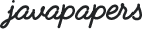
This Android tutorial will walk you through to create an alarm clock Android application. This alarm app is planned to be minimalistic and usable. It can set alarm for one occurrence for the coming day. You will get alarm ring sound, a notification message and a message in the app UI. This application and device can be idle or sleeping when the alarm triggers.
We will be using the AlarmManager
API to set and ring the alarm notification. We will have a TimePicker component and a toggle switch in the UI to set the alarm time.
Application is planned to be simple as possible and you can use this as a base framework and enhance it by adding fancy features.
We need to give uses-permission for WAKE_LOCK
, other than that the AndroidManifest.xml is pretty standard one. Just need to include the service and receiver.
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.javapapers.androidalarmclock"> <uses-permission android:name="android.permission.WAKE_LOCK" /> <application android:allowBackup="true" android:icon="@drawable/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme"> <activity android:name=".AlarmActivity" android:label="@string/app_name"> <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <service android:name=".AlarmService" android:enabled="true" /> <receiver android:name=".AlarmReceiver" /> </application> </manifest>
The Android Activity is designed to be simple. We have a TimePicker
component followed by a ToggleButton
. That’s it. Choose the time to set the alarm and toggle the switch to on. The alarm will work.
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" android:paddingBottom="@dimen/activity_vertical_margin" tools:context=".MyActivity"> <TimePicker android:layout_width="wrap_content" android:layout_height="wrap_content" android:id="@+id/alarmTimePicker" android:layout_alignParentTop="true" android:layout_centerHorizontal="true" /> <ToggleButton android:layout_width="wrap_content" android:layout_height="wrap_content" android:text="Alarm On/Off" android:id="@+id/alarmToggle" android:layout_centerHorizontal="true" android:layout_below="@+id/alarmTimePicker" android:onClick="onToggleClicked" /> <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:textAppearance="?android:attr/textAppearanceLarge" android:text="" android:id="@+id/alarmText" android:layout_alignParentBottom="true" android:layout_centerHorizontal="true" android:layout_marginTop="20dp" android:layout_below="@+id/alarmToggle" /> </RelativeLayout>
AlarmActivity uses the AlarmManager to set the alarm and send notification on alarm trigger.
package com.javapapers.androidalarmclock; import android.app.Activity; import android.app.AlarmManager; import android.app.PendingIntent; import android.content.Intent; import android.os.Bundle; import android.util.Log; import android.view.View; import android.widget.TextView; import android.widget.TimePicker; import android.widget.ToggleButton; import java.util.Calendar; public class AlarmActivity extends Activity { AlarmManager alarmManager; private PendingIntent pendingIntent; private TimePicker alarmTimePicker; private static AlarmActivity inst; private TextView alarmTextView; public static AlarmActivity instance() { return inst; } @Override public void onStart() { super.onStart(); inst = this; } @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_my); alarmTimePicker = (TimePicker) findViewById(R.id.alarmTimePicker); alarmTextView = (TextView) findViewById(R.id.alarmText); ToggleButton alarmToggle = (ToggleButton) findViewById(R.id.alarmToggle); alarmManager = (AlarmManager) getSystemService(ALARM_SERVICE); } public void onToggleClicked(View view) { if (((ToggleButton) view).isChecked()) { Log.d("MyActivity", "Alarm On"); Calendar calendar = Calendar.getInstance(); calendar.set(Calendar.HOUR_OF_DAY, alarmTimePicker.getCurrentHour()); calendar.set(Calendar.MINUTE, alarmTimePicker.getCurrentMinute()); Intent myIntent = new Intent(AlarmActivity.this, AlarmReceiver.class); pendingIntent = PendingIntent.getBroadcast(AlarmActivity.this, 0, myIntent, 0); alarmManager.set(AlarmManager.RTC, calendar.getTimeInMillis(), pendingIntent); } else { alarmManager.cancel(pendingIntent); setAlarmText(""); Log.d("MyActivity", "Alarm Off"); } } public void setAlarmText(String alarmText) { alarmTextView.setText(alarmText); } }
AlarmReceiver is a WakefulBroadcasReceiver
, this is the one that receives the alarm trigger on set time. From here we initiate different actions to notify the user as per our choice. I have given three type of notifications, first show a message to user in the activity UI, second play the alarm ringtone and third send an Android notification message. So this is the place to add enhancement for different types of user notifications.
