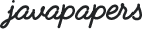
BlockingQueue Collection is a type of Java Queue. BlockingQueue is part of Java concurrent util package. It is best used in multi threading and producer-consumer scenarios. While adding an element to a a BlockingQueue, if there is no space it can wait till it becomes available. Similarly while retrieving, it will wait till an element is available if it is empty.
Throws exception | Returns value | Blocks | Timed | |
---|---|---|---|---|
Insert | add(e) | offer(e) | put(e) | offer(e, time, unit) |
Remove | remove() | poll() | take() | poll(time, unit) |
Examine | element() | peek() | not applicable | not applicable |
There are four types of methods available for each operations like insert, remove and examine in a BlockingQueue. The respective type of method has to be chosen according to our use case requirement.
These methods are used in a multi-threaded scenario. BlockingQueue extends the Collection interface and so an implementation of a BlockingQueue will contain the standard collection methods as well. For example removing an element can be done using remove(index). BlockingQueue is always best used in a threaded scenario.
All these methods are guaranteed to be thread-safe. Other standard methods that are part of the collection may or may not be thread-safe and the choice is left to the implementation.
BlockingQueue is an interface. Either we should go for custom implementation or choose the existing implementations from the Java JDK. Following the different implementations available for the BlockingQueue in Java.
Following posts will have examples on implementing BlockingQueue using the above JDK BlockingQueue implementations and a custom implementation for BlockingQueue as well.
Comments are closed for "Java BlockingQueue".
Hi,
did I understand it right that all those 12 above are thread-safe and, for example, addAll(..) is not?
It all depends on the implementation. BlockingQueue is an interface.
As per the spec, those 10 methods are to be thread-safe. Other Collection methods like addAll(..) is left to the implementer, its his choice to make it thread-safe or not.
So we should check the implementing class to know about this detail.
[…] tutorial is to learn about the collection LinkedBlockingQueue which is an implementation of Java BlockingQueue. LinkedBlockingQueue order elements first-in-first-out (FIFO). With respect to bounds of the […]
[…] PriorityBlockingQueue is a concurrent collection and an implementation of BlockingQueue. PriorityBlockingQueue is an unbounded collection. Ordering of elements in the […]
[…] tutorial is to learn about the concurrent collection SynchronousQueue. It is an implementation of BlockingQueue. Among all Java concurrent collections, SynchronousQueue is different. Capacity of a synchrounous […]
[…] BlockingQueue is a queue that supports blocking operations for that will wait for the queue to become non-empty when retrieving element from it and wait for space to become available when inserting elements. […]