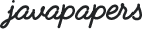
In this Android tutorial, I will walk you through to create an Android app to send SMS. It would be cool to have our own SMS application to send SMS messages.
There can be missing features in the SMS application that comes by default with our Android phone. We can use the example application here and build on top of it according to our needs.
Application is simple and easy to build. SmsManager
class takes care of sending the SMS message. We just need to get an instance of it and send the SMS message. We need to add permission to SEND_SMS
in the Android manifest file.
Just note the permission added to send sms in the below manifest file.
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.javapapers.androidsms"> <uses-permission android:name="android.permission.SEND_SMS" /> <application android:allowBackup="true" android:icon="@drawable/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme"> <activity android:name=".SendSmsActivity" android:label="@string/app_name"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> </application> </manifest>
This layout file has the mobile number to send SMS and the SMS message to be sent.
<LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:orientation="vertical" > <TextView android:id="@+id/toPhoneNumberTV" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Phone Number" /> <EditText android:id="@+id/toPhoneNumberET" android:layout_width="fill_parent" android:layout_height="wrap_content" android:inputType="phone"/> <TextView android:id="@+id/smsMessageTV" android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="SMS Message" /> <EditText android:id="@+id/smsMessageET" android:layout_width="fill_parent" android:layout_height="wrap_content" android:inputType="textMultiLine"/> <Button android:id="@+id/sendSMSBtn" android:layout_width="fill_parent" android:layout_height="wrap_content" android:text="Send SMS"/> </LinearLayout>
Here is the Android Activity class. Its just one thing, instantiate the SmsManager
class and send SMS using it.
package com.javapapers.androidsms; import android.app.Activity; import android.os.Bundle; import android.telephony.SmsManager; import android.view.View; import android.widget.Button; import android.widget.EditText; import android.widget.Toast; public class SendSmsActivity extends Activity { Button sendSMSBtn; EditText toPhoneNumberET; EditText smsMessageET; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_sms); sendSMSBtn = (Button) findViewById(R.id.sendSMSBtn); toPhoneNumberET = (EditText) findViewById(R.id.toPhoneNumberET); smsMessageET = (EditText) findViewById(R.id.smsMessageET); sendSMSBtn.setOnClickListener(new View.OnClickListener() { public void onClick(View view) { sendSMS(); } }); } protected void sendSMS() { String toPhoneNumber = toPhoneNumberET.getText().toString(); String smsMessage = smsMessageET.getText().toString(); try { SmsManager smsManager = SmsManager.getDefault(); smsManager.sendTextMessage(toPhoneNumber, null, smsMessage, null, null); Toast.makeText(getApplicationContext(), "SMS sent.", Toast.LENGTH_LONG).show(); } catch (Exception e) { Toast.makeText(getApplicationContext(), "Sending SMS failed.", Toast.LENGTH_LONG).show(); e.printStackTrace(); } } }
Comments are closed for "Android Send SMS Tutorial".
Dear Joe,
I find your continuous contribution on the internet of a great value. Since last time I wrote to you, we have closed the college, as we are now concentrating on making software, and we are doing great.
Now, how can we do something great for you? I have asked you before, and I would like if you can spend a little time thinking about how we can do something for you?
Warmly
David Svarrer
Dear Joe,
One thing, maybe… Try to click on paper.li, its an automated news paper, where you can link certain twitter accounts, Google+ accounts, facebook etc, and then it generates a news paper based on your chosen feeds.
That way, you can indeed create new stuff, in that you make the paper partially get content from sources you respect, and then your own sources. Then that publishes to those who are interested.
Its pretty hot, that paper – and free of charge.
Warmly
David
Thanks David. Sure I will give it a try and see if it helps.
[…] parse the SMS message out of it and update our UI. We will also show a toast message. In a previous Android tutorial to send SMS we created an app to send SMS messages. These two tutorials combined together will create one […]
[…] request, we will create a single app that is capable of sending and receiving SMS message. To send SMS messages refer the earlier tutorial’s code snippet. All I have done now is to create a single main […]
hi. thanks for the nice tutorial. but pls can u help out? I want to send a bulk SMS through http requests. do u have any idea on how I can implement it? thanks a lot
Hi, I am making an application where i need to send sms at a particular time of the day. I had set up all the time picker activities and all. But I am confused if I just compare the current system time and the set time, and then use the sendSMS class, would it be sent? Also, does the system needs to be in a certain condition for this to work?
Any help would be greatly appreciated.
Thanks
sir,I am very fond of learning new things as it is in programming, games or other activities but straight speaking i am more interested in making android apps i want to know why we use onclickListener if we can use onTouchListener can u sort out my problem