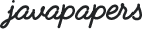
Activity is an Android component that is created as a virtual box. User interface has to be designed on top of this box to allow user to interact with this activity. This is exactly like the HTML’s box method where each HTML tag is considered as a box. Users interact with an android app using screens provided by activity.
An Android app can have multiple activities and one among them will be designated as main activity. If an android app has more than one activity, only one of them can be in active state at a time. These states of the activity are maintained by a stack called back stack. Currently running activity will be on top of the stack. When a new Activity starts the older activity is pushed down the stack and the current activity becomes the top element in the stack which is given user access.
Each activity is interlinked by calling other activities. These subsequent calls form activity life cycle which will be handled by activity manager by interacting. An android activity life cycle consists of three states namely,
When an activity transitions from one state to another, system calls the given callback methods. Following are the available callback methods,
To create an Android activity, a class needs to be written extending the Activity class and by writing the callback methods corresponding to create, stop, resume or destroy operations. These methods (listed above) will be called based on the various stages of the activity among the life cycle process.
Example Activity which has only two callback methods,
public class MainActivity extends Activity { @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); objStatus.updateStatusList("MainActivity","Created"); } @Override protected void onPause() { super.onPause(); objStatus.updateStatusList("MainActivity","Paused"); }}
onCreate() method is the primary callback method which is called when an activity is created. For example, operations like initialization and layout projection will take place.
On projecting layout, View is an important term which is a class that occupies the activity box. Each activity has several views that are coming from viewGroup. There are some built in viewGroup such as form widgets and layout. User can also create their set of views covered by viewGroup. Views are shown using setContentView() method.
When an activity goes out of focus, onPause() method is invoked by andoird system. Pause is the preparation stage before running another activity. This is the place where the existing state of the activity should be persisted.
An Android activity should be declared in the Android manifest file.
For example, the activity is declared as below in the manifest file,
<application> … … <activity android:name=".MainActivity" android:label="@string/title_activity_main" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> … … </application>
In this android:name property represents the class name of the activity.
startActivity() method is used to start another activity. This is done by creating an Intent,
Intent objIntent = new Intent(MainActivity.this, SubActivity.class); //or Intent objIntent = new Intent(Intent.ACTION_MAIN);
After instantiating indent, startActivity() is used to start an activity with this instance.
startActivity(objIntent);
To finish an activity finish() method should be called. This is applicable for the currently active activity. Example,
MainActivity.this.finish();
But, if we want to close a specific activity, then an Intent should be created and passed on to this method as below,
Intent objIntent = new Intent(this, MainActivity.class);
finishActivity(objIntent);
And the corresponding callback method for this state of operation is onFinish(),
public class MainActivity extends Activity { @Override public void onFinish() { … MainActivity.this.finish(); … } }
onPause() is called when an activity is about to lose focus. onStop() is called when the activity is has already lost the focus and it is no longer in the screen. But onPause() is called when the activity is still in the screen, once the method execution is completed then the activity loses focus. So, onPause() is logically before onStop().
From onPause() it is possible to call onResume() but it is not possible once onStop() is called. Once onStop() is called then onRestart() can be called.
onDestroy() is last in the order after onStop(). onDestory() is called just before an activity is destroyed and after that it is gone it is not possible to resurrect this. Simply destroyed and buried!
Now let us see an example of android activity. We will have two activities and see how each of them changes state and how their callback functions are called. The activities are MainActivity and SubActivity. activity_main layout includes a TextView and a Button widget. This is created by drag and drop frome the graphical layout in Eclipse and by setting appropriate properties for them. You may refer our previous article on get user input to know about creating these elements in UI.
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:background="#8BB381" android:onClick="@string/startsub" > <TextView android:id="@+id/textView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerHorizontal="true" android:layout_centerVertical="true" android:background="#FFFFFF" android:text="@string/hello_world" android:textColor="#cc9a7a" tools:context=".MainActivity" /> <Button android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentLeft="true" android:layout_alignParentTop="true" android:layout_marginLeft="18dp" android:layout_marginTop="15dp" android:onClick="@string/startsub" android:text="@string/move_next" android:textColor="#FFFFFF" /> </RelativeLayout>
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:background="#8BB381" android:onClick="@string/startsub" > <TextView android:id="@+id/textView1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerHorizontal="true" android:layout_centerVertical="true" android:background="#FFFFFF" android:text="@string/hello_world" android:textColor="#cc9a7a" tools:context=".MainActivity" /> <Button android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentLeft="true" android:layout_alignParentTop="true" android:layout_marginLeft="18dp" android:layout_marginTop="15dp" android:onClick="@string/startsub" android:text="@string/move_next" android:textColor="#FFFFFF" /> </RelativeLayout>
After designing the layout, we need to develop activity. We create a MainActivity class by extending from Activity class. Inside the MainActivity class the call back functions like onCreate(), onPause() will be created to save the current state of each phase during the life cycle.
