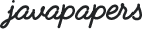
Do you know how to customize the default behaviour of the serialization protocol? Tom Cruise starrer MI4 hits theatres this week in India and we experience unusual media frenzy. In no way this is realted but by impulse I mention about MI4 here. In this article I am going to write on how to customize Ghost protocol, oops serialization protocol.
One of my reader was asked a quesion in an interview on how to customize the serialization behaviour and this article serves as an answer for that question.
In general there are three approaches to serialization in java:
Here protocol means, the way (process or approach) object is serialized and de-serialized.
We have seen enough about using the default protocol to implement serialization and how instances are created during serialization. In this current article we shall see about modifying the default protocol.
import java.io.Serializable; public class Lion implements Serializable { private String sound; public Lion() { System.out.println("Lion's constructor invoked."); setSound("roar"); } public String getSound(){ return sound; } public void setSound(String sound){ this.sound = sound; } }
Above is a simple java class which we are going to use to demonstrate serialization. First let use do simple straight forward serialization and then make the customization in step 2.
import java.io.FileInputStream; import java.io.FileOutputStream; import java.io.IOException; import java.io.ObjectInputStream; import java.io.ObjectOutputStream; public class JavaSerialize { public static void main(String args[]) throws IOException, ClassNotFoundException { Lion leo = new Lion(); // serialize System.out.println("Serialization done."); FileOutputStream fos = new FileOutputStream("serial.out"); ObjectOutputStream oos = new ObjectOutputStream(fos); oos.writeObject(leo); // deserialize FileInputStream fis = new FileInputStream("serial.out"); ObjectInputStream ois = new ObjectInputStream(fis); Lion deserializedObj = (Lion) ois.readObject(); System.out.println("DeSerialization done. Lion: " + deserializedObj.getSound()); } }
Output:
Lion's constructor invoked. Serialization done. DeSerialization done. Lion: roar
Above class just uses the standard mechanism and demonstrates serialization using Lion class.
We know Serializable is a java marker interface. When a class implements Serializable interface it gives information to the JVM that the instances of these classes can be serialized. Along with that, there is a special note to the JVM
look for following two methods in the class that implements Serializable. If found invoke that and continue with serialization process else directly follow the standard serialization protocol.
So this gives us a chance to write these two methods insided the Class that implements Serializable and you get a hook to the serialization process. You can write your custom code inside these two methods and customize the standard behaviour of serialization.
I have modified the Lion class to include these two methods and on the fly I change a property of Lion to demonstrate this. This is not overriding or overloading methods and this is a mechanism provided by serialization. These two included methods are declared private but JVM can access the private methods of an object. There is no change to the class that does the serialization and de-serialization.
import java.io.IOException; import java.io.ObjectInputStream; import java.io.ObjectOutputStream; import java.io.Serializable; public class Lion implements Serializable { private String sound; public Lion() { System.out.println("Lion's constructor invoked."); setSound("roar"); } public String getSound(){ return sound; } public void setSound(String sound){ this.sound = sound; } private void writeObject(ObjectOutputStream out) throws IOException { setSound("meow"); out.defaultWriteObject(); } private void readObject(ObjectInputStream in) throws IOException, ClassNotFoundException { in.defaultReadObject(); } }
Output:
Lion's constructor invoked. Serialization done. DeSerialization done. Lion: meow
Comments are closed for "Customize Default Serialization Protocol".
This is really great article. Your blog is just awesome.
SIr…!!!thank you so much for sharing this article..!
it clears my concept about serialization.
thank you.
Very Simple to understand. Thanks for sharing…
In order to control de-serialization of a Singleton object, which is serializable, we can add a method readResolve():
// This method is called immediately after an object of this class is deserialized.
// This method returns the singleton instance.
protected Object readResolve() {
return singleton;
}
While de-serializing, JVM calls this method, so you can always return the same object always inspite of the Serializable object being Singleton.
As usual, awesome article. Now that you are so much getting into serialization, can we expect one more article explaining the use of “serialVersionUID” in serialization?
Just a small request. See if that can be fulfilled.
And keep the good work as usual. ;-)
hi,
i am bit confused with de-serialization. can u explain wat really happens while de-serializing the object and how constructor’s behave.
Good Article on Serialization and Deserialization .
This s a real good stuff..
Thanks for this article, could you please explain about Externalizable in you own way for my better understanding.
Thanks in Advance.
Good Explanation. Thanks
Good one
Thanks a ton dude.
Thanks. this is really awesome
Sir,
can I write normal static class
If Not why
and i am able to write a inner static class why it is allowed ?
thank you so much for the detailed explanation.
very clear concept
great article bro.i love ur articles a lot bcz they are very simple and very clear.plz keep posting ur articles bro.
Thank you
Great article bro
Sir can you give and example in your way about externalizable interface
This is very helpfull
Dude !!! Your posts are Awesome…they really clear ones concepts…do keep it up…
Hi Joo,
Very good article about deserialization,I have one query here. suppose I have a singleton serializable object, how to deserialize it in a different JVM or Server.
Best Regards,
Venkata
Thank you so much.. it really helped me to understand this concept very well
Thank you so much, very clear and easy to understand
[…] might have seen in my previouse article on how to customize the default implementation of Serializable. These two methods readExternal and writeExternal (Externalizable) supersedes this customized […]
Thanks a lot. God level! Really wanted to know if we can customize them or not. Got it now.
Hats off! Impressive article, sir!
Great information. Thank you.
However, I would request you if you can provide some basic example implementation or scenario where Externalizable would be good idea to use and how to go for it.
Thanks.