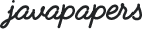
@Order is used to define sort order for components annotated with this annotation. @Order annotation is available in Spring Framework since version 2.0. Till Spring 4 , it supports ordering only for AspectJ aspects. Spring 4 made a nice little enhancement to @Order annotation. It introduced support to ordering of auto-wired components in collections like Lists and Arrays.
Internally, @Order uses “OrderComparator” class in Spring XML Based applications or “AnnotationAwareOrderComparator” class in Spring Annotation Based Applications to order components. In this Spring tutorial we shall see about @Order annotation in detail with an example Spring project.
In Spring Framework, @Order annotation is defined as shown below:
@Retention(value=RUNTIME) @Target(value={TYPE,METHOD,FIELD}) @Documented public @interface Order
This annotation contains one attribute “value” which accepts the integer values like 1, 2, etc. Lowest Values have higher precedence. That is Lowest values comes first in List or Array.
Now, let us demonstrate how this annotation works in both Spring 3.x and Spring 4.x Frameworks.
Let us assume that we are going to develop an Application using Spring 4 Framework for an University. One of the scenario of this applications is that a set of Student Ranks says RankOne, RankTwo,RankThree etc. We use List or Array to store these Ranks Components in Results component using Auto-wiring. We need to observe how they order in List or Array in both Spring 3.x and Spring 4.x Frameworks.
We are going to develop this Application scenario using Spring 3.2 Framework. Create a Maven Project in Spring STS Suite with the following components. Develop one interface to represent Students Rank and set of implementations like RankOne,RankTwo, RankThree etc.
package com.javapapers.spring3.autowire.collection; public interface Ranks { }
package com.javapapers.spring3.autowire.collection; import org.springframework.core.annotation.Order; import org.springframework.stereotype.Component; @Component public class RankOne implements Ranks{ private String rank = "RankOne"; public String toString(){ return this.rank; } }
package com.javapapers.spring3.autowire.collection; import org.springframework.core.annotation.Order; import org.springframework.stereotype.Component; @Component public class RankTwo implements Ranks{ private String rank = "RankTwo"; public String toString(){ return this.rank; } }
package com.javapapers.spring3.autowire.collection; import org.springframework.core.annotation.Order; import org.springframework.stereotype.Component; @Component public class RankThree implements Ranks{ private String rank = "RankThree"; public String toString(){ return this.rank; } }
Component to hold student ranks in a collection as shown below.
package com.javapapers.spring3.autowire.collection; import java.util.List; import org.springframework.beans.factory.annotation.Autowired; import org.springframework.stereotype.Component; @Component public class Results { @Autowired private Listranks ; @Override public String toString(){ return ranks.toString(); } }
<?xml version="1.0" encoding="UTF-8"?> <beans xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xmlns="http://www.springframework.org/schema/beans" xmlns:context="http://www.springframework.org/schema/context" xsi:schemaLocation="http://www.springframework.org/schema/beans http://www.springframework.org/schema/beans/spring-beans.xsd http://www.springframework.org/schema/context http://www.springframework.org/schema/context/spring-context.xsd"> <context:annotation-config /> <context:component-scan base-package="com.javapapers.spring3"/> </beans>
package com.javapapers.spring3.autowire.collection; import org.springframework.context.ApplicationContext; import org.springframework.context.support.ClassPathXmlApplicationContext; public class RanksClient { public static void main(String[] args) { ApplicationContext context = new ClassPathXmlApplicationContext("beans.xml"); Results results = (Results)context.getBean("results"); System.out.println(results); } }
<project xmlns="http://maven.apache.org/POM/4.0.0" xmlns:xsi="http://www.w3.org/2001/XMLSchema-instance" xsi:schemaLocation="http://maven.apache.org/POM/4.0.0 http://maven.apache.org/xsd/maven-4.0.0.xsd"> <modelVersion>4.0.0</modelVersion> <groupId>org.springframework.samples</groupId> <artifactId>Spring3.1AutoWiringCollectionObject</artifactId> <version>1.0.0</version> <properties> <java.version>1.8</java.version> <spring-framework.version>3.1.0.RELEASE</spring-framework.version> </properties> <dependencies> <dependency> <groupId>org.springframework</groupId> <artifactId>spring-context</artifactId> <version>${spring-framework.version}</version> </dependency> </dependencies> <build> <plugins> <plugin> <groupId>org.apache.maven.plugins</groupId> <artifactId>maven-compiler-plugin</artifactId> <version>3.1</version> <configuration> <source>${java.version}</source> <target>${java.version}</target> </configuration> </plugin> </plugins> </build> </project>
The final project should look like below.
It’s time to run this application and observe the results. As our client has plain main() method, just run the client java file as “Java application” in Spring STS Suite.
[RankOne, RankThree, RankTwo]
Here, Our Ranks component contains a list of Ranks in unordered format. As Spring 3.x does not support @Order
annotation to order Auto-wired List, Ranks component contains a list of unordered Ranks. Now lets head out to Spring 4 and see the difference @Order
makes there for this same example.
Download Project: Spring 3 AutoWiring CollectionObject
Create a Spring 4 Maven Project with the following components. As this project uses same Spring 3 components, I will list only changes here.
Use all Java classes as it is except with the following changes. Add @Order annotation for all Ranks implemented class that is RankOne, RankTwo and RankThree.
package com.javapapers.spring4.autowire.collection; import org.springframework.core.annotation.Order; import org.springframework.stereotype.Component; @Component @Order(1) public class RankOne implements Ranks{ // Same code as given above }
So the only change is, we have added the @Order
annotation as shown above. Add Spring 4 in Maven pom as dependency. Now run the project and you will get the following output.
[RankOne, RankTwo, RankThree]
As we have assigned @Order
annotation for all Ranks-Implemented classes as 1,2 and 3; they have auto-wired in the same order.
@Order annotation by default follows lowest to higher ordering that is Lowest value has high priority. That means they comes first in List or Array. Because by default, ordering precedence is set to Ordered.LOWEST_PRECEDENCE
. If you want highest values first then we need to change this value to Ordered.HIGHEST_PRECEDENCE
.
Download Project: Spring 4 AutoWiring Collection Object
Comments are closed for "Spring @Order Annotation".
beautifully explained. (y)
Hello,
Very well explained. One question regarding 3.x implementation.
How
@Autowired
private List ranks ;
will add Rank objects in list ? Should this be like
@Autowired
private List ranks
Or I am missing something ?
Thanks