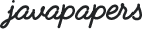
Debugging a remotely running Java application using Eclipse IDE is an important skill in our Java debug arsenal. Eclipse IDE is what I use mostly and so I took it for example. Configuring the same for debug with other IDEs like NetBeans, IntelliJ should be similar.
We will need this remote debug mechanism when the application is running separately outside of IDE. Mostly when the application might be in different system in the network. We will have the source or project configured in IDE in our system. Scenarios include, issue coming only in some X system and application working good in other systems. In that case, we want to remotely debug the application running in that X system.
This is a how-to tutorial and if you know how to remote debug a Java application using Eclipse, please terminate reading this, you may not find anything interesting and finally you will blame me, saying you didn’t expect a so basic article from me. If you are looking for Eclipse debugging tips in general, you can refer to this earlier written super hit article.
java -Xdebug -runjdwp:transport=dt_socket, server=y, suspend=n, address=<debug-port> <ClassName>
package com.javapapers.java; import java.awt.Color; import java.awt.event.ActionEvent; import java.awt.event.ActionListener; import javax.swing.JButton; import javax.swing.JFrame; import javax.swing.JPanel; public class DebugRemote { public static void main(String args[]) throws InterruptedException { final JFrame frame = new JFrame("Remote Debug Demo"); JPanel panel = new JPanel(); final JButton btn = new JButton("On"); btn.setBackground(new Color(50, 200, 100)); frame.getContentPane().add(panel); panel.add(btn); frame.setVisible(true); frame.pack(); btn.addActionListener(new ActionListener() { public void actionPerformed(ActionEvent arg0) { if ("On".equals(btn.getText())) { btn.setText("Off"); btn.setBackground(new Color(200, 50, 100)); } else { btn.setText("On"); btn.setBackground(new Color(50, 200, 100)); } } }); } }
I am running the above program in Java remote debug mode.
Example remote debug application start:
java -Xdebug -runjdwp:transport=dt_socket, server=y, suspend=n, address=6666 com.javapapers.java.DebugRemote
Now, I have kept the break point inside the action listener method and so on click of the button, Eclipse wakes up on debug mode and I get control for remote debugging.
Comments are closed for "Java Remote Debug with Eclipse".
I know how to remote debug in Java. I read your warning not to read this, I ignored and proceeded.
This topic is all about a single line command and nothing great in it.
But still above all, the way you have built an article around it is master class. Going through it is like reading an entertainment magazine. Technology made simple and lively!
Thanks and keep writing forever.
Is the remote debugging supplied in-build in tomcat server? I have to debug my web-application on remote computer. Please let me know.
Very informative and nice way of explaining things.
Waiting for more such articles.
Thanks Joe.
Hi Joe,
Could you please explain how to do remote debug for web application which is running in web application server.
Thank you Joe
@Venkat Bandi I too have the same question.
Hi Joe, Can you please post the steps for the same .
All the Humour Origin is the Keithy Sierra and Bert Bates
No, you need to pass debug parameters while launching tomcat server in catalina.sh
Nice Article Joe
in which file this entry need to go ” java -Xdebug -runjdwp:transport=dt_socket, server=y, suspend=n, address= “