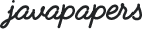
Overloading and overriding are completely different. Only the notion about interface (function) name is same. Overloading is the ability to use same interface name but with different arguments. Purpose of functions might be same but the way they work will differ based on the argument types. Overriding is applicable in the context of inheritance. When there is a need to change the behavior of a particular inherited method, it will be overridden in the sub-class.
Overloading itself is not polymorphism and similarly run-time binding itself is not polymorphism. All these and more are used to exercise the object oriented property polymorphism.
Let’s discuss overloading in detail. In websites, I generally see a overloaded truck as example for overloading. It symbolizes that, adding more attributes, methods to a class and making it look bulkier is overloading. Please get it right, in fact when we use overloading for the outsiders view the class will look compact.
I have shown two images, first one is called Harmonium. Its a classical music instrument from India and its a primitive version of piano. The other is a modern digital keyboard. In our overloading context, Harmonium is before overloading and keyboard is after overloading.
This is an attempt to closely mimic overloading :-)
public class OverloadExample { public static void main(String args[]) { System.out.println(playMusic("C sharp","D sharp")); System.out.println(playMusic("C","D flat","E flat")); } public static String playMusic(String c, String d) { return c+d; } public static String playMusic(String c, String d, String e){ return c+d+e; } }
package com.javapapers.java; public class NullArgumentOverloading { public static void main(String[] args) { NullArgumentOverloading obj = new NullArgumentOverloading(); obj.overLoad(null); } private void overLoad(Object o) { System.out.println("Object o argument method."); } private void overLoad(double[] dArray) { System.out.println("double array argument method."); } }
Can you guess the output?
When a class is inherited we get the base class’ methods and attributes based on the respective access modifiers. A specific inherited method’s behavior may not be suitable in the sub-class. So we replace the inherited method’s behavior as required. After overriding, the old behavior is completely obsolete.
Look at this monster truck, the old small four wheels are replaced with huge wheels to suit the need. This is overriding in real world.
Implicitly every object in Java is extended from Object class. Object has a method named equals. In String class the default behavior is overridden. The String equals behavior is modified to check if the character sequence is same as the compared one and returns true.
Comments are closed for "Overloading vs Overriding in Java".
Nice comprehensive information sir….Thanks.
Nice example to understand the concepts easily Thanks
Nicest example that I have ever seen or think of for these blury concepts “overloading” and “overriding” in my 12 years exposure to java. Your simple way of explaining is superb! Hearty thanks & wishes!!
very very thank u sir …very nice explanation sir
thanks a lot for explanation.. only now I understand clearly the difference between overloading and overriding
This information is superb.Thanx alot.I understand this topic very easily by those examples. the summary of key points on how Java implements overloading and overriding is superb. Thanq
A nice way to explain OL and OR.
Awesome…
Nice Observation on how Java does overriding. Thanks.
Great explanation
wow…simply superb
thnx sir it is helpful for understanding overloading and overriding.
thanks
overriding is explained well, overloading concept is also good but the use of word “interface” is quite confusing….
(it is Just Good For Beginers)..
And…. It is not Sufficient…for advanced experts. overloading puzzle is good.
Give Two three Programatical Examples…
Can you give a code example for over riding like over loading….?
Overloading simplest example
public class OverLoad
{
void sum(int x, int y)
{
int sum=x+y;
System.out.println(“sum is: “+sum);
}
void sum(int x, int y, int z)
{
int sum=x+y+z;
System.out.println(“sum is: “+sum);
}
public static void main(String arg[])
{
OverLoad ol=new OverLoad();
ol.sum(5,4);
ol.sum(5,4,3);
}
}
o/p-> sum is: 9
sum is: 12
Overriding simplest example
class A
{
void calculate(int x, int y)
{
int sum=x+y;
System.out.println(“sum is: “+sum);
}
}
public class B extends A
{
void calculate(int x, int y)
{
int product=x*y;
System.out.println(“product is: “+product);
}
public static void main(String arg[])
{
B b=new B();
b.calculate(5,4);
}
}
Run this code o/p will be 20
class A
{
void calculate(int x, int y)
{
int sum=x+y;
System.out.println(“sum is: “+sum);
}
}
public class B extends A
{
/*void calculate(int x, int y)
{
int product=x*y;
System.out.println(“product is: “+product);
}*/
public static void main(String arg[])
{
B b=new B();
b.calculate(5,4);
}
}
run this code o/p will be 9
now i think u are clear… if still not. leave the comment
your concept is very clear please send me some concept and example in my email related to applet,exception ,thread… Thanks
Joseph , I could not digest “Run time binding is not polymorphism”.But I believe it is otherwise. Overloading is static binding since at compile time it is bind,And also overloading is not polymorphism”, whereas overriding is polymorphism since it is binded at runtime.
