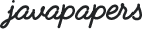
This is a Java puzzle involving the Collection List interface. This is a Java beginner level puzzle and will help understand how the size works in List element. What is the output for the following Java code snippet?
package com.javapapers.java.puzzle; import java.util.ArrayList; import java.util.Arrays; import java.util.List; public class ListPuzzle { public static void main(String args[]) { List<String> circus1 = new ArrayList<String>(); circus1.add("Monkey"); circus1.add("Elephant"); List<String> zoo1 = new ArrayList<String>(circus1); zoo1.add("Lion"); System.out.println("zoo1 size: " + zoo1.size()); String[] circus2 = { "Monkey", "Elephant" }; List<String> zoo2 = Arrays.asList(circus2); zoo2.add("Tiger"); System.out.println("zoo2 size: " + zoo2.size()); } }
In this Java program we are creating two different List instances zoo1 and zoo2. zoo1 is created using the ArrayList constructor which allows to pass a collection. zoo2 is created using the utility class Arrays which is used for manipulating arrays. There is no issue in the zoo1 instantiation and addition of an element to it.
The problem is on line where we add an element to the zoo2 object. zoo2 instance is a fixed size List instance. The method asList() of Arrays returns a fixed size List instance. This instance serves as a bridge for an array to a collection. We cannot add a new element or remove an element from the returned instance. We can set the value of an already existing element using its index. Any change we do will be propagated to the array source.
So we will get UnSupportedOperationException at the line where we add an element to zoo2. This is one of the popular errors faced a by a beginner Java programmer.
zoo1 size: 3 Exception in thread "main" java.lang.UnsupportedOperationException at java.util.AbstractList.add(AbstractList.java:148) at java.util.AbstractList.add(AbstractList.java:108) at com.javapapers.java.puzzle.ListPuzzle.main(ListPuzzle.java:20)
Comments are closed for "Java List size Puzzle".
Why below snipet will not compile.
int array[]={3,5,7,8};
List lArray=Arrays.asList(array);
Its says type mismatch
The above code will work but if you verify the size of the list it will be 1 because it considers entire int(primitive) array as one object. If you change the same code above to wrapper (INTEGER) array the size of the list will be 4. This means when you are converting an array to list it should be an array of wrapper class. Hope this explanation helped you..
[…] ArrayBlockingQueue is based on a bounded Java array. Bounded means it will have a fixed size, once created it cannot be resized. This fixed size collection can be understood using this Java List size puzzle. […]
Very nice article for beginners like me! and the pgm explains the concept very well! Thanks Joe.