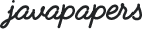
First lets understand what does “java import” does to your java program!
Consider the java import statements:
1) import package.ClassA;
2) import package.*;
Java statement (1) gives you a license to use ClassA inside the whole program without the package reference. That is you can use like ClassA obj = new ClassA(); or ClassA.getStatic(); Java statement (2) allows you to use all the java classes that belong the imported package in the above manner. That is without the package reference.
If you don’t use import statement, you can still use the classes of that package. But you should invoke it with package reference whereever you use.
That is like, package.ClassA obj = new package.ClassA(); – looks very ugly isn’t it?
Now coming to the static import part. Like the above ugly line we have been unknowingly using (abusing) the java static feature.
Consider the java example: double r = Math.cos(Math.PI * theta);
How about writing the same java code like: double r = cos(PI * theta); – looks more readable right?
This is where static import in java comes to help you.
import static java.lang.Math.PI;
import static java.lang.Math.cos;
Do the above static imports and then you can write it in the more readable way!
The normal import declaration imports classes from packages, so that they can be used without package reference. Similarly the static import declaration imports static members from classes and allowing them to be used without class reference.
Now, we have got an excellent java feature from java 1.5. Ok now we shall see how we can abuse this!
like, import static java.lang.Math.*; – yes it is allowed! Similarly you do for class import.
Please don’t use this feature, because over a period you may not understand which static method or static attribute belongs to which class inside the java program. The program may become unreadable.
General guidelines to use static java import:
1) Use it to declare local copies of java constants
2) When you require frequent access to static members from one or two java classes
Comments are closed for "Java Static Import".
Interesting , I never knew that you can import a static method in java. Looks cool. But to me , it would be easy to read / understand if we associate the class name. i.e Math.cos rather calling cos()
Yes that is right. Avoid using java static import in general straight forward cases.
But consider using the java static import in following two scenarios:
1) When you have to only one or max two static imports in a class many number(say atleast 20) of times.
2) Constant Interface Antipattern
Example for above case 2):
When you create a interface with constants and extend it to use (note below ‘PI’ referring the constant). In this scenario, better use the bottom code.
public interface MyConstant {
public static final double PI = 3.14159;
}
public class MyMath implements MyConstant {
public double myComplexMathMethod() {
return (PI * 100);
}
}
————–
public final class MyConstant {
private MyConstant() {}
public static final double PI = 3.14159;
}
import static MyConstant.PI;
public class MyMath {
public double myComplexMathMethod() {
return (PI * 100);
}
}
http://en.wikipedia.org/wiki/Constant_interface – has good information related to the topic.
Hi,
I happened to see your post on “Static import in Java” and find it quite informative.
Thanks for sharing valuable knowmledge.
Bizz..
Hi,
This information on java static import is very good..
Thanx for explaining in such a simple manner.
—– krunal shah
Static import in java is new for me. I have never heard about it. Thanks man.
Gr8 work………Thanks a lot
very nice
Thanx a lot. Very informative keep on
Hi this is best answer we get on java related.
So keep trying to work good,better and best.
there is an interesting thing, that you have not mentioned,
what is the difference between:
1.
————————
import java.io.*;
class main
{
public static void main(String[] args)
{
FileWriter fw = null;
}
}
————————
2.
————————
import java.io.FileWriter;
class main
{
public static void main(String[] args)
{
FileWriter fw = null;
}
}
————————
most programmers don’t like to use the second version, which doesn’t waste resources, and cut wasted times, also .. the second option might be the only option, when you have either to use a private static member, or when the class is private !
thank you …
very good explanation about static import.I came across this site while searching for this topic in google.It is very interesting
good explanation
very good explanation
Detailed explanation. Thanks.
nice answer….
thnx..
i dont understand
why do we want to declare a final static variable in an interface and then static import t into another class
cant we directly declare a static final variable in the class itself and use it in the class…
less import hassles..
Quite informative
I do not fully understand: when you import static you import the static fields and methods from a package AND the classes in that package, or you import ONLY the static stuff?
Could someone answer to this, please?
Hi,
how static import is useful to achieve real time concepts means on real time objects…
Very nice!!
Previously I had a doubt about how does java inbuilt “System” class uses the Object Reference “out” of PrintStream class and “in” of InputStream class without using import statement. Now your post answers it. Thanks alot.
its all new features in java for me. keep up the good wor
Very Good Information..Thanks for sharing
may I request you to add your profile link – ‘About me’ in menu list that gives quick access to read about your profile as you are helping lot so readers must be interested to look at your profile…
Thanks!
excellent!!
I liked your way of writing..to the point!!
Thank you..
Can Static import will import static nested classes or nested enums or nested interfaces into our class????????
