message by selecting each message
Thanks it works
The example seems to send okay, but receiving SMS messages seems to cause it to crash (and the default SMS app receives the incoming text). I assume this is due to the restrictions added in 4.4.
What steps would you need to take to make this app appear as a candidate to become the default SMS app?