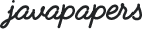
Java PriorityBlockingQueue is a concurrent collection and an implementation of BlockingQueue. PriorityBlockingQueue is an unbounded collection. Ordering of elements in the PriorityBlockingQueue is done same as in PriorityQueue.
We should see PriorityBlockingQueue
as a PriorityQueue
with added operations for blocking retrieval. All the rules of PriorityQueue applies here like elements are ordered based on their priority level among the elements.
Let us have a look at a producer-consumer based example to understand the PriorityBlockingQueue
.
package com.javapapers.java.collections; import java.util.Random; import java.util.UUID; import java.util.concurrent.BlockingQueue; public class PriorityBlockingQueueProducer implements Runnable { protected BlockingQueue<String> blockingQueue; final Random random = new Random(); public PriorityBlockingQueueProducer(BlockingQueue<String> queue) { this.blockingQueue = queue; } @Override public void run() { while (true) { try { String data = UUID.randomUUID().toString(); System.out.println("Put: " + data); blockingQueue.put(data); Thread.sleep(500); } catch (InterruptedException e) { e.printStackTrace(); } } } }
package com.javapapers.java.collections; import java.util.concurrent.BlockingQueue; public class PriorityBlockingQueueConsumer implements Runnable { protected BlockingQueue<String> blockingQueue; public PriorityBlockingQueueConsumer(BlockingQueue<String> queue) { this.blockingQueue = queue; } @Override public void run() { while (true) { try { String data = blockingQueue.take(); System.out.println(Thread.currentThread().getName() + " take(): " + data); Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } } } }
package com.javapapers.java.collections; import java.util.concurrent.BlockingQueue; import java.util.concurrent.LinkedBlockingQueue; public class PriorityBlockingQueueExample { public static void main(String[] args) { final BlockingQueue<String> priorityBlockingQueue = new LinkedBlockingQueue<String>(); PriorityBlockingQueueProducer queueProducer = new PriorityBlockingQueueProducer( priorityBlockingQueue); new Thread(queueProducer).start(); PriorityBlockingQueueConsumer queueConsumer1 = new PriorityBlockingQueueConsumer( priorityBlockingQueue); new Thread(queueConsumer1).start(); PriorityBlockingQueueConsumer queueConsumer2 = new PriorityBlockingQueueConsumer( priorityBlockingQueue); new Thread(queueConsumer2).start(); } }
Put: ebc55884-84d7-4621-b3d7-2e7c9c428a13 Thread-1 take(): ebc55884-84d7-4621-b3d7-2e7c9c428a13 Put: 1f8e4b4e-03df-4068-a2e1-423f1f6091a1 Thread-2 take(): 1f8e4b4e-03df-4068-a2e1-423f1f6091a1 Put: 55a38169-24a1-459b-ad1a-51b0ba36a7b8 Thread-1 take(): 55a38169-24a1-459b-ad1a-51b0ba36a7b8 Put: 84af8597-2796-4050-8c19-711d68f8ce6b Thread-2 take(): 84af8597-2796-4050-8c19-711d68f8ce6b Put: 3cb2bcae-8a71-4d75-96bb-72549ffd6283 Thread-1 take(): 3cb2bcae-8a71-4d75-96bb-72549ffd6283
Comments are closed for "Java PriorityBlockingQueue".
[…] PriorityBlockingQueue […]
final BlockingQueue priorityBlockingQueue = new LinkedBlockingQueue();
should be
final BlockingQueue priorityBlockingQueue = new PriorityBlockingQueue();