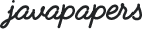
In this article, I will explain what a closure is and clear the confusion surrounding anonymous inner classes and closures, then java’s current situation with respect to closures.
First I want to emphasize the below two points:
Function types and inline function-valued expression are called closures. Let me decrypt this definition for you. An anonymous function that contains some context surrounding it as a snapshot and can be passed as a parameter. This closure defintion has two parts. First one is about callback. That is, a pointer to a function that can be passed as a parameter. Second part of the definition is, this callback function will enclose some contextual information surrounding it as a snapshot and passed along with the function.
A closure is also referred to as a ‘first class object’ which can refer to attributes from its enclosing scope. As defined by Christopher Strachey in ‘Understanding Programming Languages’, a first class object can be stored in a data structure, passed as a parameter, can be returned from a function, can be constructed at runtime and independent of any identity.
In case if you are curious in Mathematics a closure is, when you operate on a members fo a set and if the resultant is always a member of that set then its called a closure.
Anonymous classes in java are close to being called as a closure. They don’t 100% support the definition but come close to it and thats why we see lot of literature calling anonymous inner classes as closure. Why do I say its not 100%? An anonymous inner class can access “only” the final local variable of the enclosing method. It is because of this restriction, anonymous inner class in java is not a closure.
If you remember the memory management in java, you can recall that the local variables are stored in a stack. These java stacks are created when the method starts and destroyed when it returns. Unlike local variables, final fields are stored in method area which lives longer even after the return of the method. If we want to make anonymous inner class as a closure, then we should allow it to access all the fields surrounding it. But, as per the current memory management, they will be destroyed and will not be accessible after the method has returned.
In that case will we get closure in java in future? We have a specification written by Peter Ahe, James Gosling, Neal Gafter and Gilad Bracha on closures for java. It gives detailed description of how a closure can be implemented in java and example code on how to use them. We have JSR 335 for closures in java named as Lambda Expressions for the Java Programming Language.
Comments are closed for "Java Closures".
Wow! Neatly explained. Thanks a lot! Would have been more clear if any example was provided.
Whether Java’s anonymous inner classes are closures does not depend on an arbitrary chosen definition from a particular mathematical model of programming.
They are in fact closures, even if they are not captured as variables of a first-class type (yet). They do implement all the functionality to qualify as a closure! Just like you said – they do save the context (of final variables). Any variable in this context can be captures beforehand as a final one: T finalFoo = (T)nonFinalFoo;
However, they are not lambda-functions! (a.k.a. lambda-expressions) Anonymous inner class fail to have any free variables. That is, all their variables are captured at definition time; instead of capturing the context itself at definition time and evaluating them at runtime.
An example of lambda-function grade closure is are JavaScript functions!
The this variable always refers to the executing object context. (And can be also set to an explicit one.)
x = 5;
function log() {alert(this.x);}
log();
var bar = {x: 2, f: log};
bar.f();
what is the use of clusters why we are using clusters.do u have any any that can u reply discuss me
Great Explanation. Cleared my confusions.
[…] brace is creation of an anonymous inner class. Without considering the second brace if you see the first brace alone then its nothing new for us. […]
In the definition of the closure given in the article… what does it mean to be “independent of any identity.” ?
Hi Joe,
Loved this blog. But I am still confused as to why scoped inner classes can only access final variable of enclosing method.
You mention that “Unlike local variables, final fields are stored in method area which lives longer even after the return of the method”. Can you elaborate on this? How is storing in method area help inner classes access them while storing in stack cannot ? I went through your memory management blog but did not find an answer. Would love to hear your thoughts on this ?
thanks for info its very useful
what I understand is : java.io.InputStreamReader(InputStream)
java.io.OutputStreamWriter(OutputStream) implements Decorator Pattern, please comment!
[…] for lambda expressions. They are part of Java Specification Request (JSR 335). I wrote about closures in Java two years back. Lambda expression are informally called as closures. We are closer to the release […]
hi Thanks for this tutorial,
Can u please tell me why Anonymous inner class are not closure but Lambda expression is closure .Because both can access only final variable of the enclosing method.please share me some Example to differentiate.
thanks,
Roushan