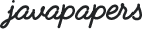
This tutorial serves as ProcessBuilder feature introduction. For some need, I was attempting to print OS environment variables using java. Generally we get that done using the versatile System class like System.getenv(); This is very old as you can see the method name itself is not as per java convention, available from JDK 1.0
Since JDK 1.5 we have got a class java.lang.ProcessBuilder which is used to create OS processes. This API is available from JDK 1.5 but key features like redirect are added in JDK 1.7. We can use this ProcessBuilder to get current thread’s environment variables as below
package com.javapapers.corejava; import java.util.Map; public class ProcessBuilderSample { public static void main(String args[]) { ProcessBuilder testProcess = new ProcessBuilder(); Map environmentMap = testProcess.environment(); System.out.println(environmentMap); // usual way System.out.println(System.getenv()); } }
This is not the sole purpose of this class. It helps to create and execute OS processes and also gives us lot of flexibility over the already available System class.
Following example demonstrates how we can execute a set of native OS commands and get output/error redirected to a file. We can pass the commands from a file as we have done in this sample or we can pass it at run-time.
package com.javapapers.corejava; import java.io.File; import java.io.IOException; public class ProcessBuilderSample { public static void main(String args[]) throws IOException { ProcessBuilder dirProcess = new ProcessBuilder("cmd"); File commands = new File("C:/process/commands.bat"); File dirOut = new File("C:/process/out.txt"); File dirErr = new File("C:/process/err.txt"); dirProcess.redirectInput(commands); dirProcess.redirectOutput(dirOut); dirProcess.redirectError(dirErr); dirProcess.start(); } }
To run the above program,
create a folder name “process” in C:/ and create the three files.
In commands.bat, put the following commands or something you like,
chdir
dir
date /t
echo Hello World
remember to run on JDK 1.7 and you can see the output in out.txt
Comments are closed for "OS Processes using Java ProcessBuilder".
Nice one as always :)
Something new to me.
Joe, ROCKS!!!
Good one..
Nice… thanks
Good one. Thanks.
nice example….
I like your posts about java. Thanks from Slovakia (-;
Really its very…nice blog. Its provide a lot of new things in java.
Thanks joe from sahil
very nice
Simple but Nice!Good job! :-)
Hi Joe,
Is above program works in Linux environment?
It is very nice. It can be elaborated using examples.
Nice to know new updates. Very helpful. Thank you sir.
Thanks for the introducing new features. Can we have more posts regarding this topic. It will be very helpful to learn more about process builder.
Great work….Please keep it up..
hello sir,
good stuff, I would like a software that monitors the user’s activity such as openning of files, copying, moving, deletion etc. How can i do it in Java?
hi..
i got error in all these line..because these
methods not lies in class..
dirProcess.redirectInput(commands);
dirProcess.redirectOutput(dirOut);
dirProcess.redirectError(dirErr);
what is solution..?
Very nice. Thanks from Albania!
im developing one scheduling of jsp web application can you sugest me how to do that by the way i did one simple banking application ie at one time 4 to 5 user needs to be run on same pc please
excellent..
How to run java prorame using java
I did not see the redirectInput, redirectErr, redirectErr methods in java 1.5 docs. May be u need to check this again