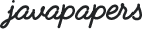
This Android tutorial will help to learn how to show splash screen in an Android application. Splash screen is generally used when there is a need to do some background process when an application is initiated. Background process like loading the database, images, making a call over network. These kind of activities will consume time and a splash screen can be shown during that time. A splash screen can be the app icon, company logo and nice welcome image etcetera. Sometimes, splash screens are shown even when there is no need for a background process. This is just to showcase the brand through logo or some image and in this kind of promotional situations it is done based on fixed time interval.
In this tutorial, let us see how a splash screen can be shown in an Android application. Let us see a generic code which can be used for both the cases, to show a splash screen during a background process or to show a splash screen for a fixed time interval.
package com.javapapers.android.androidsplashscreen; import android.app.Activity; import android.content.Intent; import android.os.AsyncTask; import android.os.Bundle; public class SplashScreenActivity extends Activity { private static final int SPLASH_SHOW_TIME = 5000; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_splash_screen); new BackgroundSplashTask().execute(); } /** * Async Task: can be used to load DB, images during which the splash screen * is shown to user */ private class BackgroundSplashTask extends AsyncTask{ @Override protected void onPreExecute() { super.onPreExecute(); } @Override protected Void doInBackground(Void... arg0) { // I have just given a sleep for this thread // if you want to load database, make // network calls, load images // you can do them here and remove the following // sleep // do not worry about this Thread.sleep // this is an async task, it will not disrupt the UI try { Thread.sleep(SPLASH_SHOW_TIME); } catch (InterruptedException e) { e.printStackTrace(); } return null; } @Override protected void onPostExecute(Void result) { super.onPostExecute(result); Intent i = new Intent(SplashScreenActivity.this, AppMainActivity.class); // any info loaded can during splash_show // can be passed to main activity using // below i.putExtra("loaded_info", " "); startActivity(i); finish(); } } }
<!-- @drawable/backrepeat - refers to the image - check backrepeat.xml --> <RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" android:layout_width="fill_parent" android:layout_height="fill_parent" android:background="@drawable/backrepeat" > <ImageView android:id="@+id/imgLogo" android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_centerInParent="true" android:src="@drawable/javapapers" android:tileMode="repeat" /> <TextView android:layout_width="fill_parent" android:layout_height="wrap_content" android:layout_alignParentBottom="true" android:layout_marginBottom="10dp" android:gravity="center_horizontal" android:text="javapapers.com" android:textColor="#454545" android:textSize="12sp" /> </RelativeLayout>
This is just to show how to tile (repeat) an image background in an Android activity
<?xml version="1.0" encoding="utf-8"?> <bitmap xmlns:android="http://schemas.android.com/apk/res/android" android:src="@drawable/background" android:tileMode="repeat" />
This Android Manifest file is just to show that the launcher activity is the splash screen.
<?xml version="1.0" encoding="utf-8"?> <manifest xmlns:android="http://schemas.android.com/apk/res/android" package="com.javapapers.android.androidsplashscreen" android:versionCode="1" android:versionName="1.0" > <uses-sdk android:minSdkVersion="8" android:targetSdkVersion="18" /> <application android:allowBackup="true" android:icon="@drawable/ic_launcher" android:label="@string/app_name" android:theme="@style/AppTheme" > <activity android:name="com.javapapers.android.androidsplashscreen.SplashScreenActivity" android:label="@string/app_name" > <intent-filter> <action android:name="android.intent.action.MAIN" /> <category android:name="android.intent.category.LAUNCHER" /> </intent-filter> </activity> <activity android:name="com.javapapers.android.androidsplashscreen.AppMainActivity" android:label="@string/app_name" > </activity> </application> </manifest>
package com.javapapers.android.androidsplashscreen; import android.app.Activity; import android.os.Bundle; import android.view.Menu; /* * A placeholder main activity for this Android App * After splash screen is displayed this main acitivity * will be shown. */ public class AppMainActivity extends Activity { @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_app_main); } @Override public boolean onCreateOptionsMenu(Menu menu) { getMenuInflater().inflate(R.menu.app_main, menu); return true; } }
<RelativeLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:paddingBottom="@dimen/activity_vertical_margin" android:paddingLeft="@dimen/activity_horizontal_margin" android:paddingRight="@dimen/activity_horizontal_margin" android:paddingTop="@dimen/activity_vertical_margin" tools:context=".AppMainActivity" > <TextView android:layout_width="wrap_content" android:layout_height="wrap_content" android:layout_alignParentTop="true" android:layout_centerHorizontal="true" android:layout_marginTop="182dp" android:text="@string/hello_world" android:textSize="30dp" /> </RelativeLayout>
Comments are closed for "Android Splash Screen".
nice post for splash.thanx
nice one
Must raise case on parameters ‘void’ to ‘Void’ on private inner class declaration. Other than that … nice tutorial!
[…] Above shown screen shot is the splash screen of the chat app we are going to develop. If you want to know how to create this splash screen refer the tutorial Android splash screen. […]
Great tutorial, thank you!
nice tutorial..thanks..
in my MainActivity there is a webview.It takes too much time to load the page. so i want to start loading the webpage in my MainActivity in background during splashscreen running. so where should i code?
please help me..
Nice post thanks for it
I am new to android.
I seen that many application put application overview as slides how can i achieve that.
please can you help me.
Sorry to mention about application name
airbnb having same thing
Nice post.. This information may improve the user experience.