Good Explanation…
Thanks for it
Hey dude,
The code you have given:
———————————-
public final class MyConstant {
private MyConstant() {}
public static final double PI = 3.14159;
}
import static MyConstant.PI;
public class MyMath {
public double myComplexMathMethod() {
return (PI * 100);
}
}
————–
This doesnt work:
It gives error as unable to find Symbol when I run MyMath :-(
in java which statement come first?import statement or static import statement.
Excellent write up.. A bunch of thanks….
Nice explanation…
Good explaination…
Hi,
I wanted to ask if using import copies and pastes the content of the entire class in my file, just like in c
Thanks
i like your way of writing
very clear idea..thanks
Very Good explaination…….
Nice to know about static import.
I didn’t know this. it can be so useful if used sensibly. Nice article.
Thanks for the notes. You have explained the concept in a good way.
Thank you for sharing.Clean explanation.
good explanation
Very useful Blog…………
good
very usefull………..
useful blog…..
Thanx very much…….
I have read many java books/articles/material but java static import is something I learned first time, as they mentioned the importance , scenarios and in very simple language.
I have read many java books/articles/material but java static import is something I learned first time, as they mentioned the importance , scenarios and in very simple language.
I have read many java books/articles/material but java static import is something I learned first time, as they mentioned the importance , scenarios and in very simple language.
Hi Joe;
While commenting here it dnt shows the successful insertion of comment and thus I tried few times and when reload the page it shows four times comments insertion.
Please look after this.
:Abhiroop
Hi Joe
As usual, nice and simple explanation.
Great Job.
-Prasad
good explanation.
Easy word and easy to learn.
thanks….
nice one, thanks
Nice explanation….. thanks a lot… really…..
I know Core Java So good. but i want to learn a lot about it..
So can anyone suggest me a very very difficult book over core java…..?
Thanks for explaining in very simple way :)
Nice way of explaining about static import with examples to know when and where we have to use. :)
Sir,
I’ve one dought, why we need not create object for static method ?
Sir,
I’ve one dought, why we need not create object for static method ?
Keep to good work going Joe! I find your blog informative and like the way you write.
Cheers,
Bharat Sharma
Very Good Explaination!!!!!!!!
i have bumped into a treasure of a JAVA BLOG!!
Thanks Joe :)
Hi,
Nice post
Thanks you
Nice, Thanks
The way you have explained makes one never forget the topic. Thanks Joe.
nice presentation till now i didn’t heard about this static import yes i agree with you about ofter period of time we cant trace the application if using many static import in a single class
thanks
Awesome.
Good Explanation…………!!!
Thanks for it… :)
that’s really helpful.
Excellent
Thank you Good Explanation
Is static import supports only java.lang.Math package
this is good tutorial for me,
tank you joe
No , you can import static contents of any class.
Awesome.. Thank u very much
HI Joe,
reading in all posts and sites the static clause as part of import statement, we may use it in code as below
“static import something.*”
This is syntactically wrong.
I am not telling to correct your post, but feed in the thought to all those who read, its always
” import static ” in java.
Thanks for explanation…
i dont think so joe is wrong.this syntax is
correct.
import static java.lang.Math.PI;
double j = PI*100;
System.out.println(“printvalue”+j);
it is working..
Thanks for simplied explanation
This post is very enlightening.Thank you sir.
what is static memebers.can u plz briefly
I did not get this:
“the second option might be the only option, when you have either to use a private static member, or when the class is private !”
How can we import private classes?? Never heard of that. Also I think we can not import private static members. Please give an example if I am wrong.
Static members are members of a class, not an object. They are always available, and only one copy of a static member exists for any given class. So, if you define a class as follows:
public class MyClass {
public static final String STATIC_CONSTANT = “somevalue”;
}
The variable ‘STATIC_CONSTANT’ is available through the class, but not through objects of the class. You would access it outside of the class by using:
MyClass.STATIC_CONSTANT
And, static values are accessible without an instance of the class having been created.
Those are two separate classes and should be within their own files.
One file, which should be named MyConstant.java, is:
public final class MyConstant {
private MyConstant() {}
public static final double PI = 3.14159;
}
The other file, which should be named MyMath.java, is:
import static MyConstant.PI;
public class MyMath {
public double myComplexMathMethod() {
return (PI * 100);
}
}
It works just fine.
thnx joe! it is really useful for the programmer like me, expecting lot more so simple explanations from your end.
Thanx joe.
Thanks Joe, explained in a easier manner :)
Today during a discussion the Static import concept came up. I was curious and googled and see what I got this article at 2nd number in search list and this was so helpful and easy to understand. Thanks Joe.
Thanks for sharing valuable knowledge…
again you rock joe . I am big fan of your blog because you make very simple and easy approach to learn the most complected issues of java . Go ahead Joe and keep on posting new blogs. God Bless
very nice . explained beautifully thanks :)
Nice and simple explanation