package com.javapapers.androidalarmclock; import android.app.Activity; import android.content.ComponentName; import android.content.Context; import android.content.Intent; import android.media.Ringtone; import android.media.RingtoneManager; import android.net.Uri; import android.support.v4.content.WakefulBroadcastReceiver; public class AlarmReceiver extends WakefulBroadcastReceiver { @Override public void onReceive(final Context context, Intent intent) { //this will update the UI with message AlarmActivity inst = AlarmActivity.instance(); inst.setAlarmText("Alarm! Wake up! Wake up!"); //this will sound the alarm tone //this will sound the alarm once, if you wish to //raise alarm in loop continuously then use MediaPlayer and setLooping(true) Uri alarmUri = RingtoneManager.getDefaultUri(RingtoneManager.TYPE_ALARM); if (alarmUri == null) { alarmUri = RingtoneManager.getDefaultUri(RingtoneManager.TYPE_NOTIFICATION); } Ringtone ringtone = RingtoneManager.getRingtone(context, alarmUri); ringtone.play(); //this will send a notification message ComponentName comp = new ComponentName(context.getPackageName(), AlarmService.class.getName()); startWakefulService(context, (intent.setComponent(comp))); setResultCode(Activity.RESULT_OK); } }
The receiver will start the following IntentService
to send a standard notification to the user.
package com.javapapers.androidalarmclock; import android.app.IntentService; import android.app.NotificationManager; import android.app.PendingIntent; import android.content.Context; import android.content.Intent; import android.support.v4.app.NotificationCompat; import android.util.Log; public class AlarmService extends IntentService { private NotificationManager alarmNotificationManager; public AlarmService() { super("AlarmService"); } @Override public void onHandleIntent(Intent intent) { sendNotification("Wake Up! Wake Up!"); } private void sendNotification(String msg) { Log.d("AlarmService", "Preparing to send notification...: " + msg); alarmNotificationManager = (NotificationManager) this .getSystemService(Context.NOTIFICATION_SERVICE); PendingIntent contentIntent = PendingIntent.getActivity(this, 0, new Intent(this, AlarmActivity.class), 0); NotificationCompat.Builder alamNotificationBuilder = new NotificationCompat.Builder( this).setContentTitle("Alarm").setSmallIcon(R.drawable.ic_launcher) .setStyle(new NotificationCompat.BigTextStyle().bigText(msg)) .setContentText(msg); alamNotificationBuilder.setContentIntent(contentIntent); alarmNotificationManager.notify(1, alamNotificationBuilder.build()); Log.d("AlarmService", "Notification sent."); } }
Comments are closed for "Android Alarm Clock Tutorial".
Sorry 4 double-posting :(
I forgot to add the service in the manifest
now it works, but when the receiver recieve the event it throws a exception:
Unable to start receiver de.it2do.effektivlernen.AlarmReceiver: java.lang.SecurityException: Neither user 10043 nor current process has android.permission.WAKE_LOCK.
My Manifest.xml has the permission:
…
Can you help me? >.<
I keep getting a ‘noclassdeffound’ error. The exact line is on:
Intent myIntent = new Intent(AlarmActivity.this, AlarmReceiver.class);
AlarmReceiver.class is the one not found. Any fix for this?
how to make 5 alarm with 1 timepicker??
Once the alarm starts it never gets stopped. Switching the toggle button doesn’t cancel the sound, rather it turns into a loop and the ring repeats faster.
Why so?
This app contains lots of bugs. I don’t recommend people to follow this tutorial.
Can you show the use of media player to set the alarm tone in continuous loop.
I am having the same problem as Naif. This code does not include anything to turn off the alarm.
My code is Completely done..without error..But when I set the time the alarm does not work..The music also not sound
This program contains fully erors. . . :/
OMG…
Can’t run at all
Great job!I was able to get the application up and going within an hour, and this is my very first attempt to build an Android app. If anyone encounters any error(s), it’s more than likely due to syntax/setup related issues. Everything is here, couldn’t be any simplier. Thanks for posting, this was really helpful.
tanx a lot.
very good.
thanks! great work…
what is use for this code
public static AlarmActivity instance() {
return inst;
}
@Override
public void onStart() {
super.onStart();
inst = this;
}