package com.javapapers.androidactivity; import android.os.Bundle; import android.app.Activity; import android.content.Intent; import android.view.View; import android.widget.TextView; public class MainActivity extends Activity { TextView objText; ActivityStatus objStatus = new ActivityStatus(); String StatusList; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); objStatus.updateStatusList("MainActivity","Created"); } @Override protected void onStart() { super.onStart(); objStatus.updateStatusList("MainActivity","Started"); } @Override protected void onRestart() { super.onRestart(); objStatus.updateStatusList("MainActivity","Restarted"); } @Override protected void onResume() { super.onResume(); objStatus.updateStatusList("MainActivity","Resumed"); StatusList = objStatus.getStatusList(); objText = (TextView)findViewById(R.id.textView1); objText.setText(StatusList); } @Override protected void onPause() { super.onPause(); objStatus.updateStatusList("MainActivity","Paused"); } @Override protected void onStop() { super.onStop(); objStatus.updateStatusList("MainActivity","Stoped"); } @Override protected void onDestroy() { super.onDestroy(); objStatus.updateStatusList("MainActivity","Destroyed"); } public void startSubActivity(View view) { Intent objIntent = new Intent(MainActivity.this, SubActivity.class); startActivity(objIntent); } }
To save state we need to define the updateStatusList() with two parameters, that is, Activity Name and Activity Status. This method will be invoked in each state of the activity using,
objStatus.updateStatusList(“MainActivity”,”Created”);
The getStatusList() will be used to get the status of all activities inside our application. This method will be invoked inside onResume(), after updating the resumed state.
The updateStatusList() and getStatusList() are used for save/get purposes. The following class holds those functions.
package com.javapapers.androidactivity; import java.util.ArrayList; import java.util.List; public class ActivityStatus { private static List<String> activityStatusAry = new ArrayList<String>(); public void updateStatusList(String activityName, String activityStatus) { for(int i=0;i<activityStatusAry.size();i++) { if(activityStatusAry.get(i).indexOf(activityName)!=-1) { activityStatusAry.remove(i); } } activityStatusAry.add(activityName + " " + activityStatus); } public String getStatusList() { String statusOutput=""; for(int i=0;i<activityStatusAry.size();i++) { statusOutput += activityStatusAry.get(i) +"\r\n"; } return statusOutput; } }
After all the callback functions, startSubActivity() method will handle the start event. Intent initialization refers to start SubActivity from current activity. Then startActivity() is used to start the SubActivity with the reference of the Intent instance.
package com.javapapers.androidactivity; import android.os.Bundle; import android.app.Activity; import android.content.Intent; import android.view.View; import android.widget.TextView; public class SubActivity extends Activity { TextView objText; ActivityStatus objStatus = new ActivityStatus(); String StatusList; @Override public void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_sub); objStatus.updateStatusList("SubActivity","Created"); } @Override protected void onStart() { super.onStart(); objStatus.updateStatusList("SubActivity","Started"); } @Override protected void onRestart() { super.onRestart(); objStatus.updateStatusList("SubActivity","Restarted"); } @Override protected void onResume() { super.onResume(); objStatus.updateStatusList("SubActivity","Resumed"); StatusList = objStatus.getStatusList(); objText = (TextView)findViewById(R.id.textView1); objText.setText(StatusList); } @Override protected void onPause() { super.onPause(); objStatus.updateStatusList("SubActivity","Paused"); } @Override protected void onStop() { super.onStop(); objStatus.updateStatusList("SubActivity","Stoped"); } @Override protected void onDestroy() { super.onDestroy(); objStatus.updateStatusList("SubActivity","Destroyed"); } public void startActivityMain(View view) { Intent objIntent = new Intent(SubActivity.this, MainActivity.class); startActivity(objIntent); } }
Android Activity Example Source Code
Comments are closed for "Android Activity".
Thanks for such detailed information.
Hi Joe,
Thanks for this nice article on android.
Sashi
Your articles are really helping me for may android project. Continue with android.
Great One :)
Good one:)
Reading for the first time I got confused between onPause() ,onResume(), onStop().
A picture is worth a thousand words for transitions among these states.
i need a seminar topic on ajax, please send the catchy title….
Hi Joe, I think we can use the “Log” class for logging purpose instead of separate Activity status class(for demonstration).
you can also give a trail with “Toast messages”
haha, i think javapapers is very good!
I am a new comer, is there any Chinese here?
Very Good explanation of Android Activity……….
Thanks for this lovely tutorial…..
good example inandriod.pls put andriod fragmentations.
Sure Jyothikumar noted, will write on that topic soon.
The R file is not found in the source code.
[…] that will play the audio file. Service is an Android application component and different from Android activity component. Following steps will help to create an android app to play audio as a service in […]
[…] entered by the user. The content to be broadcasted will be taken from the user through a main Android activity component. On clicking the send button, this content will be send to the broadcast receiver […]
[…] have three Android Activity for List, Add and Edit operations and ensure that these are declared in manifest file. And then we […]
Thanks for you detailed explanation of android life cycle. I am new to android or java. I got a further question about this:
For an app with lots of activity, do we have only one onCreate()? I made an app once in a while come up with a box says “Unfortunately has stopped”. Is that because I have onCreate() in every activity ?
hey Chenlibo,
This is a friendly community out here. Shoot your question, hang around and enjoy!
its realy helping , every point breafly explain
Detailed explanation of life cycle helped in understanding
thank you
[…] tutorial is to explain how to create a date picker dialog in android. This dialog will disable the background activity until the user select the date and click ‘done’ tab of the dialog […]
i want to learn eclipse. tell me how to start and how to proceed.
[…] our example, this is the default Android Activity for the Android app. This is the email compose screen, when we start this example app we will just […]
THANKS!!
[…] have two Android Activity SplashScreenActivity and AppMainActivity. One for a splash screen and another for application main […]
Hi Joe, why those methods are called as callback methods? Why not simply functions?