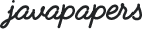
In this Android tutorial we’ll learn to make a calculator APP. Let us plan for a simple and basic functions calculator as it is for learning purposes only. This will help you understand the layouts, buttons and action handling.
We’ll start with making our layout containing numeric Buttons
, operator Button
, an equal Button
, a TextView
and a delete Button
. Give them id
according to their name so that when we’ll write java code, it will be super helpful to understand which buttons we are dealing with.
This file contains the main layout of the calculator APP.
<?xml version="1.0" encoding="utf-8"?> <LinearLayout xmlns:android="http://schemas.android.com/apk/res/android" xmlns:app="http://schemas.android.com/apk/res-auto" xmlns:tools="http://schemas.android.com/tools" android:layout_width="match_parent" android:layout_height="match_parent" android:background="#ecf0f1" android:orientation="vertical" tools:context="com.javapapers.calculator.MainActivity"> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_weight="0.3" android:orientation="horizontal"> <TextView android:id="@+id/display" android:layout_width="match_parent" android:layout_height="match_parent" android:background="#ecf0f1" android:textSize="30sp" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_weight="0.2" android:orientation="horizontal"> <Button android:id="@+id/buttonDel" android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_margin="1dp" android:layout_weight="0.25" android:background="@drawable/button" android:text="Del" android:textColor="#ecf0f1" android:textSize="20sp" /> <Button android:id="@+id/buttoneql" android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_margin="1dp" android:layout_weight="0.25" android:background="@drawable/button" android:text="=" android:textColor="#ecf0f1" android:textSize="30sp" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_weight="0.2" android:orientation="horizontal"> <Button android:id="@+id/button1" android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_margin="1dp" android:layout_weight="0.25" android:background="@drawable/selector" android:text="1" android:textColor="#ecf0f1" android:textSize="20sp" /> <Button android:id="@+id/button2" android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_margin="1dp" android:layout_weight="0.25" android:background="@drawable/selector" android:text="2" android:textColor="#ecf0f1" android:textSize="20sp" /> <Button android:id="@+id/button3" android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_margin="1dp" android:layout_weight="0.25" android:background="@drawable/selector" android:text="3" android:textColor="#ecf0f1" android:textSize="20sp" /> <Button android:id="@+id/buttondiv" android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_margin="1dp" android:layout_weight="0.25" android:background="@drawable/operator_selector" android:text="/" android:textColor="#ecf0f1" android:textSize="30sp" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_weight="0.2" android:orientation="horizontal"> <Button android:id="@+id/button4" android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_margin="1dp" android:layout_weight="0.25" android:background="@drawable/selector" android:text="4" android:textColor="#ecf0f1" android:textSize="20sp" /> <Button android:id="@+id/button5" android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_margin="1dp" android:layout_weight="0.25" android:background="@drawable/selector" android:text="5" android:textColor="#ecf0f1" android:textSize="20sp" /> <Button android:id="@+id/button6" android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_margin="1dp" android:layout_weight="0.25" android:background="@drawable/selector" android:text="6" android:textColor="#ecf0f1" android:textSize="20sp" /> <Button android:id="@+id/buttonsub" android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_margin="1dp" android:layout_weight="0.25" android:background="@drawable/operator_selector" android:text="-" android:textColor="#ecf0f1" android:textSize="30sp" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_weight="0.2" android:orientation="horizontal"> <Button android:id="@+id/button7" android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_margin="1dp" android:layout_weight="0.25" android:background="@drawable/selector" android:text="7" android:textColor="#ecf0f1" android:textSize="20sp" /> <Button android:id="@+id/button8" android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_margin="1dp" android:layout_weight="0.25" android:background="@drawable/selector" android:text="8" android:textColor="#ecf0f1" android:textSize="20sp" /> <Button android:id="@+id/button9" android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_margin="1dp" android:layout_weight="0.25" android:background="@drawable/selector" android:text="9" android:textColor="#ecf0f1" android:textSize="20sp" /> <Button android:id="@+id/buttonmul" android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_margin="1dp" android:layout_weight="0.25" android:background="@drawable/operator_selector" android:text="x" android:textColor="#ecf0f1" android:textSize="30sp" /> </LinearLayout> <LinearLayout android:layout_width="match_parent" android:layout_height="wrap_content" android:layout_weight="0.2" android:orientation="horizontal"> <Button android:id="@+id/buttonDot" android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_margin="1dp" android:layout_weight="0.25" android:background="@drawable/selector" android:text="." android:textColor="#ecf0f1" android:textSize="20sp" /> <Button android:id="@+id/button0" android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_margin="1dp" android:layout_weight="0.25" android:background="@drawable/selector" android:text="0" android:textColor="#ecf0f1" android:textSize="20sp" /> <Button android:id="@+id/Remainder" android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_margin="1dp" android:layout_weight="0.25" android:background="@drawable/operator_selector" android:text="%" android:textColor="#ecf0f1" android:textSize="30sp" /> <Button android:id="@+id/buttonadd" android:layout_width="wrap_content" android:layout_height="match_parent" android:layout_margin="1dp" android:layout_weight="0.25" android:background="@drawable/operator_selector" android:onClick="onClick" android:text="+" android:textColor="#ecf0f1" android:textSize="30sp" /> </LinearLayout> </LinearLayout>
button.xml
This is the button shape used for the calculator buttons.
