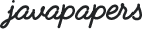
Java JVM provides you a hook to register a thread with the shutdown initiation sequence. Once a thread is registered, on every shutdown that thread is run.
JVM’s shutdown sequence has two phases.
After the above two phases are complete, java virtual machine halts.
Its not a complicated exercise, but a less known feature of java. You might require this to release critical resources in the event of unexpected JVM shutdown. You use finally in a try block to release resources and that is completely different.
Just a single line of code will register with the hook of JVM shutdown. You use, Runtime class to register the thread.
System.getRuntime().addShutdownHook();
public class JVMShutdownHookTest { public static void main(String[] args) { JVMShutdownHook jvmShutdownHook = new JVMShutdownHook(); Runtime.getRuntime().addShutdownHook(jvmShutdownHook); System.out.println("JVM Shutdown Hook Registered."); System.out.println("Pre exit."); System.exit(0); System.out.println("Post exit."); } private static class JVMShutdownHook extends Thread { public void run() { System.out.println("JVM Shutdown Hook: Thread initiated."); } } } Output: JVM Shutdown Hook Registered. Pre exit. JVM Shutdown Hook: Thread initiated.
In the above program, I manualy call the System.exit() and make the JVM to shutdown. Once the System.exit is invoked the phase I of shutdown sequence is initiated and that starts then registered thread ‘jvmShutdownHook’ and then halts the JVM.
Runtime.runFinalizersOnExit() method is almost similar to addShutdownHook() but its depreciated in 1.2
In case if you are wondering on how to detect the web context shutdown in application server scenarios, you can use ServletContextListner
There is no guarantee that always the shutdown hooks will run. There might be a devastating crash of the whole system and JVM might crash due to it. In similar circumstances there is no guarantee that shut down hooks will invoke the thread. Similarly if native OS kills the JVM process then this shutdown hook sequence will not be initiated. Therefore in all cases when the JVM is shutdown abnormally the hook will not be initiated.
Note:
Comments are closed for "JVM Shutdown Hook".
what is difference between System.exit(0);
and System.exit(1);
Is calling Runtime.getRuntime().halt(status), a safe behavior? This would kill other registered threads running in parallel. Correct me if am wrong.
Really Interesting. Got some new Knowledge about something new in Java.
Thanks for the nice and interesting topic.
Can you provide some real time examples where it can be helpful?
I didn’t understand the meaning of “hook” here.and without understanding the proper mean you use this word,this post is meaningless.please don’t mind and reply.
Sir i could not understand about JVM Shutdown. Is it means that Jvm will not work when we apply this code…….??????Please reply me……..
Thanks! Easy to read and informative. I like the stoplight graphics, too.
Rick
@Pradeep
Simply look at the javadocs and you can easily see answer to your question…Anyhow here is a snippet..
” By convention, a nonzero status code indicates abnormal termination.”
So in short System.exit(0) and System.exit(1) will both shutdown the JVM. Non-zero code by convention is used if there is an abnormal shutdown. In the context of this particular post we will use 0 since the shutdown is a well thought shutdown.
Mr. Utkal,
whoever you are, hook is “A piece of metal or other material, curved or bent back at an angle, for catching hold of or hanging things on.”
don’t wate your time on Java website when you want to be an english professor. its not for you.
Good one,,,,
can you post info about Java Class Loaders and Custom class loaders in Java .
nice and useful.
Hi Joe,
You should add captcha for posting comments. This portion is easy to automate and break your website.
Really cool site. Keep it up.
Can shutdown hook be added for scheduled shutdown’s aswell?
@Java Learner
Definitely a shutdown hook can be added for scheduled shutdown as the process of attaching a shutdown hook (which is a thread here) has nothing to do with whether it is a scheduled/unscheduled shutdown. In fact, as Joe has rightly pointed out, in case of unscheduled shutdown, the JVM may not guarantee to invoke the shutdown hook. So a shutdown hook is more useful for a scheduled shutdown of JVM.
Please explain coupling and cohesion
Nice one…Sir,
Plz provide some lession on Comparator and Comparable interface.And with some real time example when to use.
helped me in understanding shudown hooks thanks
Hi.. I have been readiing your post on design patterns and other “Eye-Catching” topics. You have really written very well organized, very useful topics with very simple layman language.. I really appreciate this. Keep writting.
Thanks
Kunal
realy nice topic ,was unknown to this… thanks
sir What is the difference between import in java and include in ‘c’
First time learned about security manager and Shutdown in jvm ….
While learning in more granular layer in
Shutdown class I found a new ThreadDeath class . What’s importance of this class and why they made serialVersionUID hardcoded , where exactly its in use?
interesting articles ….
Excellent work
This blog is very useful
Thank you sooo much…
hello, i want the write shutdown time in a .txt file in real time.
below code doesn’t work ,,,
=======================
public void run()
{
long starttime = 0,shutdown=0;
SimpleDateFormat ft = new SimpleDateFormat(“EEE, yyyy-MM-dd HH:mm:ss a”);
String shut_down_time = ft.format(new Date());
if(flag==true)
{
try
{
Date d=ft.parse(startup_time);
Date dt=ft.parse(shut_down_time);
starttime = d.getTime();
shutdown = dt.getTime();
}
catch (ParseException ex)
{
System.err.println(“abnormal : “+ex.getMessage());
}
Date dNow = new Date();
// log(“Flag true total Active time=”+m.millisecondsToString(shutdown – starttime));
log(“Total idle time = 0 “);
log(“============================================================================”);
log(“Without Idle”);
log(“\n”+”Online Grand Total : “+(shutdown – starttime)+” “+”\n”+”Idle Grand Total : “+total_idle_time+” “);
log(“Total Active Time = “+m.millisecondsToString(shutdown – starttime)+” “+”\nTotal Inactive Time = “+m.millisecondsToString(total_idle_time)+” “+”\n”+ft.format(dNow) + ” =Shutdown time “);
log(“=============================================================================”);
// log(“\n”+”Online Grand Total : “+(shutdown – starttime)+” “+”\n”+”Idle Grand Total : “+total_idle_time+” “);
log(“\nEND”);
}
this code snippet with explanation was so helpful, like this forum.
Thanks.:)
this is very good one…so i think that i need to implement this to my program to understand better.
Thanks:
sir how to save all the data in java application(desktop) if power is off or system is restarted automatically . please reply as soon as possible its urgent.
Thanks in advance
sir how to save all the data in java application(desktop) if power is off or system is restarted automatically . please reply as soon as possible its urgent.
Thanks
Hi Joe,
If we kill the java process using kill command in linux environment would these hooks be in effect.
No, the hooks may not work. It will be an abrupt shut down.
Hi Joe,
I suggest adding some real life examples would greatly benefit readers. For example, taking a thread dump using shutdown hooks is only sane way to track down what caused the JVM crash
There is no difference at all… Both are exactly same. if you check the code of this method it has nothing to do with the argument you pass.
nicely written and useful, thanks
Great piece of information. Thanks for putting it out so well.
Thanks for sharing your knowledge. Very simple and easy to understand.