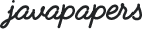
A hot topic in Java 8 is support for lambda expressions. They are part of Java Specification Request (JSR 335). I wrote about closures in Java two years back. Lambda expression are informally called as closures. We are closer to the release of Java 8 and lets see how it is implemented.
Lambda expressions are similar to methods, it has arguments, a body and return type. They can also be called as anonymous methods. A method without name.
One of the main features of lambda expressions is it enables passing a method as argument to another method. I see that because of this feature it is going to unravel umpteen programming possibilities.
An interface with no method is a marker interface. An interface with only one abstract method is a functional interface. ActionListener class is an example of functional interface. We use anonymous class to implement ActionListener and in this kind of scenario, instead of using anonymous inner classes to implement, lambda expressions can be used. It will be simple and better compared to anonymous inner classes.
Need JDK8 to experiment with lambda expressions. Presently JDK 8 is available as snapshot release JDK 8 Build b 84.
(Argument List) Arrow Token {Body }
(type argument, ….) –> { java statements; }
Lambda Expression Examples – Long list of examples, sure you will enjoy!
() -> { System.out.printlns("Hello World!");} (int a, int b) -> a + b () -> { return 1; } (String name) -> { System.out.println("Hello "+name); } n -> n % 2 != 0
Following sample program implements ActionListener using both anonymous class and lambda expression.
package com.javapapers.java; import java.awt.BorderLayout; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import javax.swing.JButton; import javax.swing.JFrame; public class AnonymousListener { public static void main(String[] args) { JButton anonBtn = new JButton("Java Button"); //actionlistener using anonymous class anonBtn.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent ae) { System.out.println("Anonymous Click!"); } }); //actionlistener using lambda expression anonBtn.addActionListener(e -> { System.out.println("Lambda Click!"); }); JFrame frame = new JFrame("Lambda Expression Sample"); frame.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE); frame.add(anonBtn, BorderLayout.CENTER); frame.pack(); frame.setVisible(true); } }
Comments are closed for "Lambda Expression in Java 8".
Nice article
May be this code helpful for your reader :
public class Calculator {
interface IntegerMath {
int operation(int a, int b);
}
public int operateBinary(int a, int b, IntegerMath op) {
return op.operation(a, b);
}
public static void main(String… args) {
Calculator myApp = new Calculator();
IntegerMath addition = (a, b) -> a + b;
IntegerMath subtraction = (a, b) -> a – b;
System.out.println(“40 + 2 = ” +
myApp.operateBinary(40, 2, addition));
System.out.println(“20 – 10 = ” +
myApp.operateBinary(20, 10, subtraction));
}
}
Why do we use Lambda Expression?
Thanks!!
One issue about anonymous classes is that if the implementation of your anonymous class is very simple, such as an interface that contains only one method, the syntax of anonymous classes may seem too unwieldy and unclear. In these cases, you’re usually trying to pass functionality as an argument to another method, such as what action should be taken when someone clicks a button. Lambda expressions enable you to do this, to treat functionality as method argument, or code as data.
Closures is good but I am sure these syntax and currently available java methods are very similar to Scala and anyway adding closures to java is nice and now java become an MultiParadigm language (Object/Function)
Fantastic…!!!! thanks for the explanation…..
Saved my time to do research on the same :)
Something new in Java. Thanks for sharing
nice to see latest concepts on java..
sir your tutoriles is very excelent for java thanku
gonna revolutionize if it comes out to be true…
How do I get a handle to the ‘event’ object in the Lambda expression example shown with ActionListener..??
My actual question do you think Lambda expressions are a replacement to functional interface implementations..??
Comment
That’s good to know, considering they’ll put it out in the next version of Java. It’ll also make writing listener classes a piece of cake! Thank you for writing this article!
Nice Article..
I am exploring features of Java 8
Will you please explain how virtual Extension method are used in Lambda Expr..?
If you provide an Example it would gr8 for understanding..
Thanks in Advance..:)
Simplest lambda expression could be
()-> system.out.println(“expression”);
() is argument . Here we are passing no argument . So if there is no argument () is the way to define empty argument
system.out.println(“expression”) is expression . Expression is nothing but the body of the lambda expression. Here arguments can be used in various body statements
For example
(a,b)->a+b;
(a,b) is argument here . a+b is expression.
lambda expression are also called anonymous methods. Lambda expressions follow scala type of syntax .
Why do we need these?
These expression add functional edge to the language. Let’s try to understand that with an example
Before that let me make an statement –
An interface having only one method is called functional interface.
You might have written anonymous class using Runnable ,ActionListener interface . For example :
Public class Engine {
public static void main(String args[]){
new Thread(new Runnable(){
public void run()
{
int count=10
while (count>0){
System.out.println(count);
COUNT–;
}
}).start();
}
in above code we are using Runnable interface an anonymous class.
First of all a very nice article & good description of lamda expression.
I belong to field of dot.net c#, but now i am developing app in android with java , and i am missing my lamda exp querying against the object list.
List lst = lst.where(l=>l.age<20).ToList();
now with JAVA 8 i can use this again in android, thats grt.
check out some cool examples when using java 8 lambda and jdeferred
What is the type of ‘e’ in lambda expressin for
//actionlistener using lambda expression
anonBtn.addActionListener(e -> { System.out.println(“Lambda Click!”);
});
[…] of the hot topics in Java 8, which is about to be released. Few months back, I wrote article for introduction to Lambda expressions. In this article I will be presenting few examples using which we can understand Lamda expressions […]
[…] reference using :: is a convenience operator. Method reference is one of the features belonging to Java lambda expressions. Method reference can be expressed using the usual lambda expression syntax format using –> In […]
[…] 22/06/2014 Previous|Next […]
good one
Good example.