<?xml version="1.0" encoding="utf-8"?> <shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="rectangle" android:useLevel="false"> <solid android:color="#3498db" /> <corners android:radius="30px"/> <padding android:left="5dp" android:top="5dp" android:right="3dp" android:bottom="3dp"/> </shape>
selector.xml
<?xml version="1.0" encoding="utf-8"?> <selector xmlns:android="http://schemas.android.com/apk/res/android"> <item android:state_pressed="false" android:state_focused="false"> <shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="rectangle" android:useLevel="false"> <corners android:radius="30px"/> <solid android:color="@color/btn_clr"/> <padding android:left="5dp" android:top="5dp" android:right="3dp" android:bottom="3dp"/> </shape> </item> <item android:state_pressed="false" android:state_focused="true"> <shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="rectangle" android:useLevel="false"> <corners android:radius="30px"/> <solid android:color="@color/selected"/> <padding android:left="5dp" android:top="5dp" android:right="3dp" android:bottom="3dp"/> </shape> </item> <item android:state_pressed="true" android:state_focused="false"> <shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="rectangle" android:useLevel="false"> <corners android:radius="30px"/> <solid android:color="@color/selected"/> <padding android:left="5dp" android:top="5dp" android:right="3dp" android:bottom="3dp"/> </shape> </item> </selector>
operator_selector.xml
<?xml version="1.0" encoding="utf-8"?> <selector xmlns:android="http://schemas.android.com/apk/res/android"> <item android:state_pressed="false" android:state_focused="false"> <shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="rectangle" android:useLevel="false"> <corners android:radius="30px"/> <solid android:color="@color/green"/> <padding android:left="5dp" android:top="5dp" android:right="3dp" android:bottom="3dp"/> </shape> </item> <item android:state_pressed="false" android:state_focused="true"> <shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="rectangle" android:useLevel="false"> <corners android:radius="30px"/> <solid android:color="@color/operator_btn_clr"/> <padding android:left="5dp" android:top="5dp" android:right="3dp" android:bottom="3dp"/> </shape> </item> <item android:state_pressed="true" android:state_focused="false"> <shape xmlns:android="http://schemas.android.com/apk/res/android" android:shape="rectangle" android:useLevel="false"> <corners android:radius="30px"/> <solid android:color="@color/operator_btn_clr"/> <padding android:left="5dp" android:top="5dp" android:right="3dp" android:bottom="3dp"/> </shape> </item> </selector>
These files are used to design the app button and you should put these in drawable folder.
Now let’s see about the calculator’s working logic. We’ll start by declaring all the Buttons
, output screen(i.e TextView
), two user inputs and all the operator which is Boolean
(you’ll see why). After declaration, we will make the object of all buttons
(i.e all numbers, operators) and our output screen (i.e where we’ll show the result which is a TextView
). We will set the setOnClickListener
on all buttons
. With this calculator’s buttons are take into control.
After that, we want to show the number which user has pressed and what digit he has entered. Inside the onClick
method we are using this code edt1.setText(edt1.getText() + "number we want to add on the trail of existing number");
. What it does is, whenever user will press the calculator’s button
, it will take the text which is already present on the screen (i.e TextView) and will add the number to the trail which is pressed by the user.
Calculator onClick
For our operator’s onClick
method, we are checking that if user has entered something in the calculator’s input then it will be assigning
the existing number which user has input to our input variable one(input1)
and then defining which operation he has entered so we’ll change our operation’s Boolean
value to true (like Division = true;
that’s means if Division is true then the user has pressed the Division button so according to this we can do the operation) and then we will clear the calculator screen by using setText
method so that the user can enter the next number.
