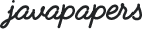
This is a Java puzzle to pep up our floating point arithmetic skills. Guess the output for the following Java puzzle program,
public class Precision { public static void main(String... args){ double d = 0.0d; for (int i = 0; i < 10; i++) { d = d + 0.1d; } System.out.println(d); } }
For the above Java program, we expect to get output as 1.0 and it will not happen.
Lets start from a basic fact, 0.1 + 0.1 is not equal to 0.2
A decimal number is represented in binary in runtime. 0.1 is a continuing number in binary. What is a continuing number? We know that in decimal system we cannot define 1/3 finitely. It keeps on continuing like,
0.333333333333333333333333333333...
Same like this, in binary representation 0.1 keeps on continuing. Even though 0.1 is a continuing number, there are only fixed number of bits available to represent it. To understand how many bits are available, we should understand IEEE-754. Java follows "ANSI/IEEE Standard 754-1985 (IEEE, New York)" for floating point operations. There are 53 bits available to represent in binary.
Lets now convert 0.1 to binary and round it off to 53 places,
0.0001100110011001100110011001100110011001100110011001101
So, the above number is what gets stored when we create a double 0.1
If we convert the above binary back to decimal we get,
0.1000000000000000055511151231257827021181583404541015625
which is slightly greater than 0.1 (where it all started). Eventually when we keep on adding 0.1 to it, the 'slightly greater' number swells and overflows. Because of overflow and rounding-off we get 0.9999999999999999 as output.
So what should we do to get the desired result. It is better to round-off at each step to desired number of digits as situation demands and then do the calculation. BigDecimal works as we are used to the decimal calculations and so it will be a better substitute. Even BigDecimal will falter on 'by third' calculations so keep an eye on it.
Comments are closed for "Java Puzzle: Floating Point Precision".
Very cool!
Thanks Ashwin.
[…] is to discuss about the numeric promotion that happens when using operators. Similar to the last Java puzzle on floating point precision, this article will also make raise some eyebrows. Last week a regular reader of Javapapers Palani […]
Thanks Joe,
I read Java puzzlers some time back , a lot of puzzles were there very interesting ones. Read it if you haven’t
Thanks
Pratik
Sure Pratik. I have read some from it long back. Thanks for the info.