Clear the confusion
overloadin=it is the relation biw the method declare in supper class and subclass having thr same method name name diffirent no input and diffirent type of input–;;; for eg
public void m1()// no argument constructer
public void m1(int i)// argumenter contructer
public void m1(inti,String s)// this is all about the method overloading
Nice Explanation for Overriding. The truck example for overriding is cool.
Dear Joseph, this is the very simple and easy to understand article.so we are thank to you………………
If example is given for overriding then it would be possible to understand more clearly….
example is given above in my comments
Is it true that Overloading is not a polymorphism?
this overloading and overriding concept is very clearly explained,and before reading this topic i didn’t understand these two task difference,now am understand completely. thank to you
nice sir…
Hi joe!
So gud!
All your answers are awesome relating to realtime easy understanding thank you for sharing your knowlegde. can you please explain java polymorphism it hasnt been very clear to me all time although partially i understand still not clear.
thanks
raj
Nice fundamental introduction on overloading and overriding. A deep analysis would be appreciated.
Hi,
In one of the interview I got this question:
Consider this example:
class P {
public void abc() {
}
Now class C inherits class P
class C extends class P{
public void abc() {
// adds some additional functionality;
}
Now, here is the question:
Due to some reasons if you want the functionality of abc method of class P (i.e parents )in child’s class, then how we can achieve this?. Is it through key word super?
I understand this is very strange, but it was asked in interview
Thank u,
Give an example when you can not overload a method in Java.
excellent Java tutorial and simple thanks
wow simply super…..great explanation .thank u
hi its really useful 4 me to understand tanqu…
Consider this example:
class P {
public void abc() {
}
Now class C inherits class P
class C extends class P{
public void abc() {
// adds some additional functionality;
}
Now, here is the question:
Due to some reasons if you want the functionality of abc method of class P (i.e parents )in child’s class, then how we can achieve this?. Is it through key word super?
I did not understand the question here..
Can’t we do like this –
P sup = new P();
P sub = new C();
sup.abc(); //Calls super class method
sub.abc(); //Calls sub class method
Please let me know if its not correct..
Thanks,
Deepesh
thnx sir it is helpful for understanding all concepts. Iam a beginner,I want to download all your core concepts for java, How Can i download, please send to my mail.
Thanxs a lot, great job!!!
Thanks for explaining in a simple language.
Good Work :)
hi jo..thank you so much…its very useful website…
Karunakar
There are times when your article is complete and one requries no more reading.
this is NOT one of them. Please try to put some examples and rules/protocol associated.
eg: you didnt explain the access modifiers / scope in over-riding
eg: return type in overloading
gud effort nevertheless…
Thankyou so much..!
Never read a better article elsewhere. Simple description and I will never forget overloading and overriding hereafter.
excellent !
can u give some more example in c++
Shilpi
Amazing way of drilling the concept of Overloading and Overriding into the head.
Thanks Joe
can return type differ in overloading and overriding?
Nice one, Thanks…..
nice explanation but little bit confusion can u please explain it clearly…….
Really good and practical
best tutorial
@Nitin:Good one…:-)
very nice example with real world…:)
Thank you sir :) i got to know the concept
can you override the static.
i really thanks to you very much ……i understood both overloading and overriding concept very well.
Nitin given this example to explain overriding but i have a doubt in this below example that is “What is the use of extending A class in B?” without extending that class also we will get the same result na…
Overriding simplest example
class A
{
void calculate(int x, int y)
{
int sum=x+y;
System.out.println(“sum is: “+sum);
}
}
public class B extends A
{
void calculate(int x, int y)
{
int product=x*y;
System.out.println(“product is: “+product);
}
public static void main(String arg[])
{
B b=new B();
b.calculate(5,4);
}
}
Run this code o/p will be 20
Overriding simplest example
class A
{
void calculate(int x, int y)
{
int sum=x+y;
System.out.println(“sum is: “+sum);
}
}
public class B extends A
{
void calculate(int x, int y)
{
int product=x*y;
System.out.println(“product is: “+product);
}
public static void main(String arg[])
{
B b=new B();
b.calculate(5,4);
}
}
Run this code o/p will be 20
Superb its very useful thing which u have explained in detail with a very gud examples …Thanks a lot
Thank you sir : )
I like your way of explanation.
Its good and easy to understand.
Thank you for this nice explanations!
Good one.
hi joe i have seen couple of your articles which were very intereseting and simple … thanks for sharing your knowledge.
nice answer
thank you sir
very nice article
There is any concept of consructor overriding?
very nice explanation sir….
u have explained it very well….
thanks for giving a clear view in real time application….