package com.javapapers.calculator; import android.support.v7.app.AppCompatActivity; import android.os.Bundle; import android.view.View; import android.widget.Button; import android.widget.TextView; public class MainActivity extends AppCompatActivity { double input1 = 0, input2 = 0; TextView edt1; boolean Addition, Subtract, Multiplication, Division, mRemainder, decimal; Button button0, button1, button2, button3, button4, button5, button6, button7, button8, button9, buttonAdd, buttonSub, buttonMul, buttonDivision, buttonEqual, buttonDel, buttonDot, Remainder; @Override protected void onCreate(Bundle savedInstanceState) { super.onCreate(savedInstanceState); setContentView(R.layout.activity_main); button0 = (Button) findViewById(R.id.button0); button1 = (Button) findViewById(R.id.button1); button2 = (Button) findViewById(R.id.button2); button3 = (Button) findViewById(R.id.button3); button4 = (Button) findViewById(R.id.button4); button5 = (Button) findViewById(R.id.button5); button6 = (Button) findViewById(R.id.button6); button7 = (Button) findViewById(R.id.button7); button8 = (Button) findViewById(R.id.button8); button9 = (Button) findViewById(R.id.button9); buttonDot = (Button) findViewById(R.id.buttonDot); buttonAdd = (Button) findViewById(R.id.buttonadd); buttonSub = (Button) findViewById(R.id.buttonsub); buttonMul = (Button) findViewById(R.id.buttonmul); buttonDivision = (Button) findViewById(R.id.buttondiv); Remainder = (Button) findViewById(R.id.Remainder); buttonDel = (Button) findViewById(R.id.buttonDel); buttonEqual = (Button) findViewById(R.id.buttoneql); edt1 = (TextView) findViewById(R.id.display); button1.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { edt1.setText(edt1.getText() + "1"); } }); button2.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { edt1.setText(edt1.getText() + "2"); } }); button3.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { edt1.setText(edt1.getText() + "3"); } }); button4.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { edt1.setText(edt1.getText() + "4"); } }); button5.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { edt1.setText(edt1.getText() + "5"); } }); button6.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { edt1.setText(edt1.getText() + "6"); } }); button7.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { edt1.setText(edt1.getText() + "7"); } }); button8.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { edt1.setText(edt1.getText() + "8"); } }); button9.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { edt1.setText(edt1.getText() + "9"); } }); button0.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { edt1.setText(edt1.getText() + "0"); } }); buttonAdd.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { if (edt1.getText().length() != 0) { input1 = Float.parseFloat(edt1.getText() + ""); Addition = true; decimal = false; edt1.setText(null); } } }); buttonSub.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { if (edt1.getText().length() != 0) { input1 = Float.parseFloat(edt1.getText() + ""); Subtract = true; decimal = false; edt1.setText(null); } } }); buttonMul.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { if (edt1.getText().length() != 0) { input1 = Float.parseFloat(edt1.getText() + ""); Multiplication = true; decimal = false; edt1.setText(null); } } }); buttonDivision.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { if (edt1.getText().length() != 0) { input1 = Float.parseFloat(edt1.getText() + ""); Division = true; decimal = false; edt1.setText(null); } } }); Remainder.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { if (edt1.getText().length() != 0) { input1 = Float.parseFloat(edt1.getText() + ""); mRemainder = true; decimal = false; edt1.setText(null); } } }); buttonEqual.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { if (Addition || Subtract || Multiplication || Division || mRemainder) { input2 = Float.parseFloat(edt1.getText() + ""); } if (Addition) { edt1.setText(input1 + input2 + ""); Addition = false; } if (Subtract) { edt1.setText(input1 - input2 + ""); Subtract = false; } if (Multiplication) { edt1.setText(input1 * input2 + ""); Multiplication = false; } if (Division) { edt1.setText(input1 / input2 + ""); Division = false; } if (mRemainder) { edt1.setText(input1 % input2 + ""); mRemainder = false; } } }); buttonDel.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { edt1.setText(""); input1 = 0.0; input2 = 0.0; } }); buttonDot.setOnClickListener(new View.OnClickListener() { @Override public void onClick(View v) { if (decimal) { //do nothing or you can show the error } else { edt1.setText(edt1.getText() + "."); decimal = true; } } }); } }
After entering the second number in the calculator input, user will press the equal button
. Inside the onClick
method of “=” we are doing our operations so first we are assigning
the value to our second input variable (input2) and then we are using if-else statement
to identify (by knowing which operation has a Boolean value as True) which operation is required to be performed or which operation user want to do so in the if
condition we are putting addition/Subtract
as needed (it’s true because we are changing it’s Boolean value to true when user presses the operation button; you can see the onClick
method of any operator).
Inside the body of if
statement we are doing the calculation maths and again changing its Boolean
value to false. Inside the onClick
method of Delete button
we are setting the text of output screen to “” (i.e. nothing) by using setText
and changing the value of all the input to zero. We know that we can’t use two decimal points on single number so if the user has pressed decimal once, he cannot use it again in the calculator’s input until he has pressed any operator button (i.e when user will click the calculator’s operator button, after that digit entered will definitely be the second one and user can put the decimal in second digit; that’s why we are changing its Boolean
value to false in the operator onClick
method).
Hope you liked my simple Android Calculator.
Comments are closed for "How to Build Android Calculator APP".
thanks Joe this helped me better understand layouts which have been one of my weaker android skills