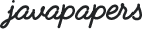
This tutorial is part of the Java NIO tutorial series. In this tutorial, we will see about what is a Path and a File with respect to Java NIO. Path is step one in files and IO processing. Files are stored in a disk or some media based on a file system. Tree structure based file system is popularly prevalent in current day operating systems. Tree structure starts with a root node and branches. There can be multiple root nodes (C:, D:) as we have in Windows.
A path can be used to identify and locate a file in the file system. There are two types of paths, relative and absolute. Absolute path is self sufficient, that is a file can be located with an absolute path without any other information. So the absolute path starts with the root node and ends with the file name identifier. In case of relative path, we need to combine it with another path to get the absolute path to locate the file.
java.nio.file.Path is an interface and it is used to represent a system dependent file path which can be used to locate a file in a file system.
Following is an example way to instantiate a file (relative) path, “lib” is a directory name relative to current directory and “nio.jar” is a file name in that directory.
//import java.nio.file.FileSystems; //import java.nio.file.Path; Path path = FileSystems.getDefault().getPath("lib", "nio.jar");
Following is another example to instantiate a file (absolute) path.
//import java.nio.file.Path; //import java.nio.file.Paths; Path filePath = Paths.get("/etc/user/foo");
NOTE: Paths.get
is a convenience method for FileSystems.getDefault().getPath
. So the same code can be written as FileSystems.getDefault().getPath("/etc/user/foo");
To get the current working directory with NIO, we can use the following code and it returns absolute path of current working directory.
Path currentDirectory = Paths.get("").toAbsolutePath();
To join two paths in NIO, we can use the resolve
method. If an absolute path is passed as parameter to resolve method, then the same is returned.
// In Windows OS Path path1 = Paths.get("C:\\Users\\Java\\examples"); // Output is C:\Users\Java\examples\Test.java System.out.println(path1.resolve("Test.java"));
In NIO, when we want to construct a Path from one location to another we can use the method relativize
. This can be used to navigate between two paths.
Path path1 = Paths.get("C:\\Users"); Path path2 = Paths.get("C:\\Users\\Java\\examples"); // outcome is Java\examples Path path1_to_path2 = path1.relativize(path2); // outcome is ..\.. Path path2_to_path1 = path2.relativize(path1);
When we want to redundant information from Path, NIO provides the normalize
method. In popular file systems, current directory is denoted by “.” and the parent directory by “..”. These notations can be present in a path. If we want to remove these notations from the Path and converts it.
//normalize Path path5 = Paths.get("C:\\Users\\Java\\.\\examples"); //output C:\Users\Java\examples System.out.println(path5.normalize()); //output C:\Users\examples Path path6 = Paths.get("C:\\Users\\Java\\..\\examples"); System.out.println(path6.normalize());
package com.javapapers.java.nio; import java.nio.file.FileSystems; import java.nio.file.Path; import java.nio.file.Paths; public class PathFileNIO { public static void main(String... strings) { // instantiate a relative path Path p1 = FileSystems.getDefault().getPath("lib", "nio.jar"); // instantiate a absolute path - windows OS Path p2 = Paths.get("C:\\Users\\Java\\examples"); // get current working directory Path currentDirectory = Paths.get("").toAbsolutePath(); System.out.println(currentDirectory.toAbsolutePath()); // resolve path in Windows OS Path path1 = Paths.get("C:\\Users\\Java\\examples"); // Output is C:\Users\Java\examples\Test.java System.out.println(path1.resolve("Test.java")); // relativize Path path3 = Paths.get("C:\\Users"); Path path4 = Paths.get("C:\\Users\\Java\\examples"); // outcome is Java\examples Path path3_to_path4 = path3.relativize(path4); System.out.println(path3_to_path4); // outcome is ..\.. Path path4_to_path3 = path4.relativize(path3); System.out.println(path4_to_path3); //normalize Path path5 = Paths.get("C:\\Users\\Java\\.\\examples"); //output C:\Users\Java\examples System.out.println(path5.normalize()); //output C:\Users\examples Path path6 = Paths.get("C:\\Users\\Java\\..\\examples"); System.out.println(path6.normalize()); } }
Comments are closed for "Java NIO Path".
[…] NIO Path […]
I was reading this tutorial on my mobile and one thought came to my mind that you should have native android & iOs app for your site… if you want then i’ll do this for you… waititng for your reply. Thanks.
I used to think of NIO as a complex and difficult to understand thing, but now after reading this article I believe it’s not what I used to think of it as…
Thanks Joe