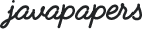
Buffer is a block of data that is to be written to a channel or just read from a channel. It is an object that holds data and acts as an endpoint in a NIO channel. Buffer provides a formal mechanism to access data and tracks the read and write processes.
Buffer is one of the main differences between the old Java I/O and the NIO. Previously data is read directly from a stream or written directly into it. Now the data is read from a buffer or written into it. Channels are synonymous to streams in the NIO. To know more about NIO channel, please read the previous tutorial Java NIO Channel.
There is a buffer type for each primitive type. All the buffer classes implement the Buffer
interface. The most used buffer type is ByteBuffer
. Following are the buffer types available in Java NIO package.
Buffer is a fixed size type, we can store only a maximum of a “fixed amount” of data. That maximum fixed size is called capacity of the buffer. Once the buffer is full it should be cleared before writing to it. Once a capacity is set it never changes in its lifetime.
On write mode, limit is equal to the capacity of the buffer. On read mode, limit is one past the last filled index of the buffer. When the buffer is being written, the limit keeps incrementing. Limit of a buffer is always greater than or equal to zero and less than or equal to capacity. 0 <= limit <= capacity
.
Position points to the current location in buffer. When the buffer is created, position is set to zero. On write or read, the position is incremented to next index. Position is always set between zero and the limit of the buffer.
Mark is like setting a bookmark of the position in a buffer. When we mark() is called the current position is recorded and when reset() is called the marked position is restored.
flip() method is used to prepare a buffer for get operation or makes it ready for a new sequence of write. flip() sets the limit to the current position and then position to zero.
clear() method is used to prepare a buffer for put operation or makes it ready for new sequence of read. clear() sets the limit to the capacity and position to zero.
rewind() method is used to read again the data that it already contains. rewind() sets the buffer position to zero.
allocate(size)
and it returns a Buffer instance.
ByteBuffer byteBuffer = ByteBuffer.allocate(512);
byteBuffer.flip();
int numberOfBytes = fileChannel.read(byteBuffer);
char c = (char)byteBuffer.get();
ByteBuffer byteBuffer = ByteBuffer.allocate(512);//512 becomes the capacity
byteBuffer.put((byte) 0xff);
Above two are just example cases to read/write from a buffer. There are different types of Buffers available and variety of methods to read/write. You should choose them according to your use case.
Following is an example that demonstrates both read and write operations using buffer.
package com.javapapers.java.nio; import java.io.IOException; import java.nio.ByteBuffer; import java.nio.channels.FileChannel; import java.nio.file.Path; import java.nio.file.Paths; import java.nio.file.StandardOpenOption; public class BufferExample { public static void main(String[] args) throws IOException { Path path = Paths.get("temp.txt"); write(path); read(path); } private static void write(Path path) throws IOException { String input = "NIO Buffer Hello World!"; byte[] inputBytes = input.getBytes(); ByteBuffer byteBuffer = ByteBuffer.wrap(inputBytes); FileChannel channelWrite = FileChannel.open(path, StandardOpenOption.CREATE, StandardOpenOption.WRITE); channelWrite.write(byteBuffer); channelWrite.close(); } private static void read(Path path) throws IOException { FileChannel channelRead = FileChannel.open(path); ByteBuffer byteBuffer = ByteBuffer.allocate(512); channelRead.read(byteBuffer); byte[] byteArray = byteBuffer.array(); String fileContent = new String(byteArray).trim(); System.out.println("File Content: " + fileContent); channelRead.close(); } }
Comments are closed for "Java NIO Buffer".
[…] Java NIO Buffer […]