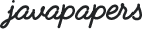
This Java tutorial is to learn about handling file using Java NIO. This tutorial is part of the Java NIO tutorial series. We will see about basic file operations like how to delete, copy, move a file and more.
There are two methods available in Files
class using which we can delete files. The methods are delete(Path)
and deleteIfExists(Path)
. Using these methods we can,
// create a path for file named temp.txt from current folder Path path = FileSystems.getDefault().getPath(".", "temp.txt"); try { Files.delete(path); } catch (NoSuchFileException x) { System.err.println(path + "no exists"); } catch (DirectoryNotEmptyException x) { System.err.println(path + "directory not empty"); } catch (IOException x) { System.err.println(path + "file permission issue"); S}
Using deleteIfExists(Path)
method does not throw exception when the file does not exists. It just fails silently.
When we attempt to delete a directory, it should be empty to be deleted. Therefore we should delete all files and sub-directories that are inside a directory before deleting it. In Files
class we have got method to walk a file tree. Using SimpleFileVisitor
we can create a simple visitor to visit all files. Using these two we can delete a directory and all its contents recursively.
package com.javapapers.java.nio; import java.io.IOException; import java.nio.file.FileVisitResult; import java.nio.file.Files; import java.nio.file.Path; import java.nio.file.Paths; import java.nio.file.SimpleFileVisitor; import java.nio.file.attribute.BasicFileAttributes; /* * delete a directory and its contents recursively */ public class DeleteVisitor extends SimpleFileVisitor<Path> { @Override public FileVisitResult visitFile(Path file, BasicFileAttributes attributes) throws IOException { Files.delete(file); System.out.println(file.getFileName() + " deleted."); return FileVisitResult.CONTINUE; } @Override public FileVisitResult postVisitDirectory(Path directory, IOException ioe) throws IOException { Files.delete(directory); System.out.println(directory.getFileName() + " directory deleted."); return FileVisitResult.CONTINUE; } public static void main(String args[]) { Path directoryPath = Paths.get(".", "todelete"); DeleteVisitor delelteVisitor = new DeleteVisitor(); try { Files.walkFileTree(directoryPath, delelteVisitor); } catch (IOException ioe) { ioe.printStackTrace(); } } }
temp3.txt deleted.
subdirectory directory deleted.
temp1.txt deleted.
temp2.txt deleted.
todelete directory deleted.
if (path.isDirectory()) { for (File file : path.listFiles()) delete(file); }
Deleting a directory is done, but how do we create a directory using file NIO. Files
class provides a method createDirectory(Path)
to create a directory. It throws exception if a directory already exists in the same name.
Path path = Paths.get("tempdir/subdirectory"); try { Path newDirectory = Files.createDirectory(directoryPath); } catch(FileAlreadyExistsException e){ //directory already exists } catch (IOException e) { //permission issue e.printStackTrace(); }
NIO Files
class provides copy(..)
method to copy directory. When a directory is copied, files inside that directory will not be copied. Unlike directories, when copying a symbolic link the target is also copied. However, there are options available to not copy the target files.
Path sourcePath = Paths.get("foo/test.txt"); Path destinationPath = Paths.get("bar/test-copy.txt"); try { Files.copy(sourcePath, destinationPath); } catch(FileAlreadyExistsException e) { //file already exists and unable to copy } catch (IOException e) { //permission issue e.printStackTrace(); }
We can forcible overwrite an existing file. We can pass a parameter to copy method to replace existing file.
Path sourcePath = Paths.get("foo/test.txt"); Path destinationPath = Paths.get("bar/test-copy.txt"); try { Files.copy(sourcePath, destinationPath, StandardCopyOption.REPLACE_EXISTING); } catch (IOException e) { // permission issue e.printStackTrace(); }
The NIO utility class Files contains method to move files from a source to a destination path. If the target is the same file then the move fails silently. It the moved file is a symbolic link then only the link is moved and not the target. When it comes to moving a directory, if it is empty then it is moved and we can rename it in the process. If the directory contains files that are not links are special files that cannot be moved then it fails. Renaming a file / directory without moving it to a new location can also be done.
Path sourcePath = Paths.get("foo/test.txt"); Path destinationPath = Paths.get("bar/test-copy.txt"); try { Files.move(sourcePath, destinationPath, StandardCopyOption.REPLACE_EXISTING); } catch (IOException e) { e.printStackTrace(); }
Comments are closed for "Java NIO Files – Delete Copy Move".
[…] Java NIO Files – Delete Copy Move […]