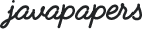
This Java tutorial is to help understand what are abstract classes and methods. This tutorial is applicable for Java beginners. An abstract class in Java cannot be instantiated. It does not end with it. Can a Java abstract class have a constructor? Can an abstract method be defined as static? If you are not comfortable with these questions, then you should read this tutorial and refresh the basics.
A Java class that is declared using the keyword abstract
is called an abstract class. New instances cannot be created for an abstract class but it can be extended. An abstract class can have abstract methods and concrete methods or both. Methods with implementation body are concrete methods. An abstract class can have static fields and methods and they can be used the same way as used in a concrete class. Following is an example for Java abstract class.
public abstract class Animal { }
We cannot create new instances for the above Animal class as it is declared as ‘abstract’.
A method that is declared using the keyword abstract
is called an abstract method. Abstract methods are declaration only and it will not have implementation. It will not have a method body. A Java class containing an abstract class must be declared as abstract class. An abstract method can only set a visibility modifier, one of public or protected. That is, an abstract method cannot add static or final modifier to the declaration. Following is an example for Java abstract method.
public abstract class Animal { String name; public abstract String getSound(); public String getName() { return name; } }
When an abstract class is implemented in Java, generally all its abstract methods will be defined. If one or more abstract method is not defined in the implementing class, then it also should be declared as an abstract class. Following is an example class that implements the Animal abstract class. @Override
is a Java annotation used to state that this method overrides the method in the super class.
public class Lion extends Animal { @Override public String getSound() { return "roar"; } }
It is possible for an abstract class to implement a Java interface. If the implementing class does not implement all of the abstract methods from the interface, then this must be defined an abstract class in Java. In the below example, Animal class does not implement the abstract method from Species interface. Though Animal does not have any abstract method on its own, it must be declared as abstract since it did not implement the abstract method from Species interface. Any class that extends the Animal class should implement the getClassification abstract method. Difference between an interface and abstract class is methods in an interface are implicitly abstract. Go through this linked tutorial to know more about the differences.
public interface Species { public String getClassification(); }
public abstract class Animal implements Species { String name; public String getName() { return name; } }
We should go for abstract class when we are working with classes that contains similar code. That is, there is a possibility to template behavior and attributes. Common behavior can be elevated to a super class and provide implementation to it. Then add behavior that cannot be implemented and declare it as abstract. Classes that are similar to this abstract class will extend it and use the already implemented methods and add implementation for abstract methods.
In the above Animal example given, name is a common attributes for animals and so it is elevate to the Animal super class. getName is a common behavior for all animal and it depends on the common attribute name. So implementation for this getName is provided in the abstract super class. getSound depends on individual animals and so it is declared abstract and left for extending class to implement it. What we gain from this template pattern is avoiding repetition of common code.
AbstractMap
is an abstract class part of Collections Framework in the Java JDK. It is extended by a long list of Subclasses ConcurrentHashMap, ConcurrentSkipListMap, EnumMap, HashMap, IdentityHashMap, TreeMap, WeakHashMap. These class share and reuse many methods from this abstract class like get
, put
, isEmpty
.
Yes, an abstract class can have constructor in Java. It can be a useful option to enforce class constraints like setting up a field. Let me demonstrate it using our Animal example below.
public abstract class Animal { String name; public Animal(String name) { this.name = name; } public String getName() { return name; } public abstract String getSound(); }
This abstract class defines a constructor with an argument that is used to setup the field name. Classes that extends this abstract class should define a constructor with implicit super()
call to the super abstract class. Otherwise we will get an error as “Implicit super constructor Animal() is undefined. Must explicitly invoke another constructor”. This is to do some initialization before instantiation.
public class Lion extends Animal { public Lion(String name) { super(name); } @Override public String getSound() { return "roar"; } }
No, an abstract class cannot be declared as final
in Java. Because it will completely negate the purpose of an abstract class. An abstract class should be extended to create instances. If it is declared final, then it cannot be extended and so an abstract class cannot be declared as final.
Comments are closed for "Java Abstract Class and Methods".
This is really a necessity to read through Java basics once in a while. Whenever there is such a need I come to JavaPapers.
This tutorial is simple and comprehensive. You are the best.
Do you have any idea to come up with Java video tutorials?
Pure gold! Nothing else to say.
First I thought it is so basic and felt good after reading through. Thanks.
Good tutorial Joe.
Very nice tutorial.
Perfect tutorial for beginners .Thanks
Hello Joe,
As usual this is very good tutorial. One suggestion is that in the UML diagram drawn the direction of the realization and inheritance arrows is to be reversed.
Thank you.
Very good tutorial!
@Kapil, thanks for the comment. I have corrected it :-)
Very good explanation. Much appreciated for your efforts put on this. :)
And noticed “A Java class containing an abstract class must be declared as abstract class.” I guess, instead of class, need to add method.
We have all methods are abstract in abstract class.
Interface or Abstract class which one is better for the above scenario.
Dear Sir,
I have a list of question as below.
1-why to use abstract class?
2-what is the benefit we get from abstract?
3-is it necessary to make a class abstract who extends an abstract class but not implement all abstract method? but it works.
I know to declare and use abstract class but did not get understood
Thanks & Regards,
Sunil Kumar