Nitin given this example to explain overriding but i(raju) have a doubt in this below example that is “What is the use of extending A class in B?” without extending that class also we will get the same result na… (raju……)
Overriding simplest example
class A
{
void calculate(int x, int y)
{
int sum=x+y;
System.out.println(“sum is: “+sum);
}
}
public class B extends A
{
void calculate(int x, int y)
{
int product=x*y;
System.out.println(“product is: “+product);
}
public static void main(String arg[])
{
B b=new B();
b.calculate(5,4);
}
}
Run this code o/p will be 20
Raju on April 26th, 2012 10:53 pm
——————————–
Raju, without extending class A also possible, but if u want to access method(s) in class A, u need to create object for class A seperately.
If we write class B extends A
there is no need of creating objects for both classes. simply we can create object for class B and also access method(s) in class A also. That is behaviour of inheritence.
class to class – extends
interface to interface – extends
class to interface- implements
good explanations… it helps to learn easily. thank you to everyone.
Nice explanation!
good
good Example Sir
thanks
hii,
these concepts r too easy to understand
ok sir, i understood your concept but i want
to get run time input with super key word code with explanation.
thnx i understand this very easily………
Thanks…
It’s my pleasure…
Simple and more understandable..
[…] have two classes B and C inheriting from A. Assume that B and C are overriding an inherited method and they provide their own implementation. Now D inherits from both B and C […]
hai sir this is good i able to understood this and thank u so much
thnk u so much sir
An Excellent explanation and helps a lot to the person who is new to java….very good explanation to all the articles…I am following the posts regularly….Thank you so much….
Excellent !.
Wonderful
Excellent explanation thank you sir.and i have one doubt what is static overloading and dynamic overloading .give me one example
best comparison i have evry found on net is this one….. cleared all my doubts. thx for this. (first time in my life i am writting a comment on some blog in last 7 years)
great explanation.. Helped me understand the terms better. Thank u..
nice example with explain…
very good nitin
nice example……it’s easy to understand explanation
I clear my concept about overriding & overloading but I want practical example on both where I can programiticaly use it.
Simply Super
Very good example about both.
Thanks
Raj Chaudhary
Statement : Derived classes still can override the overloaded methods. Compiler will not binding the method calls since it is overloaded, because it might be overridden now or in the future.
Can anyone help me understand this statement?
Hello sir your way of explanation is very good.
Thank you very much sir.
the method is good for the st udents but to put briefly in the libriry system.
hi,
joe am a bigneer i need your help please let me know were do i get good understandable programs to practice and simultaneously complete java topics to learn.reply me as soon mas possible urgent……………………….
thank you sir,
it is very helpful me
NICE
the best example i’ve seen… thanks a lot sir.
simply superb…
its very interesting and easy to understand…Thanks a lot…
great sir…..thank u…
[…] to the standard console and a newline. There are multiple println methods with different arguments (overloading). Every println makes a call to print method and adds a newline. print calls write() and the story […]
very very very niceee………………
[…] use it in all your classes. The class which you want to be cloned should implement clone method and overwrite it. It should provide its own meaning for copy or to the least it should invoke the super.clone(). […]
The example of harmonium and piano has explained the term Overloading in awesome way…
Cant forget this term ever in my life from now…
the example explained is awesome, cant ever forget this term in my life.
very very nice example
by
devaraj.k
i have one question regarding overloading, can we achieve overloading in case of inheritance.
As explained in below example is overloading is achieved in class B.
class A
{
public void test(int i)
{
System.out.println(“From class A test method”);
}
}
class B extends A
{
public void test(int i,int j)
{
System.out.println(“From class B method”);
}
public static void main(String args[])
{
}
}
nice
thank u sir
[…] and write a custom excel view. There is a method buildExcelDocument in this class, which we should override to build the […]
Here in this context, I have used the word interface to express a Java method signature. Surely this is not Java interface class.
I am expressing that those words cannot be used interchangeably. When we say polymorphism, it is a whole larger concept comprising of all these things.
Yes it can be overloaded and no issues. But, the method with single String-array argument will be invoked as always.
in the puzzle given in your tutorial, obj.overload(null) is calling double array method. wanted to know why the object o method is not called? because even object o can be null.
We dnt need 2 define variable
Hi Joe,
Thanks for the information because There will be always confusion about the overriding and overloading. It is good that you have explained well.
Thanks,
u r god to java developers
simply create an object to child class and call the super class method
hi joe,
it would be better if you use a good example than this.
Brother super explanation hats off bro.
Hi Joe,
Could you please explain why “double array argument method” is called in the puzzle? Thanks in advance.
I have a doubt. Every teacher call method overloading is a static polymorphism. But every method is binded with the object at the runtme. Because object created at run time. Then how it is static?
Thanks a lot. Nice, clear, confusion-free explanation. While trying that code snippet of overloading with null object. it is showing errors in method definition. Help plz, anyone interested in discussing on that
superb!thank you for giving the clear example .