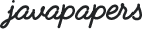
This Java tutorial is to learn about the collection LinkedBlockingQueue which is an implementation of Java BlockingQueue
. LinkedBlockingQueue order elements first-in-first-out (FIFO). With respect to bounds of the LinkedBlockingQueue
, it stands in between ArrayBlockingQueue
and DelayQueue
.
LinkeBlockingQueue has an aditional constructor which provides the capability to instantiate with fixed capacity. If not the other regular argument-less constructor creates instance with Integer.MAX_VALUE
capacity.
poll
and offer
method behaves as defined in BlockingQueue. poll method without argument retrieves the head element from the queue. poll method with timeout argument removes the head element by waiting for the time specified if the element is not available.
We will see a standard producer-consumer scenario example to understand the LinkeBlockingQueue.
package com.javapapers.java.collections; import java.util.Random; import java.util.UUID; import java.util.concurrent.BlockingQueue; public class LinkedBlockingQueueProducer implements Runnable { protected BlockingQueue<String> blockingQueue; final Random random = new Random(); public LinkedBlockingQueueProducer(BlockingQueue<String> queue) { this.blockingQueue = queue; } @Override public void run() { while (true) { try { String data = UUID.randomUUID().toString(); System.out.println("Put: " + data); blockingQueue.put(data); Thread.sleep(500); } catch (InterruptedException e) { e.printStackTrace(); } } } }
package com.javapapers.java.collections; import java.util.concurrent.BlockingQueue; public class LinkedBlockingQueueConsumer implements Runnable { protected BlockingQueue<String> blockingQueue; public LinkedBlockingQueueConsumer(BlockingQueue<String> queue) { this.blockingQueue = queue; } @Override public void run() { while (true) { try { String data = blockingQueue.take(); System.out.println(Thread.currentThread().getName() + " take(): " + data); Thread.sleep(1000); } catch (InterruptedException e) { e.printStackTrace(); } } } }
package com.javapapers.java.collections; import java.util.concurrent.BlockingQueue; import java.util.concurrent.LinkedBlockingQueue; public class LinkedBlockingQueueExample { public static void main(String[] args) { final BlockingQueue<String> linkedBlockingQueue = new LinkedBlockingQueue<String>(); LinkedBlockingQueueProducer queueProducer = new LinkedBlockingQueueProducer( linkedBlockingQueue); new Thread(queueProducer).start(); LinkedBlockingQueueConsumer queueConsumer1 = new LinkedBlockingQueueConsumer( linkedBlockingQueue); new Thread(queueConsumer1).start(); LinkedBlockingQueueConsumer queueConsumer2 = new LinkedBlockingQueueConsumer( linkedBlockingQueue); new Thread(queueConsumer2).start(); } }
Put: f1ef7505-6fe5-436c-893a-dd6ab8ecdba4 Thread-1 take(): f1ef7505-6fe5-436c-893a-dd6ab8ecdba4 Put: 8c86787c-a7bf-4124-b0c4-c62a195f9096 Thread-2 take(): 8c86787c-a7bf-4124-b0c4-c62a195f9096 Put: c5248f9b-17c0-41f6-a071-fecaa1dd21d3 Thread-1 take(): c5248f9b-17c0-41f6-a071-fecaa1dd21d3 Put: 05e7243e-62d4-4f32-9c9b-4b811245c571 Thread-2 take(): 05e7243e-62d4-4f32-9c9b-4b811245c571 Put: 7848bb81-a0f4-423c-b093-21db9d4412b1 Thread-1 take(): 7848bb81-a0f4-423c-b093-21db9d4412b1
Comments are closed for "Java LinkedBlockingQueue".
[…] LinkedBlockingQueue […]